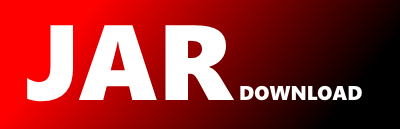
com.dua3.utility.options.StandardOption Maven / Gradle / Ivy
package com.dua3.utility.options;
import com.dua3.utility.data.DataUtil;
import java.util.Collection;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* Standard option class.
*
* @param the option's argument type.
*/
public final class StandardOption extends Option {
/**
* Construct new StandardOption instance.
*
* @param mapper the mapper used to convert the string values of arguments to the target type
* @param names the names to be used on the command line for this option
*/
private StandardOption(Function mapper,
Function super T, String> formatter,
String... names) {
super(mapper, formatter, names);
}
/**
* Create a new instance of StandardOption.
*
* @param type the target type of the option
* @param names the names to be used on the command line for this option
* @param the type parameter
* @return a new instance of StandardOption
*/
public static StandardOption create(Class extends T> type,
String... names) {
return create(s -> DataUtil.convert(s, type), v -> DataUtil.convert(v, String.class), names);
}
/**
* Create a new instance of StandardOption.
*
* @param mapper a function that converts the command line value to the target type of the option
* @param names the names to be used on the command line for this option
* @param the type parameter
* @return a new instance of StandardOption
*/
public static StandardOption create(Function mapper,
String[] names) {
return new StandardOption<>(mapper, Object::toString, names);
}
/**
* Create a new instance of StandardOption.
*
* @param mapper a function that converts the command line value to the target type of the option
* @param formatter a function that formats the target type of the option to a string
* @param names the names to be used on the command line for this option
* @param the type parameter
* @return a new instance of StandardOption
*/
public static StandardOption create(Function mapper,
Function super T, String> formatter,
String[] names) {
return new StandardOption<>(mapper, formatter, names);
}
@Override
public StandardOption description(String description) {
super.description(description);
return this;
}
@Override
public StandardOption displayName(String displayName) {
super.description(displayName);
return this;
}
@Override
public StandardOption handler(Consumer> handler) {
super.handler(handler);
return this;
}
/**
* Set the number of occurrences for this option. This method sets the minimum and maximum number of occurrences to
* the same value, i.e. calling {@code occurrence(n)} means the option has to be passed exactly {@code n} times.
*
* @param n the number of occurrences to set
* @return the option
*/
public StandardOption occurrence(int n) {
return occurrence(n, n);
}
/**
* Set the number of occurrences for this option.
*
* @param min the minimum number of occurrences
* @param max the maximum number of occurrences
* @return the option
*/
@Override
public StandardOption occurrence(int min, int max) {
super.occurrence(min, max);
return this;
}
/**
* Set the arity for this option. This method sets the minimum and maximum arity to the same
* value, i.e. calling {@code arity(n)} means the option takes exactly {@code n} arguments for each invocation.
*
* @param n the arity to set
* @return the option
*/
public StandardOption arity(int n) {
return arity(n, n);
}
/**
* Set the arity for this option.
*
* @param min the minimum arity
* @param max the maximum arity
* @return the option
*/
@Override
public StandardOption arity(int min, int max) {
super.arity(min, max);
return this;
}
/**
* Set the arity for this option.
*
* @param min the minimum arity
* @return the option
*/
public StandardOption minArity(int min) {
super.arity(min, Integer.MAX_VALUE);
return this;
}
@Override
public Optional getDefault() {
return Optional.empty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy