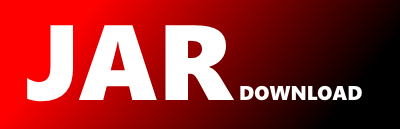
com.terapico.utils.BaseCandidatesUtil Maven / Gradle / Ivy
The newest version!
package com.terapico.utils;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.regex.Pattern;
import com.terapico.caf.baseelement.CandidateQuery;
@SuppressWarnings("unchecked")
public abstract class BaseCandidatesUtil {
protected static class Initor {
protected String modelName;
protected String workingType; // usedIn|referTo
protected String workingModelName;
public Initor(String name) {
this.modelName = name;
}
protected Map get() {
Map rst = QUERY_RELATIONS.get(this.modelName);
if (rst != null) {
return rst;
}
rst = new HashMap<>();
QUERY_RELATIONS.put(modelName, rst);
return rst;
}
protected void addData(String dataLabel, String typeName, String data) {
Map cData = (Map) get().get(dataLabel);
if (cData == null) {
cData = new HashMap<>();
get().put(dataLabel, cData);
}
List existedData = (List) cData.get(typeName);
if (existedData == null) {
existedData = new ArrayList<>();
cData.put(typeName, existedData);
}
existedData.add(data);
}
public Initor usedIn(String typeName) {
this.workingModelName = typeName;
this.workingType = "usedIn";
return this;
}
public Initor withRole(String columnName) {
addData(workingType, workingModelName, columnName);
return this;
}
public Initor referTo(String typeName) {
this.workingModelName = typeName;
this.workingType = "referTo";
return this;
}
public Initor targetType(String value) {
get().put("name", value);
return this;
}
public Initor groupBy(String typeName) {
this.workingModelName = typeName;
this.workingType = "groupBy";
return this;
}
public Initor whichType(String value) {
addData(workingType, workingModelName, value);
return this;
}
public Initor isTree(Object value) {
get().put("isTree", DataTypeUtil.getBoolean(value));
return this;
}
public Initor hasFields(String string) {
get().put("fields", string);
return this;
}
public Initor anchorColumn(String value) {
get().put("anchorColumn", value);
return this;
}
}
protected static Map> QUERY_RELATIONS = new HashMap<>();
protected abstract String normalizeModelName(String typeName) throws Exception;
protected abstract String getDisplayNameColumn(String typeName);
protected static Initor _for(String name) {
return new Initor(name);
}
protected CandidateQuery prepareQueryInput(CandidateQuery orginalQuery) throws Exception {
// 先规整参数名字,都用模型名的格式
CandidateQuery query = getQueryFromInput(orginalQuery);
// check Target related params
checkTargetParams(orginalQuery, query);
// fix params
fixOwnerParam(query);
fixListParam(query);
fixOwnerInListParam(query);
fixGroupByParam(query);
fixOwnerRoleInTarget(query);
fixOwnerRoleInList(query);
fixTargetRoleInList(query);
autoFillOwnerRoleInTarget(query);
autoFillTargetRoleInList(query);
autoFillOwnerRoleInList(query);
autoFillGroupBy(query);
System.out.println("最终的查询参数为:" + DebugUtil.dumpAsJson(query, true));
return query;
}
protected void autoFillGroupBy(CandidateQuery query) {
if (query.getGroupBy() != null) {
return; // 已经填好了
}
// 如果只有一个group by,那么就用这个
Map> groupByMap = this.getGroupByMap(query.getTargetType());
if (groupByMap == null || groupByMap.size() != 1) {
return; // 算不来
}
List fields = groupByMap.values().iterator().next();
if (fields == null || fields.size() != 1) {
return; // 无法确定
}
query.setGroupBy(fields.get(0));
}
// 1. 必须; 2. 是已知类型
protected void checkTargetParams(CandidateQuery orginalQuery, CandidateQuery query) throws Exception {
if (TextUtil.isBlank(query.getTargetType())) {
throw new Exception("您未指定查询的目标类型");
}
if (getDataByType(query.getTargetType()) == null) {
throw new Exception(orginalQuery.getTargetType()+"不是本系统内定义的类型");
}
}
// 1. type和ID必须同时提供; 2. type必须是已知类型
protected void fixOwnerParam(CandidateQuery query) {
if (TextUtil.isBlank(query.getOwnerId()) || TextUtil.isBlank(query.getOwnerType())) {
query.setOwnerType(null);
query.setOwnerId(null);
return;
}
if (getDataByType(query.getOwnerType()) == null) {
query.setOwnerType(null);
query.setOwnerId(null);
return;
}
}
// 1. type 必须是已知类型
protected void fixListParam(CandidateQuery query) {
if (TextUtil.isBlank(query.getListType())) {
query.setListType(null);
query.setOwnerRoleInList(null);
return;
}
if (getDataByType(query.getListType()) == null) {
query.setListType(null);
query.setOwnerRoleInList(null);
return;
}
}
// 1. 必须是target中的某个字段
protected void fixGroupByParam(CandidateQuery query) {
if (TextUtil.isBlank(query.getGroupBy())) {
query.setGroupBy(null);
return;
}
if (!typeHasField(query.getTargetType(), query.getGroupBy())) {
query.setGroupBy(null);
return;
}
}
// 1. owner参数要正常 2. 必须是已知的对象字段名 (target里的字段,指向Owner类型)"
protected void fixOwnerRoleInTarget(CandidateQuery query) {
if (query.getOwnerType() == null || TextUtil.isBlank(query.getOwnerRoleInTarget())) {
query.setOwnerRoleInTarget(null);
return;
}
if (!hasCorrentOwnerRole(query)) {
query.setOwnerRoleInTarget(null);
}
}
// 1. owner参数要正常 2. 必须是已知的对象字段名 (target里的字段,指向Owner类型)"
protected void fixOwnerRoleInList(CandidateQuery query) {
if (query.getOwnerType() == null || query.getListType() == null || TextUtil.isBlank(query.getOwnerRoleInList())) {
query.setOwnerRoleInList(null);
return;
}
if (!hasCorrectOwnerRoleInList(query)) {
query.setOwnerRoleInList(null);
return;
}
}
// 1. owner参数要正常 2. 必须是已知的对象字段名 (target里的字段,指向Owner类型)"
protected void fixTargetRoleInList(CandidateQuery query) {
if (query.getTargetType() == null || query.getListType() == null || TextUtil.isBlank(query.getTargetRoleInList())) {
query.setTargetRoleInList(null);
return;
}
if (!hasCorrectReferRoleByList(query)) {
query.setOwnerRoleInList(null);
return;
}
}
// 自动填充 ownerRoleInTarget
protected void autoFillOwnerRoleInTarget(CandidateQuery query) {
if (query.getOwnerType() == null) {
return; // 不用填
}
if (query.getOwnerRoleInTarget() != null) {
return; // 已经有了
}
// 如果有指定的 owner 和 target 关系 且只有一个, 就用这个
Map> rtMap = this.getReferToMap(query.getTargetType());
if (rtMap == null) {
return; // 无法计算
}
List referToField = rtMap.get(query.getOwnerType());
if (referToField == null) {
return; // 无法计算
}
query.setOwnerRoleInTarget(String.join(",",referToField));
}
// 自动填充 ownerRoleInList
protected void autoFillOwnerRoleInList(CandidateQuery query) {
if (query.getOwnerType() == null || query.getListType() == null) {
return; // 不用填
}
if (query.getOwnerRoleInList() != null) {
return; // 已经有了
}
// 如果有指定的 owner 和 list 关系 且只有一个, 就用这个
Map> rtMap = this.getReferToMap(query.getListType());
if (rtMap == null) {
return; // 无法计算
}
List referToField = rtMap.get(query.getOwnerType());
if (referToField == null) {
return; // 无法计算
}
query.setOwnerRoleInList(String.join(",", referToField));
}
// 自动填充 targetRoleInList
protected void autoFillTargetRoleInList(CandidateQuery query) {
if (query.getListType() == null || query.getTargetRoleInList() != null) {
return;
}
// 如果指定的 list 只有一个字段引用 target, 就用这个
Map> rtMap = this.getReferToMap(query.getListType());
if (rtMap == null || rtMap.size() != 1) {
return; // 无法计算
}
List referToField = rtMap.get(query.getTargetType());
if (referToField == null || referToField.size() != 1) {
return; // 无法计算
}
query.setTargetRoleInList(referToField.get(0));
}
// 检查指定的类型 typeName 中是否有字段 fieldName
protected boolean typeHasField(String typeName, String fieldName) {
Map data = getDataByType(typeName);
if (data == null) {
return false;
}
String fields = (String) data.get("fields");
if (fields != null && fields.contains(";"+fieldName+";")) {
return true;
}
Map> referToMap = this.getReferToMap(typeName);
return referToMap.values().stream().flatMap(list->list.stream()).anyMatch(fname->fname.equals(fieldName));
}
protected CandidateQuery getQueryFromInput(CandidateQuery orginalQuery) throws Exception {
CandidateQuery query = new CandidateQuery();
query.setTargetType(normalizeModelName(orginalQuery.getTargetType()));
query.setFilterKey(orginalQuery.getFilterKey());
query.setGroupBy(normalizeModelName(orginalQuery.getGroupBy()));
query.setOwnerType(normalizeModelName(orginalQuery.getOwnerType()));
query.setOwnerId(orginalQuery.getOwnerId());
query.setListType(normalizeModelName(orginalQuery.getListType()));
query.setScenceCode(orginalQuery.getScenceCode());
query.setOwnerRoleInList(normalizeModelName(orginalQuery.getOwnerRoleInList()));
query.setOwnerRoleInTarget(normalizeModelName(orginalQuery.getOwnerRoleInTarget()));
query.setTargetRoleInList(normalizeModelName(orginalQuery.getTargetRoleInList()));
query.setPageNo(Math.max(1,orginalQuery.getPageNo()));
query.setPageSize(Math.max(orginalQuery.getPageSize(), 20));
return query;
}
protected void fixOwnerInListParam(CandidateQuery query) {
if (!isCorrectListInOwner(query)) {
query.setListType(null);
query.setOwnerRoleInList(null);
} else if (!hasCorrectOwnerRoleInList(query)) {
query.setOwnerRoleInList(null);
}
}
protected String getGroupByBame(CandidateQuery query) {
Map> data = getGroupByMap(query.getTargetType());
if (data == null || data.isEmpty()) {
return null;
}
return data.keySet().iterator().next();
}
protected boolean isCorrectGroupByParam(CandidateQuery query) {
if (query.getGroupBy() == null) {
return false;
}
// 有指定, 但是可能是普通字段,也可能是对象属性
if (query.getGroupBy() != null) {
return true;
}
Map> groupByMap = getGroupByMap(query.getTargetType());
if (groupByMap == null || query.getGroupBy() == null) {
return false;
}
return groupByMap.containsKey(query.getGroupBy());
}
protected boolean isCorrectListInOwner(CandidateQuery query) {
Map> listReferToMap = getReferToMap(query.getListType());
if (listReferToMap == null || query.getOwnerType() == null) {
return false;
}
return listReferToMap.containsKey(query.getOwnerType());
}
protected boolean hasCorrectOwnerRoleInList(CandidateQuery query) {
Map> listReferToMap = getReferToMap(query.getListType());
if (listReferToMap == null || query.getOwnerType() == null || query.getOwnerRoleInList() == null) {
return false;
}
List roles = listReferToMap.get(query.getOwnerType());
if (roles == null) {
return false;
}
return roles.contains(query.getOwnerRoleInList());
}
protected boolean isCorrectReferByList(CandidateQuery query) {
Map> usedInMap = getUsedInMap(query.getTargetType());
if (usedInMap == null || query.getListType() == null) {
return false;
}
return usedInMap.containsKey(query.getListType());
}
protected boolean hasCorrectReferRoleByList(CandidateQuery query) {
Map> usedInMap = getUsedInMap(query.getTargetType());
if (usedInMap == null || query.getListType() == null || query.getTargetRoleInList() == null) {
return false;
}
List roles = usedInMap.get(query.getListType());
if (roles == null) {
return false;
}
return roles.contains(query.getTargetRoleInList());
}
protected boolean hasCorrentOwnerRole(CandidateQuery query) {
Map> referToMap = getReferToMap(query.getTargetType());
if (referToMap == null || query.getOwnerType() == null || query.getOwnerRoleInTarget() == null) {
return false;
}
List roles = referToMap.get(query.getOwnerType());
return roles.contains(query.getOwnerRoleInTarget());
}
protected Map> getReferToMap(String targetType) {
return getListDataByRelationType(targetType, "referTo");
}
protected Map> getUsedInMap(String targetType) {
return getListDataByRelationType(targetType, "usedIn");
}
protected Map> getGroupByMap(String targetType) {
return getListDataByRelationType(targetType, "groupBy");
}
protected Map> getListDataByRelationType(String targetType, String relationType) {
Map data = getDataByType(targetType);
if (data == null) {
return null;
}
return (Map>) data.get(relationType);
}
protected Map getDataByType(String targetType) {
if (targetType == null) {
return null;
}
Map data = QUERY_RELATIONS.get(targetType);
return data;
}
/**
* assign 场景下, 忽略List的任何信息, 所以只需要考虑 ownerRoleInTarget
* @param query
* @param params
* @return
*/
protected String prepareSqlForAssign(CandidateQuery query, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy