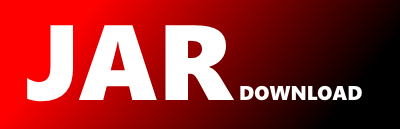
main.java.com.dragome.web.services.RequestExecutorImpl Maven / Gradle / Ivy
/*
* Copyright (c) 2011-2014 Fernando Petrola
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.dragome.web.services;
import java.io.Serializable;
import java.lang.reflect.Proxy;
import java.util.Map;
import java.util.Map.Entry;
import com.dragome.commons.ExecutionHandler;
import com.dragome.commons.javascript.ScriptHelper;
import com.dragome.services.AsyncCallbackWrapper;
import com.dragome.services.ServiceLocator;
import com.dragome.services.WebServiceLocator;
import com.dragome.services.interfaces.AsyncCallback;
import com.dragome.services.interfaces.AsyncResponseHandler;
import com.dragome.services.interfaces.RequestExecutor;
import com.dragome.web.debugging.messages.ClientToServerServiceInvocationHandler;
public class RequestExecutorImpl implements RequestExecutor
{
private boolean useGetMethod;
public RequestExecutorImpl(boolean useGetMethod)
{
this.useGetMethod= useGetMethod;
}
public String executeSynchronousRequest(String url, Map parameters)
{
final ExecutionHandler executionHandler= ServiceLocator.getInstance().getConfigurator().getExecutionHandler();
if (executionHandler.canSuspend())
{
final String[] resultArray= new String[] { "" };
AsyncCallback asyncCallbackToContinue= new AsyncCallback()
{
public void onSuccess(String result)
{
resultArray[0]= result;
executionHandler.continueExecution();
}
public void onError()
{
throw new RuntimeException("Error in async call");
}
};
AsyncCallback wrappedCallback= wrapCallback(Serializable.class, asyncCallbackToContinue);
executeHttpRequest(true, url, parameters, wrappedCallback, false, useGetMethod);
executionHandler.suspendExecution();
return resultArray[0];
}
else
return executeHttpRequest(false, url, parameters, null, false, useGetMethod);
}
public String executeFixedSynchronousRequest(String url, Map parameters)
{
return executeHttpRequest(false, url, parameters, null, false, useGetMethod);
}
public static String executeHttpRequest(boolean isAsync, String url, Map parameters, AsyncCallback asyncCallback, boolean crossDomain, boolean useGetMethod)
{
ScriptHelper.put("url", url, null);
String isAsyncAsString= isAsync ? "true" : "false";
String isCrossDomain= crossDomain ? "true" : "false";
ScriptHelper.put("asyncCall", isAsyncAsString, null);
ScriptHelper.put("asyncCallback", asyncCallback, null);
ScriptHelper.put("crossDomain", isCrossDomain, null);
ScriptHelper.put("useGetMethod", useGetMethod, null);
ScriptHelper.put("parameters2", new Object(), null);
ScriptHelper.evalNoResult("parameters2={}", null);
if (parameters != null)
for (Entry entry : parameters.entrySet())
{
ScriptHelper.put("key", entry.getKey(), null);
ScriptHelper.put("value", entry.getValue(), null);
ScriptHelper.evalNoResult("parameters2[key]=value", null);
}
Object eval= ScriptHelper.eval("jQueryHttpRequest(asyncCall, url,parameters2,asyncCallback, crossDomain, useGetMethod)", null);
return isAsync ? "" : (String) eval;
}
public String executeAsynchronousRequest(String url, Map parameters, AsyncCallback asyncCallback)
{
AsyncCallback wrappedCallback= wrapCallback(Serializable.class, asyncCallback);
return executeHttpRequest(true, url, parameters, wrappedCallback, false, useGetMethod);
}
public static AsyncCallback wrapCallback(final Class type, final AsyncCallback asyncCallback)
{
AsyncCallback result;
if (WebServiceLocator.getInstance().isRemoteDebugging())
{
result= new AsyncCallbackWrapper(asyncCallback)
{
public void onError()
{
asyncCallback.onError();
}
public void onSuccess(S result)
{
AsyncResponseHandler asyncResponseHandler= createRemoteServiceByWebSocket(AsyncResponseHandler.class);
String id= (String) ScriptHelper.eval("this.javaId", this);
asyncResponseHandler.pushResponse((String) result, id);
}
};
}
else
result= (AsyncCallback) asyncCallback;
return result;
}
public static T createRemoteServiceByWebSocket(Class type)
{
return (T) Proxy.newProxyInstance(RequestExecutorImpl.class.getClassLoader(), new Class[] { type }, new ClientToServerServiceInvocationHandler(type));
}
public void executeCrossDomainAsynchronousRequest(String url, Map parameters, AsyncCallback asyncCallback)
{
AsyncCallback wrappedCallback= wrapCallback(Serializable.class, asyncCallback);
executeHttpRequest(true, url, parameters, wrappedCallback, true, useGetMethod);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy