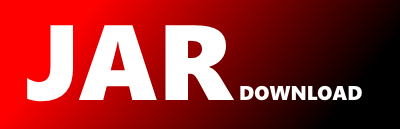
net.java.html.lib.knockout.KnockoutBindingHandlers Maven / Gradle / Ivy
package net.java.html.lib.knockout;
public class KnockoutBindingHandlers extends net.java.html.lib.Objs {
protected KnockoutBindingHandlers(net.java.html.lib.Objs.Constructor> c, java.lang.Object js) {
super(c, js);
}
private static final class $Constructor extends net.java.html.lib.Objs.Constructor {
$Constructor() {
super(KnockoutBindingHandlers.class);
}
@Override
public KnockoutBindingHandlers create(java.lang.Object obj) {
return obj == null ? null : new KnockoutBindingHandlers(this, obj);
}
};
private static final $Constructor $AS = new $Constructor();
public static KnockoutBindingHandlers $as(java.lang.Object obj) {
return $AS.create(obj);
}
public net.java.html.lib.knockout.KnockoutBindingHandler $get(java.lang.String bindingHandler) {
return (net.java.html.lib.knockout.KnockoutBindingHandler)net.java.html.lib.knockout.KnockoutBindingHandler.$as($Typings$.$get$11($js(this), bindingHandler));
}
// Controlling text and appearance
public net.java.html.lib.Objs.Property visible = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "visible");
public net.java.html.lib.knockout.KnockoutBindingHandler visible() { return visible.get(); }
public net.java.html.lib.Objs.Property text = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "text");
public net.java.html.lib.knockout.KnockoutBindingHandler text() { return text.get(); }
public net.java.html.lib.Objs.Property html = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "html");
public net.java.html.lib.knockout.KnockoutBindingHandler html() { return html.get(); }
public net.java.html.lib.Objs.Property css = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "css");
public net.java.html.lib.knockout.KnockoutBindingHandler css() { return css.get(); }
public net.java.html.lib.Objs.Property style = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "style");
public net.java.html.lib.knockout.KnockoutBindingHandler style() { return style.get(); }
public net.java.html.lib.Objs.Property attr = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "attr");
public net.java.html.lib.knockout.KnockoutBindingHandler attr() { return attr.get(); }
// Control Flow
public net.java.html.lib.Objs.Property foreach = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "foreach");
public net.java.html.lib.knockout.KnockoutBindingHandler foreach() { return foreach.get(); }
/* cannot generate if */
public net.java.html.lib.Objs.Property ifnot = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "ifnot");
public net.java.html.lib.knockout.KnockoutBindingHandler ifnot() { return ifnot.get(); }
public net.java.html.lib.Objs.Property with = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "with");
public net.java.html.lib.knockout.KnockoutBindingHandler with() { return with.get(); }
// Working with form fields
public net.java.html.lib.Objs.Property click = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "click");
public net.java.html.lib.knockout.KnockoutBindingHandler click() { return click.get(); }
public net.java.html.lib.Objs.Property event = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "event");
public net.java.html.lib.knockout.KnockoutBindingHandler event() { return event.get(); }
public net.java.html.lib.Objs.Property submit = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "submit");
public net.java.html.lib.knockout.KnockoutBindingHandler submit() { return submit.get(); }
public net.java.html.lib.Objs.Property enable = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "enable");
public net.java.html.lib.knockout.KnockoutBindingHandler enable() { return enable.get(); }
public net.java.html.lib.Objs.Property disable = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "disable");
public net.java.html.lib.knockout.KnockoutBindingHandler disable() { return disable.get(); }
public net.java.html.lib.Objs.Property value = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "value");
public net.java.html.lib.knockout.KnockoutBindingHandler value() { return value.get(); }
public net.java.html.lib.Objs.Property textInput = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "textInput");
public net.java.html.lib.knockout.KnockoutBindingHandler textInput() { return textInput.get(); }
public net.java.html.lib.Objs.Property hasfocus = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "hasfocus");
public net.java.html.lib.knockout.KnockoutBindingHandler hasfocus() { return hasfocus.get(); }
public net.java.html.lib.Objs.Property checked = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "checked");
public net.java.html.lib.knockout.KnockoutBindingHandler checked() { return checked.get(); }
public net.java.html.lib.Objs.Property options = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "options");
public net.java.html.lib.knockout.KnockoutBindingHandler options() { return options.get(); }
public net.java.html.lib.Objs.Property selectedOptions = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "selectedOptions");
public net.java.html.lib.knockout.KnockoutBindingHandler selectedOptions() { return selectedOptions.get(); }
public net.java.html.lib.Objs.Property uniqueName = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "uniqueName");
public net.java.html.lib.knockout.KnockoutBindingHandler uniqueName() { return uniqueName.get(); }
// Rendering templates
public net.java.html.lib.Objs.Property template = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "template");
public net.java.html.lib.knockout.KnockoutBindingHandler template() { return template.get(); }
// Components (new for v3.2)
public net.java.html.lib.Objs.Property component = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.knockout.KnockoutBindingHandler.class, "component");
public net.java.html.lib.knockout.KnockoutBindingHandler component() { return component.get(); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy