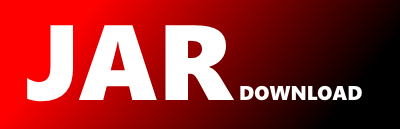
com.dropbox.sign.api.OAuthApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dropbox-sign Show documentation
Show all versions of dropbox-sign Show documentation
Use the Dropbox Sign Java SDK to connect your Java app to the service of Dropbox Sign in microseconds!
The newest version!
package com.dropbox.sign.api;
import com.dropbox.sign.ApiException;
import com.dropbox.sign.ApiClient;
import com.dropbox.sign.ApiResponse;
import com.dropbox.sign.Configuration;
import com.dropbox.sign.Pair;
import jakarta.ws.rs.core.GenericType;
import com.dropbox.sign.model.ErrorResponse;
import com.dropbox.sign.model.OAuthTokenGenerateRequest;
import com.dropbox.sign.model.OAuthTokenRefreshRequest;
import com.dropbox.sign.model.OAuthTokenResponse;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", comments = "Generator version: 7.8.0")
public class OAuthApi {
private ApiClient apiClient;
public OAuthApi() {
this(Configuration.getDefaultApiClient());
}
public OAuthApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Get the API client
*
* @return API client
*/
public ApiClient getApiClient() {
return apiClient;
}
/**
* Set the API client
*
* @param apiClient an instance of API client
*/
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* OAuth Token Generate.
* Once you have retrieved the code from the user callback, you will need to exchange it for an access token via a backend call.
* @param oauthTokenGenerateRequest (required)
* @return OAuthTokenResponse
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 successful operation * X-RateLimit-Limit -
* X-RateLimit-Remaining -
* X-Ratelimit-Reset -
4XX failed_operation -
*/
public OAuthTokenResponse oauthTokenGenerate(OAuthTokenGenerateRequest oauthTokenGenerateRequest) throws ApiException {
return oauthTokenGenerateWithHttpInfo(oauthTokenGenerateRequest).getData();
}
/**
* OAuth Token Generate.
* Once you have retrieved the code from the user callback, you will need to exchange it for an access token via a backend call.
* @param oauthTokenGenerateRequest (required)
* @return ApiResponse<OAuthTokenResponse>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 successful operation * X-RateLimit-Limit -
* X-RateLimit-Remaining -
* X-Ratelimit-Reset -
4XX failed_operation -
*/
public ApiResponse oauthTokenGenerateWithHttpInfo(OAuthTokenGenerateRequest oauthTokenGenerateRequest) throws ApiException {
// Check required parameters
if (oauthTokenGenerateRequest == null) {
throw new ApiException(400, "Missing the required parameter 'oauthTokenGenerateRequest' when calling oauthTokenGenerate");
}
String localVarAccept = apiClient.selectHeaderAccept("application/json");
Map localVarFormParams = new LinkedHashMap<>();
localVarFormParams = oauthTokenGenerateRequest.createFormData();
boolean isFileTypeFound = !localVarFormParams.isEmpty();
String localVarContentType = isFileTypeFound? "multipart/form-data" : apiClient.selectHeaderContentType("application/json");
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(
"OAuthApi.oauthTokenGenerate",
"/oauth/token",
"POST",
new ArrayList<>(),
isFileTypeFound ? null : oauthTokenGenerateRequest,
new LinkedHashMap<>(),
new LinkedHashMap<>(),
localVarFormParams,
localVarAccept,
localVarContentType,
null,
localVarReturnType,
false
);
}
/**
* OAuth Token Refresh.
* Access tokens are only valid for a given period of time (typically one hour) for security reasons. Whenever acquiring an new access token its TTL is also given (see `expires_in`), along with a refresh token that can be used to acquire a new access token after the current one has expired.
* @param oauthTokenRefreshRequest (required)
* @return OAuthTokenResponse
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 successful operation * X-RateLimit-Limit -
* X-RateLimit-Remaining -
* X-Ratelimit-Reset -
4XX failed_operation -
*/
public OAuthTokenResponse oauthTokenRefresh(OAuthTokenRefreshRequest oauthTokenRefreshRequest) throws ApiException {
return oauthTokenRefreshWithHttpInfo(oauthTokenRefreshRequest).getData();
}
/**
* OAuth Token Refresh.
* Access tokens are only valid for a given period of time (typically one hour) for security reasons. Whenever acquiring an new access token its TTL is also given (see `expires_in`), along with a refresh token that can be used to acquire a new access token after the current one has expired.
* @param oauthTokenRefreshRequest (required)
* @return ApiResponse<OAuthTokenResponse>
* @throws ApiException if fails to make API call
* @http.response.details
Status Code Description Response Headers
200 successful operation * X-RateLimit-Limit -
* X-RateLimit-Remaining -
* X-Ratelimit-Reset -
4XX failed_operation -
*/
public ApiResponse oauthTokenRefreshWithHttpInfo(OAuthTokenRefreshRequest oauthTokenRefreshRequest) throws ApiException {
// Check required parameters
if (oauthTokenRefreshRequest == null) {
throw new ApiException(400, "Missing the required parameter 'oauthTokenRefreshRequest' when calling oauthTokenRefresh");
}
String localVarAccept = apiClient.selectHeaderAccept("application/json");
Map localVarFormParams = new LinkedHashMap<>();
localVarFormParams = oauthTokenRefreshRequest.createFormData();
boolean isFileTypeFound = !localVarFormParams.isEmpty();
String localVarContentType = isFileTypeFound? "multipart/form-data" : apiClient.selectHeaderContentType("application/json");
GenericType localVarReturnType = new GenericType() {};
return apiClient.invokeAPI(
"OAuthApi.oauthTokenRefresh",
"/oauth/token?refresh",
"POST",
new ArrayList<>(),
isFileTypeFound ? null : oauthTokenRefreshRequest,
new LinkedHashMap<>(),
new LinkedHashMap<>(),
localVarFormParams,
localVarAccept,
localVarContentType,
null,
localVarReturnType,
false
);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy