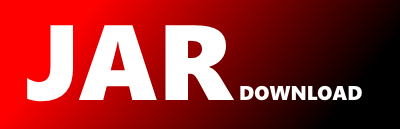
com.dubture.getcomposer.core.objects.Config Maven / Gradle / Ivy
package com.dubture.getcomposer.core.objects;
import com.dubture.getcomposer.core.collection.JsonArray;
/**
* Represents a config entity in a composer package
*
* @see http://getcomposer.org/doc/04-schema.md#config
* @author Thomas Gossmann
*
*/
public class Config extends JsonObject {
/**
* Returns the vendor-bin
property.
*
* @return the vendor-bin
property
*/
public String getVendorDir() {
return getAsString("vendor-dir");
}
/**
* Sets the vendor-bin
property.
*
* @param vendorDir the new vendor-bin
value
*/
public void setVendorDir(String vendorDir) {
set("vendor-dir", vendorDir);
}
/**
* Returns the bin-dir
property.
*
* @return the bin-dir
property
*/
public String getBinDir() {
return getAsString("bin-dir");
}
/**
* Sets the bin-dir
property.
*
* @param binDir the new bin-dir
value
*/
public void setBinDir(String binDir) {
set("bin-dir", binDir);
}
/**
* Returns the process-timeout
property.
*
* @return the process-timeout
property
*/
public Integer getProcessTimeout() {
return getAsInteger("process-timeout");
}
/**
* Sets the process-timeout
property.
*
* @param processTimeout the new process-timeout
value
*/
public void setProcessTimeout(int processTimeout) {
set("process-timeout", processTimeout);
}
/**
* Returns the github-protocols
property. If this property
* isn't present in the json, the default value
* ["git", "https", "http"]
is returned.
*
* @return the github-protocols
property
*/
public JsonArray getGithubProtocols() {
JsonArray protocols = getAsArray("github-protocols");
if (protocols == null) {
protocols = new JsonArray();
protocols.add("git");
protocols.add("https");
protocols.add("http");
}
return protocols;
}
/**
* Sets the github-protocols
property.
*
* @param githubProtocols the new github-protocols
value
*/
public void setGithubProtocols(JsonArray githubProtocols) {
set("github-protocols", githubProtocols);
}
/**
* Sets the notify-on-install
property. If this property
* isn't present in the json, the default value true
* is returned.
*
* @return the notify-on-install
property
*/
public boolean getNotifyOnInstall() {
if (has("notify-on-install")) {
return getAsBoolean("notify-on-install");
} else {
return true;
}
}
/**
* Set the notify-on-install
property.
*
* @param notifyOnInstall the new notify-on-install
value
*/
public void setNotifyOnInstall(boolean notifyOnInstall) {
set("notify-on-install", notifyOnInstall);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy