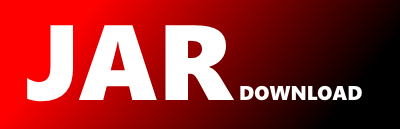
com.github.gwtbootstrap.client.ui.TabPanel Maven / Gradle / Ivy
/*
* Copyright 2012 GWT-Bootstrap
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.github.gwtbootstrap.client.ui;
import java.util.ArrayList;
import java.util.List;
import com.github.gwtbootstrap.client.ui.base.DivWidget;
import com.github.gwtbootstrap.client.ui.resources.Bootstrap;
import com.google.gwt.core.client.GWT;
import com.google.gwt.core.client.Scheduler;
import com.google.gwt.core.client.Scheduler.ScheduledCommand;
import com.google.gwt.dom.client.Element;
import com.google.gwt.dom.client.NativeEvent;
import com.google.gwt.event.dom.client.DomEvent;
import com.google.gwt.event.shared.EventHandler;
import com.google.gwt.event.shared.HandlerRegistration;
import com.google.gwt.user.client.Event;
import com.google.gwt.user.client.ui.IsWidget;
import com.google.gwt.user.client.ui.Widget;
//@formatter:off
/**
* The container for a tabbable nav.
*
* @since 2.0.4.0
* @author Dominik Mayer
* @author ohashi keisuke
*/
//@formatter:on
public class TabPanel extends DivWidget {
public static class ShowEvent extends DomEvent {
private static final Type TYPE = new Type(
"show", new ShowEvent());
private TabLink target;
private TabLink relatedTarget;
protected ShowEvent() {
}
public ShowEvent(NativeEvent event) {
setNativeEvent(event);
if(Element.is(event.getRelatedEventTarget())) {
setRelativeElement(Element.as(event.getRelatedEventTarget()));
}
}
public interface Handler extends EventHandler {
void onShow(ShowEvent showEvent);
}
@Override
protected void dispatch(Handler handler) {
handler.onShow(this);
}
@Override
public com.google.gwt.event.dom.client.DomEvent.Type getAssociatedType() {
return TYPE;
}
/**
* Get target
*
* @return target
*/
public TabLink getTarget() {
return target;
}
/**
* Set target
*
* @param target
* target
*/
public void setTarget(TabLink target) {
this.target = target;
}
/**
* Get relatedTarget。
*
* @return relatedTarget
*/
public TabLink getRelatedTarget() {
return relatedTarget;
}
/**
* Set relatedTarget
*
* @param relatedTarget
* relatedTarget
*/
public void setRelatedTarget(TabLink relatedTarget) {
this.relatedTarget = relatedTarget;
}
}
public static class ShownEvent extends DomEvent {
private static final Type TYPE = new Type(
"shown", new ShownEvent());
private TabLink target;
private TabLink relatedTarget;
protected ShownEvent() {
}
public ShownEvent(NativeEvent event) {
setNativeEvent(event);
if(Element.is(event.getRelatedEventTarget())) {
setRelativeElement(Element.as(event.getRelatedEventTarget()));
}
}
public interface Handler extends EventHandler {
void onShow(ShownEvent shownEvent);
}
@Override
protected void dispatch(Handler handler) {
handler.onShow(this);
}
@Override
public com.google.gwt.event.dom.client.DomEvent.Type getAssociatedType() {
return TYPE;
}
/**
* Get target
*
* @return target
*/
public TabLink getTarget() {
return target;
}
/**
* Set target
*
* @param target
* target
*/
public void setTarget(TabLink target) {
this.target = target;
}
/**
* Get relatedTarget。
*
* @return relatedTarget
*/
public TabLink getRelatedTarget() {
return relatedTarget;
}
/**
* Set relatedTarget
*
* @param relatedTarget
* relatedTarget
*/
public void setRelatedTarget(TabLink relatedTarget) {
this.relatedTarget = relatedTarget;
}
}
private static class TabContent extends DivWidget {
public TabContent() {
setStyleName(Bootstrap.tab_content);
}
}
private NavTabs tabs = new NavTabs();
private List tabLinkList = new ArrayList();
private TabContent tabContent = new TabContent();
/**
* Create an empty {@link Bootstrap.Tabs#ABOVE} style TabPanel.
*/
public TabPanel() {
this(Bootstrap.Tabs.ABOVE);
}
/**
* Create an empty TabPanel.
* @param position tab position.
*/
public TabPanel(Bootstrap.Tabs position) {
setStyle(position);
if(Bootstrap.Tabs.BELOW == position) {
//tabs should be added after content to display it below content in this case
super.add(tabContent);
super.add(tabs);
} else {
super.add(tabs);
super.add(tabContent);
}
setHandlerFunctions(getElement());
}
/**
* Set tab position
* @param position tab position.
*/
public void setTabPosition(String position) {
if (tabs.getParent() != null) {
remove(tabs);
remove(tabContent);
}
if (position.equalsIgnoreCase("below")) {
//tabs should be added after content to display it below content in this case
setStyle(Bootstrap.Tabs.BELOW);
super.add(tabContent);
super.add(tabs);
} else if (position.equalsIgnoreCase("left")) {
setStyle(Bootstrap.Tabs.LEFT);
super.add(tabs);
super.add(tabContent);
} else if (position.equalsIgnoreCase("right")) {
setStyle(Bootstrap.Tabs.RIGHT);
super.add(tabs);
super.add(tabContent);
} else {
setStyle(Bootstrap.Tabs.ABOVE);
super.add(tabs);
super.add(tabContent);
}
}
@Override
public void add(Widget child) {
if (child instanceof TabPane) {
add((TabPane) child);
return;
}
if (child instanceof TabLink) {
add((TabLink) child);
return;
}
if (child instanceof DropdownTab) {
add((DropdownTab) child);
return;
}
if (GWT.isProdMode()) {
throw new IllegalArgumentException(
"TabPanel can add only TabPane or TabLink or Tab or DorpdownTab. you added "
+ child);
}
}
private void add(DropdownTab dropdownTab) {
tabs.add(dropdownTab);
List tabList = dropdownTab.getTabList();
for (Tab tab : tabList) {
tabLinkList.add(tab.asTabLink());
TabPane tabPane = tab.getTabPane();
tabContent.add(tabPane);
}
}
private void add(TabPane child) {
if (child.isCreateTabLink()) {
TabLink tabLink = new TabLink(child);
tabs.add(tabLink);
tabLinkList.add(tabLink);
}
tabContent.add(child);
}
private void add(final TabLink child) {
if (child.isCreateTabPane() && child.getTabPane() == null) {
TabPane pane = new TabPane(child.getText());
child.setTabPane(pane);
tabContent.add(pane);
} else if (child.getTabPane() != null) {
tabContent.add(child.getTabPane());
}
tabs.add(child);
tabLinkList.add(child);
}
@Override
public void clear() {
tabContent.clear();
tabs.clear();
tabLinkList.clear();
}
/**
* Remove tab or tabpane.
*
* If Tablink has TabPane,romve TabPane with TabLink.
{@inheritDoc}
*/
@Override
public boolean remove(int index) {
Widget widget = tabs.getWidget(index);
if (widget instanceof TabLink) {
TabLink link = (TabLink) widget;
if (link.getTabPane() != null) {
link.getTabPane().removeFromParent();
}
tabLinkList.remove(link);
return tabs.remove(index);
} else if (widget instanceof DropdownTab) {
DropdownTab dropdownTab = (DropdownTab) widget;
List*
* {@inheritDoc} */ @Override public boolean remove(Widget w) { if (w instanceof TabLink) { TabLink link = (TabLink) w; tabLinkList.remove(link); if (link.getTabPane() != null) { link.getTabPane().removeFromParent(); } return tabs.remove(w); } else if (w instanceof DropdownTab) { DropdownTab dropdownTab = (DropdownTab) w; List
* if not found, return -1.
*
© 2015 - 2025 Weber Informatics LLC | Privacy Policy