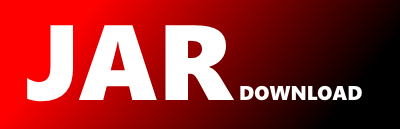
commonMain.com.ditchoom.mqtt3.persistence.SubscriptionQueries.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mqtt-4-models Show documentation
Show all versions of mqtt-4-models Show documentation
Defines the MQTT 3 and 4 control packets
package com.ditchoom.mqtt3.persistence
import app.cash.sqldelight.Query
import app.cash.sqldelight.TransacterImpl
import app.cash.sqldelight.db.QueryResult
import app.cash.sqldelight.db.SqlCursor
import app.cash.sqldelight.db.SqlDriver
import kotlin.Any
import kotlin.Long
import kotlin.String
import kotlin.Unit
public class SubscriptionQueries(
driver: SqlDriver,
) : TransacterImpl(driver) {
public fun allSubscriptions(brokerId: Long, mapper: (
broker_id: Long,
subscribe_packet_id: Long,
unsubscribe_packet_id: Long,
topic_filter: String,
qos: Long,
) -> T): Query = AllSubscriptionsQuery(brokerId) { cursor ->
mapper(
cursor.getLong(0)!!,
cursor.getLong(1)!!,
cursor.getLong(2)!!,
cursor.getString(3)!!,
cursor.getLong(4)!!
)
}
public fun allSubscriptions(brokerId: Long): Query = allSubscriptions(brokerId) {
broker_id, subscribe_packet_id, unsubscribe_packet_id, topic_filter, qos ->
Subscription(
broker_id,
subscribe_packet_id,
unsubscribe_packet_id,
topic_filter,
qos
)
}
public fun allSubscriptionsNotPendingUnsub(brokerId: Long, mapper: (
broker_id: Long,
subscribe_packet_id: Long,
unsubscribe_packet_id: Long,
topic_filter: String,
qos: Long,
) -> T): Query = AllSubscriptionsNotPendingUnsubQuery(brokerId) { cursor ->
mapper(
cursor.getLong(0)!!,
cursor.getLong(1)!!,
cursor.getLong(2)!!,
cursor.getString(3)!!,
cursor.getLong(4)!!
)
}
public fun allSubscriptionsNotPendingUnsub(brokerId: Long): Query =
allSubscriptionsNotPendingUnsub(brokerId) { broker_id, subscribe_packet_id,
unsubscribe_packet_id, topic_filter, qos ->
Subscription(
broker_id,
subscribe_packet_id,
unsubscribe_packet_id,
topic_filter,
qos
)
}
public fun queuedSubscriptions(
brokerId: Long,
subPacketId: Long,
mapper: (
broker_id: Long,
subscribe_packet_id: Long,
unsubscribe_packet_id: Long,
topic_filter: String,
qos: Long,
) -> T,
): Query = QueuedSubscriptionsQuery(brokerId, subPacketId) { cursor ->
mapper(
cursor.getLong(0)!!,
cursor.getLong(1)!!,
cursor.getLong(2)!!,
cursor.getString(3)!!,
cursor.getLong(4)!!
)
}
public fun queuedSubscriptions(brokerId: Long, subPacketId: Long): Query =
queuedSubscriptions(brokerId, subPacketId) { broker_id, subscribe_packet_id,
unsubscribe_packet_id, topic_filter, qos ->
Subscription(
broker_id,
subscribe_packet_id,
unsubscribe_packet_id,
topic_filter,
qos
)
}
public fun queuedUnsubscriptions(
brokerId: Long,
unsubscribePacketId: Long,
mapper: (
broker_id: Long,
subscribe_packet_id: Long,
unsubscribe_packet_id: Long,
topic_filter: String,
qos: Long,
) -> T,
): Query = QueuedUnsubscriptionsQuery(brokerId, unsubscribePacketId) { cursor ->
mapper(
cursor.getLong(0)!!,
cursor.getLong(1)!!,
cursor.getLong(2)!!,
cursor.getString(3)!!,
cursor.getLong(4)!!
)
}
public fun queuedUnsubscriptions(brokerId: Long, unsubscribePacketId: Long): Query =
queuedUnsubscriptions(brokerId, unsubscribePacketId) { broker_id, subscribe_packet_id,
unsubscribe_packet_id, topic_filter, qos ->
Subscription(
broker_id,
subscribe_packet_id,
unsubscribe_packet_id,
topic_filter,
qos
)
}
public fun queuedMessageCount(brokerId: Long): Query = QueuedMessageCountQuery(brokerId) {
cursor ->
cursor.getLong(0)!!
}
public fun insertSubscription(
broker_id: Long,
subscribe_packet_id: Long,
topic_filter: String,
qos: Long,
): Unit {
driver.execute(-1649619357, """
|INSERT INTO Subscription (broker_id, subscribe_packet_id,unsubscribe_packet_id, topic_filter, qos)
|VALUES (?, ?, 0,?, ?)
""".trimMargin(), 4) {
bindLong(0, broker_id)
bindLong(1, subscribe_packet_id)
bindString(2, topic_filter)
bindLong(3, qos)
}
notifyQueries(-1649619357) { emit ->
emit("Subscription")
}
}
public fun addUnsubscriptionPacketId(
unsubPacketId: Long,
brokerId: Long,
topicFilter: String,
): Unit {
driver.execute(-1291357811,
"""UPDATE Subscription SET unsubscribe_packet_id = ? WHERE broker_id = ? AND topic_filter = ?""",
3) {
bindLong(0, unsubPacketId)
bindLong(1, brokerId)
bindString(2, topicFilter)
}
notifyQueries(-1291357811) { emit ->
emit("Subscription")
}
}
public fun deleteSubscription(brokerId: Long, unsubPacketId: Long): Unit {
driver.execute(-1880377771,
"""DELETE FROM Subscription WHERE broker_id = ? AND unsubscribe_packet_id = ?""", 2) {
bindLong(0, brokerId)
bindLong(1, unsubPacketId)
}
notifyQueries(-1880377771) { emit ->
emit("Subscription")
}
}
public fun deleteAll(brokerId: Long): Unit {
driver.execute(-406069911, """DELETE FROM Subscription WHERE broker_id = ?""", 1) {
bindLong(0, brokerId)
}
notifyQueries(-406069911) { emit ->
emit("Subscription")
}
}
private inner class AllSubscriptionsQuery(
public val brokerId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("Subscription"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("Subscription"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(-2007191038, """SELECT * FROM Subscription WHERE broker_id = ?""",
mapper, 1) {
bindLong(0, brokerId)
}
public override fun toString(): String = "Subscription.sq:allSubscriptions"
}
private inner class AllSubscriptionsNotPendingUnsubQuery(
public val brokerId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("Subscription"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("Subscription"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(-639220287,
"""SELECT * FROM Subscription WHERE broker_id = ? AND unsubscribe_packet_id = 0""", mapper,
1) {
bindLong(0, brokerId)
}
public override fun toString(): String = "Subscription.sq:allSubscriptionsNotPendingUnsub"
}
private inner class QueuedSubscriptionsQuery(
public val brokerId: Long,
public val subPacketId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("Subscription"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("Subscription"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(-418384394,
"""SELECT * FROM Subscription WHERE broker_id = ? AND subscribe_packet_id = ? AND unsubscribe_packet_id = 0""",
mapper, 2) {
bindLong(0, brokerId)
bindLong(1, subPacketId)
}
public override fun toString(): String = "Subscription.sq:queuedSubscriptions"
}
private inner class QueuedUnsubscriptionsQuery(
public val brokerId: Long,
public val unsubscribePacketId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("Subscription"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("Subscription"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(1267034109,
"""SELECT * FROM Subscription WHERE broker_id = ? AND unsubscribe_packet_id = ?""", mapper,
2) {
bindLong(0, brokerId)
bindLong(1, unsubscribePacketId)
}
public override fun toString(): String = "Subscription.sq:queuedUnsubscriptions"
}
private inner class QueuedMessageCountQuery(
public val brokerId: Long,
mapper: (SqlCursor) -> T,
) : Query(mapper) {
public override fun addListener(listener: Query.Listener): Unit {
driver.addListener(listener, arrayOf("Subscription"))
}
public override fun removeListener(listener: Query.Listener): Unit {
driver.removeListener(listener, arrayOf("Subscription"))
}
public override fun execute(mapper: (SqlCursor) -> R): QueryResult =
driver.executeQuery(2074001992,
"""SELECT COUNT(broker_id) FROM Subscription WHERE broker_id = ?""", mapper, 1) {
bindLong(0, brokerId)
}
public override fun toString(): String = "Subscription.sq:queuedMessageCount"
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy