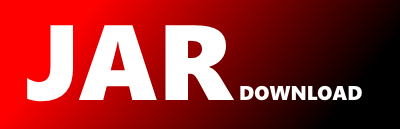
com.dooapp.gaedo.blueprints.queries.tests.AggregatedTargettedVertexTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gaedo-blueprints Show documentation
Show all versions of gaedo-blueprints Show documentation
Implementation of gaedo mechanisms backed by blueprints graph layer
package com.dooapp.gaedo.blueprints.queries.tests;
import java.util.Collection;
import java.util.LinkedList;
import java.util.regex.Pattern;
import com.dooapp.gaedo.blueprints.GraphDatabaseDriver;
import com.dooapp.gaedo.blueprints.strategies.GraphMappingStrategy;
import com.dooapp.gaedo.properties.Property;
public abstract class AggregatedTargettedVertexTest extends TargettedVertexTest implements CompoundVertexTest{
protected Collection tests = new LinkedList();
public AggregatedTargettedVertexTest(GraphMappingStrategy> strategy, GraphDatabaseDriver driver, Iterable p) {
super(strategy, driver, p);
}
public Type add(Type test) {
this.tests.add(test);
return test;
}
public OrVertexTest or() {
return add(new OrVertexTest(strategy, getDriver(), path));
}
public AndVertexTest and() {
return add(new AndVertexTest(strategy, getDriver(), path));
}
public NotVertexTest not() {
return add(new NotVertexTest(strategy, getDriver(), path));
}
/**
* Adds a new {@link EqualsTo} test to {@link #tests} and returns it
* @param path
* @param value
* @see com.dooapp.gaedo.blueprints.queries.tests.CompoundVertexTest#equalsTo(com.dooapp.gaedo.properties.Property, java.lang.Object)
* @category simple
*/
public VertexTest equalsTo(Iterable path, Object value) {
return add(new EqualsTo(strategy, getDriver(), path, value));
}
@Override
public VertexTest instanceOf(Iterable path, Class> type) {
return add(new InstanceOf(strategy, getDriver(), path, type));
}
/**
* Adds a new {@link GreaterThan} test to {@link #tests} and returns it
* @param path
* @param value
* @return
* @category simple
*/
public > VertexTest greaterThan(Iterable path, ComparableType value, boolean strictly) {
return add(new GreaterThan(strategy, getDriver(), path, value, strictly));
}
/**
* Adds a new {@link LowerThan} test to {@link #tests} and returns it
* @param path
* @param value
* @return
* @category simple
*/
@Override
public > VertexTest lowerThan(Iterable path, ComparableType value, boolean strictly) {
return add(new LowerThan(strategy, getDriver(), path, value, strictly));
}
@Override
public VertexTest matches(Iterable path, Pattern pattern) {
return add(new Matches(strategy, getDriver(), path, pattern));
}
@Override
public VertexTest containsString(Iterable path, String contained) {
return add(new ContainsString(strategy, getDriver(), path, contained));
}
@Override
public VertexTest equalsToIgnoreCase(Iterable path, String compared) {
return add(new EqualsToIgnoreCase(strategy, getDriver(), path, compared));
}
@Override
public VertexTest startsWith(Iterable path, String start) {
return add(new StartsWith(strategy, getDriver(), path, start));
}
@Override
public VertexTest endsWith(Iterable path, String end) {
return add(new EndsWith(strategy, getDriver(), path, end));
}
@Override
public VertexTest collectionContains(Iterable path, Object contained) {
return add(new CollectionContains(strategy, getDriver(), path, contained));
}
@Override
public VertexTest mapContainsValue(Iterable path, Object contained) {
return add(new MapContainsValue(strategy, getDriver(), path, contained));
}
@Override
public VertexTest mapContainsKey(Iterable path, Object contained) {
return add(new MapContainsKey(strategy, getDriver(), path, contained));
}
@Override
public VertexTest anything(Iterable path) {
return add(new Anything(strategy, getDriver(), path));
}
@Override
protected StringBuilder toString(int deepness, StringBuilder builder) {
StringBuilder returned = super.toString(deepness, builder).append("\n");
for(VertexTest v : tests) {
if(v instanceof TargettedVertexTest) {
returned = ((TargettedVertexTest) v).toString(deepness+1, returned);
} else {
returned.append("\nwhat ? a non targettted vertex test ? what the hell ?");
}
}
return returned;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy