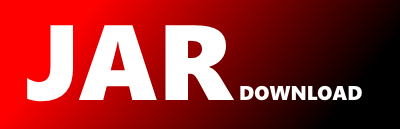
com.terapico.uccaf.explorer.BaseExplorer Maven / Gradle / Ivy
The newest version!
package com.terapico.uccaf.explorer;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.terapico.uccaf.BaseUserContext;
public abstract class BaseExplorer {
protected static final int CODE_CONTINUE = 0;
protected static final int CODE_JUMP = 1;
protected abstract void buildTeam(Map> team);
public abstract String getStartFromName();
public abstract String getExploreName();
protected Map> adventureTeam = null;
public Map> getAdventureTeam() {
if (adventureTeam != null) {
return adventureTeam;
}
synchronized (this.getClass()) {
if (adventureTeam != null) {
return adventureTeam;
}
Map> team = new HashMap<>();
buildTeam(team);
adventureTeam = team;
return adventureTeam;
}
}
public ExplorationResults explore(U ctx, Object tgtObject) throws Exception {
Map> adventureTeam = this.getAdventureTeam();
PathFinder adventurer = adventureTeam.get(getStartFromName());
List tasks = new ArrayList<>();
goThroughPaths(ctx, tgtObject, adventureTeam, adventurer, tasks);
ExplorationResults result = new ExplorationResults();
result.setTodoTasks(tasks);
return result;
}
protected void goThroughPaths(U ctx, Object tgtObject, Map> adventureTeam,
PathFinder adventurer, List tasks) throws Exception {
for (;;) {
ExplorationResults exploreResult = adventurer.explore(ctx, tgtObject);
if (exploreResult.getTodoTasks() != null && !exploreResult.getTodoTasks().isEmpty()) {
tasks.addAll(exploreResult.getTodoTasks());
}
if (exploreResult.isStop()) {
return;
}
String nextAdventurerName = adventurer.getNextAdventurerName();
if (exploreResult.isJump()) {
nextAdventurerName = exploreResult.getNextAdventurerName();
}
if (nextAdventurerName == null || !adventureTeam.containsKey(nextAdventurerName)) {
return;
}
adventurer = adventureTeam.get(nextAdventurerName);
}
}
protected void addTaskNameIntoExploreResultTaskSet(U ctx, String key, String taskName) {
@SuppressWarnings("unchecked")
Set rst = (Set) ctx.getFromContextLocalStorage(key);
if (rst == null) {
rst = new HashSet<>();
ctx.putIntoContextLocalStorage(key, rst);
}
rst.add(taskName);
}
protected void log(U ctx, String adventurer, int resultCode) {
ctx.log(String.format("Run [%s]:%d (%s)", adventurer, resultCode, getCodeName(resultCode)));
}
protected Object getCodeName(int resultCode) {
String name = getCodeNames().get(resultCode);
if (name == null) {
return "no-name";
}
return name;
}
protected abstract Map getCodeNames();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy