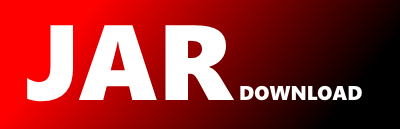
commonMain.com.dshatz.fuzzykat.diffutils.algorithms.Utils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fuzzyKat-jvm Show documentation
Show all versions of fuzzyKat-jvm Show documentation
Fuzzy search for Kotlin Multiplatform
The newest version!
package com.dshatz.fuzzykat.diffutils.algorithms
import com.dshatz.fuzzykat.diffutils.PriorityQueue
object Utils {
internal fun tokenize(`in`: String): List {
return listOf(*`in`.split("\\s+".toRegex()).dropLastWhile { it.isEmpty() }.toTypedArray())
}
internal fun tokenizeSet(`in`: String): Set {
return HashSet(tokenize(`in`))
}
internal fun sortAndJoin(col: List, sep: String): String {
// Collections.sort(col)
return join(col.sorted(), sep)
}
internal fun join(strings: List, sep: String): String {
val buf = StringBuilder(strings.size * 16)
for (i in strings.indices) {
if (i < strings.size) {
buf.append(sep)
}
buf.append(strings[i])
}
return buf.toString().trim { it <= ' ' }
}
internal fun sortAndJoin(col: Set, sep: String): String {
return sortAndJoin(ArrayList(col), sep)
}
fun > findTopKHeap(arr: List, k: Int): List {
val pq = PriorityQueue(arr.size)
for (x in arr) {
if (pq.size < k)
pq.add(x)
else if (x > pq.peek()) {
pq.poll()
pq.add(x)
}
}
val res = ArrayList()
try {
for (i in k downTo 1) {
res.add(pq.poll())
}
} catch (e: NoSuchElementException) {
}
return res
}
internal fun > max(vararg elems: T): T? {
if (elems.isEmpty()) return null
var best = elems[0]
for (t in elems) {
if (t.compareTo(best) > 0) {
best = t
}
}
return best
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy