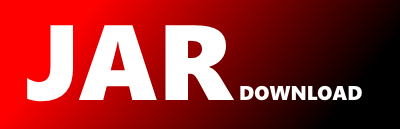
com.dooapp.gaedo.finders.informers.ObjectFieldInformer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gaedo-definition Show documentation
Show all versions of gaedo-definition Show documentation
Hold the services interfaces, informers and expressions
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy