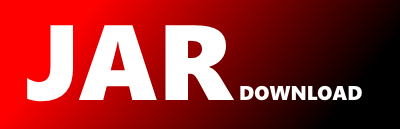
com.dottydingo.hyperion.client.builder.RequestFactory Maven / Gradle / Ivy
package com.dottydingo.hyperion.client.builder;
import com.dottydingo.hyperion.api.ApiObject;
import com.dottydingo.hyperion.api.Endpoint;
import com.dottydingo.hyperion.client.builder.query.QueryExpression;
import com.dottydingo.hyperion.client.exception.ClientException;
import java.io.Serializable;
import java.util.Collections;
import java.util.List;
/**
* Factory for creating request builders for a specific entity
*/
public class RequestFactory,ID extends Serializable>
{
private int version;
private Class type;
private String entityName;
/**
* Create a request factory using the supplied API type.
* @param type The API type, must have an @Endpoint annotation.
*/
public RequestFactory(Class type)
{
Endpoint endpoint = getEndpointAnnotation(type);
this.version = endpoint.version();
this.type = type;
this.entityName = endpoint.value();
}
/**
* Create a request factory using a specified version, API type, and entity name. Does not require that the API type
* have an @Endpoint annotation
* @param version The version
* @param type The type
* @param entityName The entity name
*/
public RequestFactory(int version, Class type, String entityName)
{
this.version = version;
this.type = type;
this.entityName = entityName;
}
/**
* Create a request builder for a create operation for a single item
* @param item The item to create
* @return The request builder
*/
public CreateSingleRequestBuilder create(T item)
{
return new CreateSingleRequestBuilder<>(version,type,entityName,item);
}
/**
* Create a request builder for a create operation for a multiple items
* @param items The items to create
* @return The request builder
*/
public CreateCollectionRequestBuilder create(List items)
{
return new CreateCollectionRequestBuilder(version,type,entityName,items);
}
/**
* Create a request builder for a delete operation using the specified IDs
* @param ids The ids to delete
* @return The request builder
*/
public DeleteRequestBuilder delete(ID... ids)
{
return new DeleteRequestBuilder(version,type,entityName,ids);
}
/**
* Create a request builder for a delete operation using the specified IDs
* @param ids The ids to delete
* @return The request builder
*/
public DeleteRequestBuilder delete(List ids)
{
return new DeleteRequestBuilder(version,type,entityName, (ID[]) ids.toArray(new Serializable[ids.size()]));
}
/**
* Create a request builder for a query operation using the specified query RQL string
* @param query The the query
* @return The request builder
*/
public QueryRequestBuilder query(String query)
{
return new QueryRequestBuilder(version,type,entityName,query);
}
/**
* Create a request builder for a query operation using the specified query expression
* @param query The the query expression
* @return The request builder
*/
public QueryRequestBuilder query(QueryExpression query)
{
return new QueryRequestBuilder(version,type,entityName,query.build());
}
/**
* Create a request builder for a query operation using the specified no query
* @return The request builder
*/
public QueryRequestBuilder query()
{
return new QueryRequestBuilder(version,type,entityName);
}
/**
* Create a request builder for a find operation using the specified IDs
* @param ids The ids to find
* @return The request builder
*/
public GetRequestBuilder find(ID... ids)
{
return new GetRequestBuilder(version,type,entityName,ids);
}
/**
* Create a request builder for an update operation for a single item
* @param item The item to update
* @return The request builder
*/
public UpdateSingleRequestBuilder update(T item)
{
return new UpdateSingleRequestBuilder<>(version,type,entityName,item);
}
/**
* Create a request builder for a create operation for a multiple items
* @param items The item to update
* @return The request builder
*/
public UpdateCollectionRequestBuilder update(List items)
{
return new UpdateCollectionRequestBuilder(version,type,entityName,items);
}
protected Endpoint getEndpointAnnotation(Class extends ApiObject> apiClass)
{
Endpoint endpoint = apiClass.getAnnotation(Endpoint.class);
if(endpoint == null)
throw new ClientException(String.format("Missing @Endpoint annotation in apiClass %s",apiClass));
return endpoint;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy