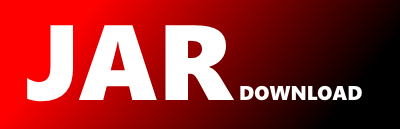
com.dropbox.sign.api.BulkSendJobApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dropbox-sign Show documentation
Show all versions of dropbox-sign Show documentation
Use the Dropbox Sign Java SDK to connect your Java app to the service of Dropbox Sign in microseconds!
The newest version!
package com.dropbox.sign.api;
import com.dropbox.sign.ApiException;
import com.dropbox.sign.ApiClient;
import com.dropbox.sign.ApiResponse;
import com.dropbox.sign.Configuration;
import com.dropbox.sign.Pair;
import jakarta.ws.rs.core.GenericType;
import com.dropbox.sign.model.BulkSendJobGetResponse;
import com.dropbox.sign.model.BulkSendJobListResponse;
import com.dropbox.sign.model.ErrorResponse;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.8.0")
public class BulkSendJobApi {
private ApiClient apiClient;
public BulkSendJobApi() {
this(Configuration.getDefaultApiClient());
}
public BulkSendJobApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get the API client
*
* @return API client
*/
public ApiClient getApiClient() {
return apiClient;
}
/**
* Set the API client
*
* @param apiClient an instance of API client
*/
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get Bulk Send Job.
* Returns the status of the BulkSendJob and its SignatureRequests specified by the `bulk_send_job_id` parameter.
* @param bulkSendJobId The id of the BulkSendJob to retrieve. (required)
* @param page Which page number of the BulkSendJob list to return. Defaults to `1`. (optional, default to 1)
* @param pageSize Number of objects to be returned per page. Must be between `1` and `100`. Default is 20. (optional, default to 20)
* @return BulkSendJobGetResponse
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 successful operation * X-RateLimit-Limit -
* X-RateLimit-Remaining -
* X-Ratelimit-Reset -
4XX failed_operation -
*/
public BulkSendJobGetResponse bulkSendJobGet(String bulkSendJobId, Integer page, Integer pageSize) throws ApiException {
return bulkSendJobGetWithHttpInfo(bulkSendJobId, page, pageSize).getData();
}
/**
* @see BulkSendJobApi#bulkSendJobGet(String, Integer, Integer)
*/
public BulkSendJobGetResponse bulkSendJobGet(String bulkSendJobId) throws ApiException {
Integer page = 1;
Integer pageSize = 20;
return bulkSendJobGetWithHttpInfo(bulkSendJobId, page, pageSize).getData();
}
/**
* @see BulkSendJobApi#bulkSendJobGetWithHttpInfo(String, Integer, Integer)
*/
public ApiResponse bulkSendJobGetWithHttpInfo(String bulkSendJobId) throws ApiException {
Integer page = 1;
Integer pageSize = 20;
return bulkSendJobGetWithHttpInfo(bulkSendJobId, page, pageSize);
}
/**
* @see BulkSendJobApi#bulkSendJobGet(String, Integer, Integer)
*/
public BulkSendJobGetResponse bulkSendJobGet(String bulkSendJobId, Integer page) throws ApiException {
Integer pageSize = 20;
return bulkSendJobGetWithHttpInfo(bulkSendJobId, page, pageSize).getData();
}
/**
* @see BulkSendJobApi#bulkSendJobGetWithHttpInfo(String, Integer, Integer)
*/
public ApiResponse bulkSendJobGetWithHttpInfo(String bulkSendJobId, Integer page) throws ApiException {
Integer pageSize = 20;
return bulkSendJobGetWithHttpInfo(bulkSendJobId, page, pageSize);
}
/**
* Get Bulk Send Job.
* Returns the status of the BulkSendJob and its SignatureRequests specified by the `bulk_send_job_id` parameter.
* @param bulkSendJobId The id of the BulkSendJob to retrieve. (required)
* @param page Which page number of the BulkSendJob list to return. Defaults to `1`. (optional, default to 1)
* @param pageSize Number of objects to be returned per page. Must be between `1` and `100`. Default is 20. (optional, default to 20)
* @return ApiResponse<BulkSendJobGetResponse>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 successful operation * X-RateLimit-Limit -
* X-RateLimit-Remaining -
* X-Ratelimit-Reset -
4XX failed_operation -
*/
public ApiResponse bulkSendJobGetWithHttpInfo(String bulkSendJobId, Integer page, Integer pageSize) throws ApiException {
if (page == null) {
page = 1;
}
if (pageSize == null) {
pageSize = 20;
}
// Check required parameters
if (bulkSendJobId == null) {
throw new ApiException(400, "Missing the required parameter 'bulkSendJobId' when calling bulkSendJobGet");
}
// Path parameters
String localVarPath = "/bulk_send_job/{bulk_send_job_id}"
.replaceAll("\\{bulk_send_job_id}", apiClient.escapeString(bulkSendJobId.toString()));
// Query parameters
List localVarQueryParams = new ArrayList<>(
apiClient.parameterToPairs("", "page", page)
);
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page_size", pageSize));
String localVarAccept = apiClient.selectHeaderAccept("application/json");
Map localVarFormParams = new LinkedHashMap<>();
localVarFormParams = new HashMap();
boolean isFileTypeFound = !localVarFormParams.isEmpty();
String localVarContentType = isFileTypeFound? "multipart/form-data" : apiClient.selectHeaderContentType();
String[] localVarAuthNames = new String[] {"api_key", "oauth2"};
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(
"BulkSendJobApi.bulkSendJobGet",
localVarPath,
"GET",
localVarQueryParams,
null,
new LinkedHashMap<>(),
new LinkedHashMap<>(),
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType,
false
);
}
/**
* List Bulk Send Jobs.
* Returns a list of BulkSendJob that you can access.
* @param page Which page number of the BulkSendJob List to return. Defaults to `1`. (optional, default to 1)
* @param pageSize Number of objects to be returned per page. Must be between `1` and `100`. Default is 20. (optional, default to 20)
* @return BulkSendJobListResponse
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 successful operation * X-RateLimit-Limit -
* X-RateLimit-Remaining -
* X-Ratelimit-Reset -
4XX failed_operation -
*/
public BulkSendJobListResponse bulkSendJobList(Integer page, Integer pageSize) throws ApiException {
return bulkSendJobListWithHttpInfo(page, pageSize).getData();
}
/**
* @see BulkSendJobApi#bulkSendJobList(Integer, Integer)
*/
public BulkSendJobListResponse bulkSendJobList() throws ApiException {
Integer page = 1;
Integer pageSize = 20;
return bulkSendJobListWithHttpInfo(page, pageSize).getData();
}
/**
* @see BulkSendJobApi#bulkSendJobListWithHttpInfo(Integer, Integer)
*/
public ApiResponse bulkSendJobListWithHttpInfo() throws ApiException {
Integer page = 1;
Integer pageSize = 20;
return bulkSendJobListWithHttpInfo(page, pageSize);
}
/**
* @see BulkSendJobApi#bulkSendJobList(Integer, Integer)
*/
public BulkSendJobListResponse bulkSendJobList(Integer page) throws ApiException {
Integer pageSize = 20;
return bulkSendJobListWithHttpInfo(page, pageSize).getData();
}
/**
* @see BulkSendJobApi#bulkSendJobListWithHttpInfo(Integer, Integer)
*/
public ApiResponse bulkSendJobListWithHttpInfo(Integer page) throws ApiException {
Integer pageSize = 20;
return bulkSendJobListWithHttpInfo(page, pageSize);
}
/**
* List Bulk Send Jobs.
* Returns a list of BulkSendJob that you can access.
* @param page Which page number of the BulkSendJob List to return. Defaults to `1`. (optional, default to 1)
* @param pageSize Number of objects to be returned per page. Must be between `1` and `100`. Default is 20. (optional, default to 20)
* @return ApiResponse<BulkSendJobListResponse>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 successful operation * X-RateLimit-Limit -
* X-RateLimit-Remaining -
* X-Ratelimit-Reset -
4XX failed_operation -
*/
public ApiResponse bulkSendJobListWithHttpInfo(Integer page, Integer pageSize) throws ApiException {
if (page == null) {
page = 1;
}
if (pageSize == null) {
pageSize = 20;
}
// Query parameters
List localVarQueryParams = new ArrayList<>(
apiClient.parameterToPairs("", "page", page)
);
localVarQueryParams.addAll(apiClient.parameterToPairs("", "page_size", pageSize));
String localVarAccept = apiClient.selectHeaderAccept("application/json");
Map localVarFormParams = new LinkedHashMap<>();
localVarFormParams = new HashMap();
boolean isFileTypeFound = !localVarFormParams.isEmpty();
String localVarContentType = isFileTypeFound? "multipart/form-data" : apiClient.selectHeaderContentType();
String[] localVarAuthNames = new String[] {"api_key", "oauth2"};
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(
"BulkSendJobApi.bulkSendJobList",
"/bulk_send_job/list",
"GET",
localVarQueryParams,
null,
new LinkedHashMap<>(),
new LinkedHashMap<>(),
localVarFormParams,
localVarAccept,
localVarContentType,
localVarAuthNames,
localVarReturnType,
false
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy