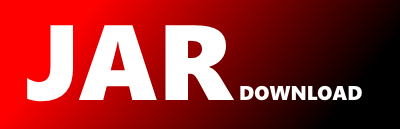
flexjson.BeanAnalyzer Maven / Gradle / Ivy
package flexjson;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
public class BeanAnalyzer
{
private static ThreadLocal
© 2015 - 2025 Weber Informatics LLC | Privacy Policy