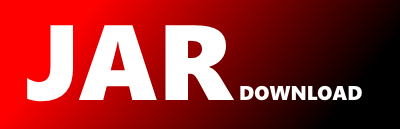
com.dslplatform.mojo.MojoContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsl-platform-maven-plugin Show documentation
Show all versions of dsl-platform-maven-plugin Show documentation
A Maven plugin for interaction with DSL Platform command line client API (https://dsl-platform.com)
package com.dslplatform.mojo;
import com.dslplatform.compiler.client.CompileParameter;
import com.dslplatform.compiler.client.Context;
import com.dslplatform.compiler.client.parameters.Settings;
import com.dslplatform.compiler.client.parameters.Targets;
import org.apache.maven.plugin.logging.Log;
import java.util.List;
import java.util.Map;
class MojoContext extends Context {
final StringBuilder errorLog = new StringBuilder();
final Log log;
MojoContext(Log log) {
super(System.out);
this.log = log;
}
public MojoContext with(Settings.Option option) {
this.put(option.toString(), null);
return this;
}
public MojoContext with(CompileParameter parameter) {
this.put(parameter, null);
return this;
}
public MojoContext with(CompileParameter parameter, String value) {
this.put(parameter, value);
return this;
}
public MojoContext with(String option) {
this.put(option, null);
return this;
}
public MojoContext with(String option, String value) {
this.put(option, value);
return this;
}
public MojoContext with(List settings) {
if (settings != null) {
for (Settings.Option option : settings)
this.with(option);
}
return this;
}
public MojoContext with(Map compileParameters) {
if (compileParameters != null)
for (Map.Entry kv : compileParameters.entrySet()) {
K parameter = kv.getKey();
String value = kv.getValue();
if (parameter instanceof CompileParameter)
this.put((CompileParameter) parameter, value);
if (parameter instanceof Targets.Option)
this.put(parameter.toString(), value);
}
return this;
}
public void show(String... values) {
if (log.isInfoEnabled()) {
for (String v : values) {
log.info(v);
}
}
}
public void log(String value) {
if (log.isDebugEnabled()) {
log.debug(value);
}
}
public void log(char[] value, int len) {
if (log.isDebugEnabled()) {
log.debug(new String(value, 0, len));
}
}
public void warning(String value) {
errorLog.append(value + "\n");
if (log.isWarnEnabled()) {
log.warn(value);
}
}
public void warning(Exception ex) {
errorLog.append(ex.getMessage());
if (log.isWarnEnabled()) {
log.warn(ex);
}
}
public void error(String value) {
errorLog.append(value + "\n");
if (log.isErrorEnabled()) {
log.error(value);
}
}
public void error(Exception ex) {
errorLog.append(ex.getMessage());
if (log.isErrorEnabled()) {
log.error(ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy