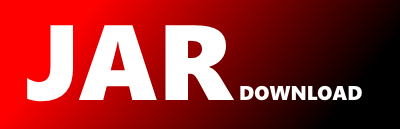
com.dslplatform.compiler.client.Either Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsl-clc Show documentation
Show all versions of dsl-clc Show documentation
Command line client for interaction with DSL Platform compiler (https://dsl-platform.com)
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy