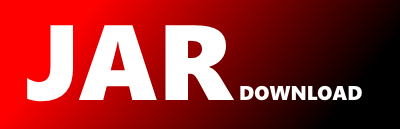
com.dooapp.gaedo.finders.collections.CollectionQueryStatement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gaedo-collections Show documentation
Show all versions of gaedo-collections Show documentation
Contains the collections implementation, and only depend upon gaedo-definition
package com.dooapp.gaedo.finders.collections;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.TreeSet;
import com.dooapp.gaedo.exceptions.range.BadRangeDefinitionException;
import com.dooapp.gaedo.exceptions.range.BadStartIndexException;
import com.dooapp.gaedo.finders.Informer;
import com.dooapp.gaedo.finders.QueryBuilder;
import com.dooapp.gaedo.finders.QueryExpression;
import com.dooapp.gaedo.finders.root.AbstractQueryStatement;
import com.dooapp.gaedo.finders.sort.SortingBackedComparator;
import com.dooapp.gaedo.utils.PropertyChangeEmitter;
/**
* Build a query statement backed by the collection this service relies upon
*
* @author ndx
*
*/
public class CollectionQueryStatement>
extends AbstractQueryStatement {
/**
* Collection used for tests
*/
private Iterable data;
public CollectionQueryStatement(QueryBuilder query,
InformerType informer, Iterable data,
PropertyChangeEmitter emitter) {
super(query, informer, emitter);
this.data = data;
}
/**
* Count the number of elements that matches the QueryExpression
*/
public int count() {
try {
return selectAll().size();
} finally {
setState(State.EXECUTED);
}
}
public Iterable get(int start, int end) {
try {
if (start < 0) {
throw new BadStartIndexException(start);
} else if (end < start) {
throw new BadRangeDefinitionException(start, end);
}
return selectAll().subList(start, end);
} finally {
setState(State.EXECUTED);
}
}
public Iterable getAll() {
try {
Iterable returned = selectAll();
if (getSortingExpression() != null && getSortingExpression().iterator().hasNext()) {
returned = new TreeSet(createComparator());
}
return returned;
} finally {
setState(State.EXECUTED);
}
}
/**
* Creates a comparator from the sorting expression
*
* @return
*/
private Comparator super DataType> createComparator() {
return new SortingBackedComparator(getSortingExpression());
}
/**
* For getting all data, we build a matcher by calling
* {@link #createMatcher()} then use it against all elements of collection
*/
private List selectAll() {
List returned = new ArrayList();
Matcher expression = createMatcher();
for (DataType element : data) {
if (expression.matches(element)) {
returned.add(element);
}
}
return returned;
}
/**
* Creates a matcher by visiting the QueryExpression
*
* @return
*/
private Matcher createMatcher() {
QueryExpression expression = buildQueryExpression();
Matcher matcher = new Matcher();
// When the expression accepts the matcher, the matcher will construct
// its evaluation tree
expression.accept(matcher);
return matcher;
}
public DataType getFirst() {
try {
return selectAll().get(0);
} finally {
setState(State.EXECUTED);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy