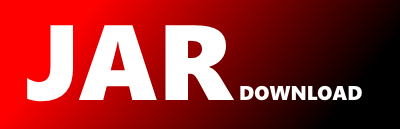
com.dooapp.gaedo.blueprints.strategies.GraphMappingStrategy Maven / Gradle / Ivy
package com.dooapp.gaedo.blueprints.strategies;
import java.util.Collection;
import java.util.Map;
import javax.persistence.CascadeType;
import com.dooapp.gaedo.blueprints.AbstractBluePrintsBackedFinderService;
import com.dooapp.gaedo.blueprints.ObjectCache;
import com.dooapp.gaedo.blueprints.queries.tests.CompoundVertexTest;
import com.dooapp.gaedo.properties.Property;
import com.tinkerpop.blueprints.Edge;
import com.tinkerpop.blueprints.Vertex;
/**
* Interface adapting some specific behaviours of a graph service. This interface should typically allow one to choose bvetween bean based graph mapping and graph based bean mapping (in which case
* one could use PropertyBag and associated classes).
* @author ndx
*
*/
public interface GraphMappingStrategy {
public static final String STRING_TYPE = String.class.getName();
/**
* Helper method providing the required properties for a class. It is an equivalent of {@link #getContainedProperties(Object, Vertex, CascadeType)}
* @param objectClass class for which properties are required
* @return map linking properties to their cascade mode
*/
public Map> getContainedProperties(Class extends Object> objectClass);
/**
* Delegation method allowing one to get the map linking properties to cascade types
* @param object object for which we want the properties
* @param vertex vertex associated to that object
* @param cascadeType type of cascadde we want the properties for
* @return a map associating each property to its cascade type (useful for persistence operation, but will have to be slightly updated for load operations).
*/
public Map> getContainedProperties(DataType object, Vertex vertex, CascadeType cascadeType);
public void assignId(final DataType value, final Object... id);
public void generateValidIdFor(DataType toCreate);
/**
* Get an id string for the given object
* @param object
* @return id of that object, or null if no id can be constructed.
*/
public String getIdString(DataType object);
/**
* Transform given object into a string that could be an object id (provided that object id was generated by {@link #getIdString(Object)})
* @param object
* @return
*/
public String getAsId(Object object);
public Collection getIdProperties();
public boolean isIdGenerationRequired();
/**
* Initialization method allowing strategy to load some infos built during service init (like, as an example, the GraphDatabaseDriver or the service itself).
* @param service
*/
public void reloadWith(AbstractBluePrintsBackedFinderService, DataType, ?> service);
/**
* Add default search to the given vertex test. That default search makes sure results are limited to object of this class (and also ensures request will have effective results).
* @param initial initial search
* @return search with class check configured in
*/
public CompoundVertexTest addDefaultSearchTo(CompoundVertexTest initial);
/**
* Get effective type, as string, of a given vertex.
* @param vertex vertex for which we want type infos
* @return effective type of vertex.
* @throws UnableToGetVertexTypeException if no vertex type info can be found
*/
public String getEffectiveType(Vertex vertex);
/**
* Method invoked when vertex has been loaded in object. Allows strategy to perform any customization.
* @param fromId TODO
* @param from
* @param into
* @param objectsBeingAccessed TODO
*/
public void loaded(String fromId, Vertex from, DataType into, ObjectCache objectsBeingAccessed);
/**
* Get collection of edges going from the given rootVertex and corresponding to the given property
* @param rootVertex
* @param p
* @return
*/
public Iterable getOutEdgesFor(Vertex rootVertex, Property p);
/**
* Determine if objectVertex associated properties should be loaded.
* @param objectVertexId object vertex id
* @param objectVertex object vertex to load
* @param objectsBeingAccessed already loaded vertices ids and associated objects
* @return
*/
public boolean shouldLoadPropertiesOf(String objectVertexId, Vertex objectVertex, ObjectCache objectsBeingAccessed);
/**
* Clone service for a new service to use a derived version of this one
* @return
*/
public GraphMappingStrategy derive();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy