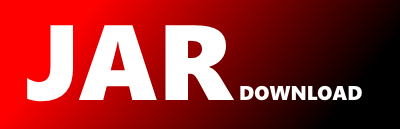
dorkbox.util.bytes.UByte Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Utilities Show documentation
Show all versions of Utilities Show documentation
Utilities for use within Java projects
/*
* Copyright (c) 2011-2017, Data Geekery GmbH (http://www.datageekery.com)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package dorkbox.util.bytes;
import java.io.ObjectStreamException;
import java.math.BigInteger;
/**
* The unsigned byte
type
*
* @author Lukas Eder
* @author Ed Schaller
* @author Jens Nerche
*/
public final class UByte extends UNumber implements Comparable {
/**
* Generated UID
*/
private static final long serialVersionUID = -6821055240959745390L;
/**
* Cached values
*/
private static final UByte[] VALUES = mkValues();
/**
* A constant holding the minimum value an unsigned byte
can
* have, 0.
*/
public static final short MIN_VALUE = 0x00;
/**
* A constant holding the maximum value an unsigned byte
can
* have, 28-1.
*/
public static final short MAX_VALUE = 0xff;
/**
* A constant holding the minimum value an unsigned byte
can
* have as UByte, 0.
*/
public static final UByte MIN = valueOf(0x00);
/**
* A constant holding the maximum value an unsigned byte
can
* have as UByte, 28-1.
*/
public static final UByte MAX = valueOf(0xff);
/**
* The value modelling the content of this unsigned byte
*/
private final short value;
/**
* Generate a cached value for each byte value.
*
* @return Array of cached values for UByte.
*/
private static final UByte[] mkValues() {
UByte[] ret = new UByte[256];
for (int i = Byte.MIN_VALUE; i <= Byte.MAX_VALUE; i++)
ret[i & MAX_VALUE] = new UByte((byte) i);
return ret;
}
/**
* Get an instance of an unsigned byte
*
* @throws NumberFormatException If value
does not contain a
* parsable unsigned byte
.
*/
public static UByte valueOf(String value) throws NumberFormatException {
return valueOfUnchecked(rangeCheck(Short.parseShort(value)));
}
/**
* Get an instance of an unsigned byte
by masking it with
* 0xFF
i.e. (byte) -1
becomes
* (ubyte) 255
*/
public static UByte valueOf(byte value) {
return valueOfUnchecked((short) (value & MAX_VALUE));
}
/**
* Get the value of a short without checking the value.
*/
private static UByte valueOfUnchecked(short value) throws NumberFormatException {
return VALUES[value & MAX_VALUE];
}
/**
* Get an instance of an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
*/
public static UByte valueOf(short value) throws NumberFormatException {
return valueOfUnchecked(rangeCheck(value));
}
/**
* Get an instance of an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
*/
public static UByte valueOf(int value) throws NumberFormatException {
return valueOfUnchecked(rangeCheck(value));
}
/**
* Get an instance of an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
*/
public static UByte valueOf(long value) throws NumberFormatException {
return valueOfUnchecked(rangeCheck(value));
}
/**
* Create an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
*/
private UByte(long value) throws NumberFormatException {
this.value = rangeCheck(value);
}
/**
* Create an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
*/
private UByte(int value) throws NumberFormatException {
this.value = rangeCheck(value);
}
/**
* Create an unsigned byte
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned byte
*/
private UByte(short value) throws NumberFormatException {
this.value = rangeCheck(value);
}
/**
* Create an unsigned byte
by masking it with 0xFF
* i.e. (byte) -1
becomes (ubyte) 255
*/
private UByte(byte value) {
this.value = (short) (value & MAX_VALUE);
}
/**
* Create an unsigned byte
*
* @throws NumberFormatException If value
does not contain a
* parsable unsigned byte
.
*/
private UByte(String value) throws NumberFormatException {
this.value = rangeCheck(Short.parseShort(value));
}
/**
* Throw exception if value out of range (short version)
*
* @param value Value to check
* @return value if it is in range
* @throws NumberFormatException if value is out of range
*/
private static short rangeCheck(short value) throws NumberFormatException {
if (value < MIN_VALUE || value > MAX_VALUE)
throw new NumberFormatException("Value is out of range : " + value);
return value;
}
/**
* Throw exception if value out of range (int version)
*
* @param value Value to check
* @return value if it is in range
* @throws NumberFormatException if value is out of range
*/
private static short rangeCheck(int value) throws NumberFormatException {
if (value < MIN_VALUE || value > MAX_VALUE)
throw new NumberFormatException("Value is out of range : " + value);
return (short) value;
}
/**
* Throw exception if value out of range (long version)
*
* @param value Value to check
* @return value if it is in range
* @throws NumberFormatException if value is out of range
*/
private static short rangeCheck(long value) throws NumberFormatException {
if (value < MIN_VALUE || value > MAX_VALUE)
throw new NumberFormatException("Value is out of range : " + value);
return (short) value;
}
/**
* Replace version read through deserialization with cached version. Note
* that this does not use the {@link #valueOfUnchecked(short)} as we have no
* guarantee that the value from the stream is valid.
*
* @return cached instance of this object's value
* @throws ObjectStreamException
*/
private Object readResolve() throws ObjectStreamException {
return valueOf(value);
}
@Override
public int intValue() {
return value;
}
@Override
public long longValue() {
return value;
}
@Override
public float floatValue() {
return value;
}
@Override
public double doubleValue() {
return value;
}
@Override
public int hashCode() {
return Short.valueOf(value).hashCode();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj instanceof UByte)
return value == ((UByte) obj).value;
return false;
}
@Override
public String toString() {
return Short.valueOf(value).toString();
}
@Override
public String toHexString() {
return Integer.toHexString(this.value);
}
@Override
public int compareTo(UByte o) {
return (value < o.value ? -1 : (value == o.value ? 0 : 1));
}
@Override
public BigInteger toBigInteger() {
return BigInteger.valueOf(value);
}
public UByte add(UByte val) throws NumberFormatException {
return valueOf(value + val.value);
}
public UByte add(int val) throws NumberFormatException {
return valueOf(value + val);
}
public UByte subtract(final UByte val) {
return valueOf(value - val.value);
}
public UByte subtract(final int val) {
return valueOf(value - val);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy