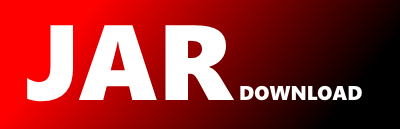
dorkbox.util.bytes.UShort Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Utilities Show documentation
Show all versions of Utilities Show documentation
Utilities for use within Java projects
/*
* Copyright (c) 2011-2017, Data Geekery GmbH (http://www.datageekery.com)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package dorkbox.util.bytes;
import java.math.BigInteger;
/**
* The unsigned short
type
*
* @author Lukas Eder
* @author Jens Nerche
*/
public final class UShort extends UNumber implements Comparable {
/**
* Generated UID
*/
private static final long serialVersionUID = -6821055240959745390L;
/**
* A constant holding the minimum value an unsigned short
can
* have, 0.
*/
public static final int MIN_VALUE = 0x0000;
/**
* A constant holding the maximum value an unsigned short
can
* have, 216-1.
*/
public static final int MAX_VALUE = 0xffff;
/**
* A constant holding the minimum value an unsigned short
can
* have as UShort, 0.
*/
public static final UShort MIN = valueOf(MIN_VALUE);
/**
* A constant holding the maximum value an unsigned short
can
* have as UShort, 216-1.
*/
public static final UShort MAX = valueOf(MAX_VALUE);
/**
* The value modelling the content of this unsigned short
*/
private final int value;
/**
* Create an unsigned short
*
* @throws NumberFormatException If value
does not contain a
* parsable unsigned short
.
*/
public static UShort valueOf(String value) throws NumberFormatException {
return new UShort(value);
}
/**
* Create an unsigned short
by masking it with
* 0xFFFF
i.e. (short) -1
becomes
* (ushort) 65535
*/
public static UShort valueOf(short value) {
return new UShort(value);
}
/**
* Create an unsigned short
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned short
*/
public static UShort valueOf(int value) throws NumberFormatException {
return new UShort(value);
}
/**
* Create an unsigned short
*
* @throws NumberFormatException If value
is not in the range
* of an unsigned short
*/
private UShort(int value) throws NumberFormatException {
this.value = value;
rangeCheck();
}
/**
* Create an unsigned short
by masking it with
* 0xFFFF
i.e. (short) -1
becomes
* (ushort) 65535
*/
private UShort(short value) {
this.value = value & MAX_VALUE;
}
/**
* Create an unsigned short
*
* @throws NumberFormatException If value
does not contain a
* parsable unsigned short
.
*/
private UShort(String value) throws NumberFormatException {
this.value = Integer.parseInt(value);
rangeCheck();
}
private void rangeCheck() throws NumberFormatException {
if (value < MIN_VALUE || value > MAX_VALUE)
throw new NumberFormatException("Value is out of range : " + value);
}
@Override
public int intValue() {
return value;
}
@Override
public long longValue() {
return value;
}
@Override
public float floatValue() {
return value;
}
@Override
public double doubleValue() {
return value;
}
@Override
public BigInteger toBigInteger() {
return BigInteger.valueOf(value);
}
@Override
public int hashCode() {
return Integer.valueOf(value).hashCode();
}
@Override
public boolean equals(Object obj) {
if (obj instanceof UShort)
return value == ((UShort) obj).value;
return false;
}
@Override
public String toString() {
return Integer.valueOf(value).toString();
}
@Override
public String toHexString() {
return Integer.toHexString(this.value);
}
@Override
public int compareTo(UShort o) {
return (value < o.value ? -1 : (value == o.value ? 0 : 1));
}
public UShort add(UShort val) throws NumberFormatException {
return valueOf(value + val.value);
}
public UShort add(int val) throws NumberFormatException {
return valueOf(value + val);
}
public UShort subtract(final UShort val) {
return valueOf(value - val.value);
}
public UShort subtract(final int val) {
return valueOf(value - val);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy