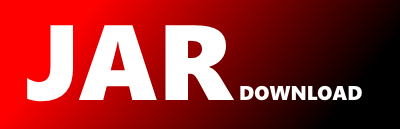
com.dooapp.gaedo.utils.BasicInvocationHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gaedo-definition Show documentation
Show all versions of gaedo-definition Show documentation
Hold the services interfaces, informers and expressions
The newest version!
package com.dooapp.gaedo.utils;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import com.dooapp.gaedo.CrudServiceException;
/**
* Base class for some of our invocation handlers. This class provides some
* useful method for handling comon cases
*
* @author ndx
*
*/
public abstract class BasicInvocationHandler {
/**
* Map of method resolvers.
*/
private final Map resolvers = new HashMap();
/**
* Map of method resolvers usable by subclass. This one is a read-only view
* of {@link #resolvers}.
*/
protected final Map loadedResolvers = Collections.unmodifiableMap(resolvers);
/**
* Target interface implemented here
*/
protected final Class toImplement;
public BasicInvocationHandler(Class toImplement) {
super();
this.toImplement = toImplement;
}
/**
* Create method resolvers for the given class (which *must* denote an
* interface and all its super interfaces. This allows simpler code to be
* written in later invoke handlers
*
* @param toImplement
*/
protected void createAllMethodResolvers(Class> toImplement) {
createResolversForDeclaredMethods(toImplement);
for (Class> parentInterface : toImplement.getInterfaces()) {
createAllMethodResolvers(parentInterface);
}
}
/**
* Create all method resolvers. For that, the declared methods of the class
* to implement are all browsed, and a resolver is created for each.
* Notice it is a good practice to invoke this method during construction
*
* @param toImplement
* target class. Each method of this class will have a resolver
* associated to it
*/
protected void createResolversForDeclaredMethods(Class>... toImplement) {
List exceptions = new LinkedList();
for (Class> currentClassToImplement : toImplement) {
for (Method m : currentClassToImplement.getDeclaredMethods()) {
try {
getResolver(m);
} catch (VirtualMethodCreationException e) {
exceptions.add(e);
}
}
}
if (exceptions.size() > 0) {
// in case of a simple exception, we try to be quite informative one
// xception root cause
if (exceptions.size() == 1) {
throw exceptions.get(0);
}
// when there are multiple ones, we will only give a shortened
// message, however complete
throw new UnableToCreateInvocationHandlerException(toImplement, exceptions);
}
}
/**
* Get resolver and cache it
*
* @param method
* @return
*/
protected VirtualMethodResolver getResolver(Method method) {
if (!resolvers.containsKey(method)) {
resolvers.put(method, createResolver(method));
}
return resolvers.get(method);
}
/**
* Create method resolver for the given method
*
* @param method
* @return
* @throws VirtualMethodCreationException
* TODO
*/
protected abstract VirtualMethodResolver createResolver(Method method) throws VirtualMethodCreationException;
/**
* @param proxy
* proxy object, considered as useless
* @param invokedMethod
* method object corresponding to the invoked code.
* @param invokedArgs
* invocation arguments.
*/
public Object invoke(Object proxy, Method invokedMethod, Object[] invokedArgs) throws Throwable {
MethodResolver resolver = getResolver(invokedMethod);
try {
return resolver.call(invokedArgs);
} catch(InvocationTargetException e) {
if(e.getCause() instanceof CrudServiceException) {
throw ((CrudServiceException)e.getCause());
} else {
throw e;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy