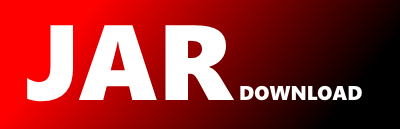
net.java.html.lib.Date Maven / Gradle / Ivy
package net.java.html.lib;
public class Date extends net.java.html.lib.Objs {
protected Date(net.java.html.lib.Objs.Constructor> c, java.lang.Object js) {
super(c, js);
}
private static final class $Constructor extends net.java.html.lib.Objs.Constructor {
$Constructor() {
super(Date.class);
}
@Override
public Date create(java.lang.Object obj) {
return obj == null ? null : new Date(this, obj);
}
@Override
public Date create(java.lang.Object obj, java.lang.reflect.Type... typeParameters) {
return obj == null ? null : new Date(this, obj);
}
};
private static final $Constructor $AS = new $Constructor();
public static Date $as(java.lang.Object obj) {
return $AS.create(obj);
}
/** Gets the day-of-the-month, using local time. */
public double getDate() {
return ((java.lang.Number)$Typings$.getDate$50($js(this))).doubleValue();
}
/** Gets the day of the week, using local time. */
public double getDay() {
return ((java.lang.Number)$Typings$.getDay$51($js(this))).doubleValue();
}
/** Gets the year, using local time. */
public double getFullYear() {
return ((java.lang.Number)$Typings$.getFullYear$52($js(this))).doubleValue();
}
/** Gets the hours in a date, using local time. */
public double getHours() {
return ((java.lang.Number)$Typings$.getHours$53($js(this))).doubleValue();
}
/** Gets the milliseconds of a Date, using local time. */
public double getMilliseconds() {
return ((java.lang.Number)$Typings$.getMilliseconds$54($js(this))).doubleValue();
}
/** Gets the minutes of a Date object, using local time. */
public double getMinutes() {
return ((java.lang.Number)$Typings$.getMinutes$55($js(this))).doubleValue();
}
/** Gets the month, using local time. */
public double getMonth() {
return ((java.lang.Number)$Typings$.getMonth$56($js(this))).doubleValue();
}
/** Gets the seconds of a Date object, using local time. */
public double getSeconds() {
return ((java.lang.Number)$Typings$.getSeconds$57($js(this))).doubleValue();
}
/** Gets the time value in milliseconds. */
public double getTime() {
return ((java.lang.Number)$Typings$.getTime$58($js(this))).doubleValue();
}
/** Gets the difference in minutes between the time on the local computer and Universal Coordinated Time (UTC). */
public double getTimezoneOffset() {
return ((java.lang.Number)$Typings$.getTimezoneOffset$59($js(this))).doubleValue();
}
/** Gets the day-of-the-month, using Universal Coordinated Time (UTC). */
public double getUTCDate() {
return ((java.lang.Number)$Typings$.getUTCDate$60($js(this))).doubleValue();
}
/** Gets the day of the week using Universal Coordinated Time (UTC). */
public double getUTCDay() {
return ((java.lang.Number)$Typings$.getUTCDay$61($js(this))).doubleValue();
}
/** Gets the year using Universal Coordinated Time (UTC). */
public double getUTCFullYear() {
return ((java.lang.Number)$Typings$.getUTCFullYear$62($js(this))).doubleValue();
}
/** Gets the hours value in a Date object using Universal Coordinated Time (UTC). */
public double getUTCHours() {
return ((java.lang.Number)$Typings$.getUTCHours$63($js(this))).doubleValue();
}
/** Gets the milliseconds of a Date object using Universal Coordinated Time (UTC). */
public double getUTCMilliseconds() {
return ((java.lang.Number)$Typings$.getUTCMilliseconds$64($js(this))).doubleValue();
}
/** Gets the minutes of a Date object using Universal Coordinated Time (UTC). */
public double getUTCMinutes() {
return ((java.lang.Number)$Typings$.getUTCMinutes$65($js(this))).doubleValue();
}
/** Gets the month of a Date object using Universal Coordinated Time (UTC). */
public double getUTCMonth() {
return ((java.lang.Number)$Typings$.getUTCMonth$66($js(this))).doubleValue();
}
/** Gets the seconds of a Date object using Universal Coordinated Time (UTC). */
public double getUTCSeconds() {
return ((java.lang.Number)$Typings$.getUTCSeconds$67($js(this))).doubleValue();
}
/**
* Sets the numeric day-of-the-month value of the Date object using local time.
* @param date A numeric value equal to the day of the month.
*/
public double setDate(double date) {
return ((java.lang.Number)$Typings$.setDate$68($js(this), date)).doubleValue();
}
/**
* Sets the year of the Date object using local time.
* @param year A numeric value for the year.
* @param month A zero-based numeric value for the month (0 for January, 11 for December). Must be specified if numDate is specified.
* @param date A numeric value equal for the day of the month.
*/
public double setFullYear(double year, double month, double date) {
return ((java.lang.Number)$Typings$.setFullYear$69($js(this), year, month, date)).doubleValue();
}
/**
* Sets the year of the Date object using local time.
* @param year A numeric value for the year.
* @param month A zero-based numeric value for the month (0 for January, 11 for December). Must be specified if numDate is specified.
* @param date A numeric value equal for the day of the month.
*/
public double setFullYear(double year) {
return ((java.lang.Number)$Typings$.setFullYear$70($js(this), year)).doubleValue();
}
/**
* Sets the year of the Date object using local time.
* @param year A numeric value for the year.
* @param month A zero-based numeric value for the month (0 for January, 11 for December). Must be specified if numDate is specified.
* @param date A numeric value equal for the day of the month.
*/
public double setFullYear(double year, double month) {
return ((java.lang.Number)$Typings$.setFullYear$71($js(this), year, month)).doubleValue();
}
/**
* Sets the hour value in the Date object using local time.
* @param hours A numeric value equal to the hours value.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setHours(double hours, double min, double sec, double ms) {
return ((java.lang.Number)$Typings$.setHours$72($js(this), hours, min, sec, ms)).doubleValue();
}
/**
* Sets the hour value in the Date object using local time.
* @param hours A numeric value equal to the hours value.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setHours(double hours) {
return ((java.lang.Number)$Typings$.setHours$73($js(this), hours)).doubleValue();
}
/**
* Sets the hour value in the Date object using local time.
* @param hours A numeric value equal to the hours value.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setHours(double hours, double min) {
return ((java.lang.Number)$Typings$.setHours$74($js(this), hours, min)).doubleValue();
}
/**
* Sets the hour value in the Date object using local time.
* @param hours A numeric value equal to the hours value.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setHours(double hours, double min, double sec) {
return ((java.lang.Number)$Typings$.setHours$75($js(this), hours, min, sec)).doubleValue();
}
/**
* Sets the milliseconds value in the Date object using local time.
* @param ms A numeric value equal to the millisecond value.
*/
public double setMilliseconds(double ms) {
return ((java.lang.Number)$Typings$.setMilliseconds$76($js(this), ms)).doubleValue();
}
/**
* Sets the minutes value in the Date object using local time.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setMinutes(double min, double sec, double ms) {
return ((java.lang.Number)$Typings$.setMinutes$77($js(this), min, sec, ms)).doubleValue();
}
/**
* Sets the minutes value in the Date object using local time.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setMinutes(double min) {
return ((java.lang.Number)$Typings$.setMinutes$78($js(this), min)).doubleValue();
}
/**
* Sets the minutes value in the Date object using local time.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setMinutes(double min, double sec) {
return ((java.lang.Number)$Typings$.setMinutes$79($js(this), min, sec)).doubleValue();
}
/**
* Sets the month value in the Date object using local time.
* @param month A numeric value equal to the month. The value for January is 0, and other month values follow consecutively.
* @param date A numeric value representing the day of the month. If this value is not supplied, the value from a call to the getDate method is used.
*/
public double setMonth(double month, double date) {
return ((java.lang.Number)$Typings$.setMonth$80($js(this), month, date)).doubleValue();
}
/**
* Sets the month value in the Date object using local time.
* @param month A numeric value equal to the month. The value for January is 0, and other month values follow consecutively.
* @param date A numeric value representing the day of the month. If this value is not supplied, the value from a call to the getDate method is used.
*/
public double setMonth(double month) {
return ((java.lang.Number)$Typings$.setMonth$81($js(this), month)).doubleValue();
}
/**
* Sets the seconds value in the Date object using local time.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setSeconds(double sec, double ms) {
return ((java.lang.Number)$Typings$.setSeconds$82($js(this), sec, ms)).doubleValue();
}
/**
* Sets the seconds value in the Date object using local time.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setSeconds(double sec) {
return ((java.lang.Number)$Typings$.setSeconds$83($js(this), sec)).doubleValue();
}
/**
* Sets the date and time value in the Date object.
* @param time A numeric value representing the number of elapsed milliseconds since midnight, January 1, 1970 GMT.
*/
public double setTime(double time) {
return ((java.lang.Number)$Typings$.setTime$84($js(this), time)).doubleValue();
}
/**
* Sets the numeric day of the month in the Date object using Universal Coordinated Time (UTC).
* @param date A numeric value equal to the day of the month.
*/
public double setUTCDate(double date) {
return ((java.lang.Number)$Typings$.setUTCDate$85($js(this), date)).doubleValue();
}
/**
* Sets the year value in the Date object using Universal Coordinated Time (UTC).
* @param year A numeric value equal to the year.
* @param month A numeric value equal to the month. The value for January is 0, and other month values follow consecutively. Must be supplied if numDate is supplied.
* @param date A numeric value equal to the day of the month.
*/
public double setUTCFullYear(double year, double month, double date) {
return ((java.lang.Number)$Typings$.setUTCFullYear$86($js(this), year, month, date)).doubleValue();
}
/**
* Sets the year value in the Date object using Universal Coordinated Time (UTC).
* @param year A numeric value equal to the year.
* @param month A numeric value equal to the month. The value for January is 0, and other month values follow consecutively. Must be supplied if numDate is supplied.
* @param date A numeric value equal to the day of the month.
*/
public double setUTCFullYear(double year) {
return ((java.lang.Number)$Typings$.setUTCFullYear$87($js(this), year)).doubleValue();
}
/**
* Sets the year value in the Date object using Universal Coordinated Time (UTC).
* @param year A numeric value equal to the year.
* @param month A numeric value equal to the month. The value for January is 0, and other month values follow consecutively. Must be supplied if numDate is supplied.
* @param date A numeric value equal to the day of the month.
*/
public double setUTCFullYear(double year, double month) {
return ((java.lang.Number)$Typings$.setUTCFullYear$88($js(this), year, month)).doubleValue();
}
/**
* Sets the hours value in the Date object using Universal Coordinated Time (UTC).
* @param hours A numeric value equal to the hours value.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCHours(double hours, double min, double sec, double ms) {
return ((java.lang.Number)$Typings$.setUTCHours$89($js(this), hours, min, sec, ms)).doubleValue();
}
/**
* Sets the hours value in the Date object using Universal Coordinated Time (UTC).
* @param hours A numeric value equal to the hours value.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCHours(double hours) {
return ((java.lang.Number)$Typings$.setUTCHours$90($js(this), hours)).doubleValue();
}
/**
* Sets the hours value in the Date object using Universal Coordinated Time (UTC).
* @param hours A numeric value equal to the hours value.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCHours(double hours, double min) {
return ((java.lang.Number)$Typings$.setUTCHours$91($js(this), hours, min)).doubleValue();
}
/**
* Sets the hours value in the Date object using Universal Coordinated Time (UTC).
* @param hours A numeric value equal to the hours value.
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCHours(double hours, double min, double sec) {
return ((java.lang.Number)$Typings$.setUTCHours$92($js(this), hours, min, sec)).doubleValue();
}
/**
* Sets the milliseconds value in the Date object using Universal Coordinated Time (UTC).
* @param ms A numeric value equal to the millisecond value.
*/
public double setUTCMilliseconds(double ms) {
return ((java.lang.Number)$Typings$.setUTCMilliseconds$93($js(this), ms)).doubleValue();
}
/**
* Sets the minutes value in the Date object using Universal Coordinated Time (UTC).
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCMinutes(double min, double sec, double ms) {
return ((java.lang.Number)$Typings$.setUTCMinutes$94($js(this), min, sec, ms)).doubleValue();
}
/**
* Sets the minutes value in the Date object using Universal Coordinated Time (UTC).
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCMinutes(double min) {
return ((java.lang.Number)$Typings$.setUTCMinutes$95($js(this), min)).doubleValue();
}
/**
* Sets the minutes value in the Date object using Universal Coordinated Time (UTC).
* @param min A numeric value equal to the minutes value.
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCMinutes(double min, double sec) {
return ((java.lang.Number)$Typings$.setUTCMinutes$96($js(this), min, sec)).doubleValue();
}
/**
* Sets the month value in the Date object using Universal Coordinated Time (UTC).
* @param month A numeric value equal to the month. The value for January is 0, and other month values follow consecutively.
* @param date A numeric value representing the day of the month. If it is not supplied, the value from a call to the getUTCDate method is used.
*/
public double setUTCMonth(double month, double date) {
return ((java.lang.Number)$Typings$.setUTCMonth$97($js(this), month, date)).doubleValue();
}
/**
* Sets the month value in the Date object using Universal Coordinated Time (UTC).
* @param month A numeric value equal to the month. The value for January is 0, and other month values follow consecutively.
* @param date A numeric value representing the day of the month. If it is not supplied, the value from a call to the getUTCDate method is used.
*/
public double setUTCMonth(double month) {
return ((java.lang.Number)$Typings$.setUTCMonth$98($js(this), month)).doubleValue();
}
/**
* Sets the seconds value in the Date object using Universal Coordinated Time (UTC).
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCSeconds(double sec, double ms) {
return ((java.lang.Number)$Typings$.setUTCSeconds$99($js(this), sec, ms)).doubleValue();
}
/**
* Sets the seconds value in the Date object using Universal Coordinated Time (UTC).
* @param sec A numeric value equal to the seconds value.
* @param ms A numeric value equal to the milliseconds value.
*/
public double setUTCSeconds(double sec) {
return ((java.lang.Number)$Typings$.setUTCSeconds$100($js(this), sec)).doubleValue();
}
/** Returns a date as a string value. */
public java.lang.String toDateString() {
return $Typings$.toDateString$101($js(this));
}
/** Returns a date as a string value in ISO format. */
public java.lang.String toISOString() {
return $Typings$.toISOString$102($js(this));
}
/** Used by the JSON.stringify method to enable the transformation of an object's data for JavaScript Object Notation (JSON) serialization. */
public java.lang.String toJSON(java.lang.Object key) {
return $Typings$.toJSON$103($js(this), /* AnyKeyword*/$js(key));
}
/** Used by the JSON.stringify method to enable the transformation of an object's data for JavaScript Object Notation (JSON) serialization. */
public java.lang.String toJSON() {
return $Typings$.toJSON$104($js(this));
}
/** Returns a date as a string value appropriate to the host environment's current locale. */
public java.lang.String toLocaleDateString() {
return $Typings$.toLocaleDateString$105($js(this));
}
/** Returns a value as a string value appropriate to the host environment's current locale. */
public java.lang.String toLocaleString() {
return $Typings$.toLocaleString$106($js(this));
}
/** Returns a time as a string value appropriate to the host environment's current locale. */
public java.lang.String toLocaleTimeString() {
return $Typings$.toLocaleTimeString$107($js(this));
}
/** Returns a string representation of a date. The format of the string depends on the locale. */
/* cannot generate toString */
/** Returns a time as a string value. */
public java.lang.String toTimeString() {
return $Typings$.toTimeString$108($js(this));
}
/** Returns a date converted to a string using Universal Coordinated Time (UTC). */
public java.lang.String toUTCString() {
return $Typings$.toUTCString$109($js(this));
}
/** Returns the stored time value in milliseconds since midnight, January 1, 1970 UTC. */
public java.lang.Number valueOf() {
return $Typings$.valueOf$110($js(this));
}
// constructor DateConstructor
public static net.java.html.lib.Date prototype = (net.java.html.lib.Date)net.java.html.lib.Date.$as($Typings$.readStaticFields$111());
/**
* Parses a string containing a date, and returns the number of milliseconds between that date and midnight, January 1, 1970.
* @param s A date string
*/
public static double parse(java.lang.String s) {
return ((java.lang.Number)$Typings$.parse$112(s)).doubleValue();
}
/**
* Returns the number of milliseconds between midnight, January 1, 1970 Universal Coordinated Time (UTC) (or GMT) and the specified date.
* @param year The full year designation is required for cross-century date accuracy. If year is between 0 and 99 is used, then year is assumed to be 1900 + year.
* @param month The month as an number between 0 and 11 (January to December).
* @param date The date as an number between 1 and 31.
* @param hours Must be supplied if minutes is supplied. An number from 0 to 23 (midnight to 11pm) that specifies the hour.
* @param minutes Must be supplied if seconds is supplied. An number from 0 to 59 that specifies the minutes.
* @param seconds Must be supplied if milliseconds is supplied. An number from 0 to 59 that specifies the seconds.
* @param ms An number from 0 to 999 that specifies the milliseconds.
*/
public static double UTC(double year, double month, double date, double hours, double minutes, double seconds, double ms) {
return ((java.lang.Number)$Typings$.UTC$113(year, month, date, hours, minutes, seconds, ms)).doubleValue();
}
/**
* Returns the number of milliseconds between midnight, January 1, 1970 Universal Coordinated Time (UTC) (or GMT) and the specified date.
* @param year The full year designation is required for cross-century date accuracy. If year is between 0 and 99 is used, then year is assumed to be 1900 + year.
* @param month The month as an number between 0 and 11 (January to December).
* @param date The date as an number between 1 and 31.
* @param hours Must be supplied if minutes is supplied. An number from 0 to 23 (midnight to 11pm) that specifies the hour.
* @param minutes Must be supplied if seconds is supplied. An number from 0 to 59 that specifies the minutes.
* @param seconds Must be supplied if milliseconds is supplied. An number from 0 to 59 that specifies the seconds.
* @param ms An number from 0 to 999 that specifies the milliseconds.
*/
public static double UTC(double year, double month) {
return ((java.lang.Number)$Typings$.UTC$114(year, month)).doubleValue();
}
/**
* Returns the number of milliseconds between midnight, January 1, 1970 Universal Coordinated Time (UTC) (or GMT) and the specified date.
* @param year The full year designation is required for cross-century date accuracy. If year is between 0 and 99 is used, then year is assumed to be 1900 + year.
* @param month The month as an number between 0 and 11 (January to December).
* @param date The date as an number between 1 and 31.
* @param hours Must be supplied if minutes is supplied. An number from 0 to 23 (midnight to 11pm) that specifies the hour.
* @param minutes Must be supplied if seconds is supplied. An number from 0 to 59 that specifies the minutes.
* @param seconds Must be supplied if milliseconds is supplied. An number from 0 to 59 that specifies the seconds.
* @param ms An number from 0 to 999 that specifies the milliseconds.
*/
public static double UTC(double year, double month, double date) {
return ((java.lang.Number)$Typings$.UTC$115(year, month, date)).doubleValue();
}
/**
* Returns the number of milliseconds between midnight, January 1, 1970 Universal Coordinated Time (UTC) (or GMT) and the specified date.
* @param year The full year designation is required for cross-century date accuracy. If year is between 0 and 99 is used, then year is assumed to be 1900 + year.
* @param month The month as an number between 0 and 11 (January to December).
* @param date The date as an number between 1 and 31.
* @param hours Must be supplied if minutes is supplied. An number from 0 to 23 (midnight to 11pm) that specifies the hour.
* @param minutes Must be supplied if seconds is supplied. An number from 0 to 59 that specifies the minutes.
* @param seconds Must be supplied if milliseconds is supplied. An number from 0 to 59 that specifies the seconds.
* @param ms An number from 0 to 999 that specifies the milliseconds.
*/
public static double UTC(double year, double month, double date, double hours) {
return ((java.lang.Number)$Typings$.UTC$116(year, month, date, hours)).doubleValue();
}
/**
* Returns the number of milliseconds between midnight, January 1, 1970 Universal Coordinated Time (UTC) (or GMT) and the specified date.
* @param year The full year designation is required for cross-century date accuracy. If year is between 0 and 99 is used, then year is assumed to be 1900 + year.
* @param month The month as an number between 0 and 11 (January to December).
* @param date The date as an number between 1 and 31.
* @param hours Must be supplied if minutes is supplied. An number from 0 to 23 (midnight to 11pm) that specifies the hour.
* @param minutes Must be supplied if seconds is supplied. An number from 0 to 59 that specifies the minutes.
* @param seconds Must be supplied if milliseconds is supplied. An number from 0 to 59 that specifies the seconds.
* @param ms An number from 0 to 999 that specifies the milliseconds.
*/
public static double UTC(double year, double month, double date, double hours, double minutes) {
return ((java.lang.Number)$Typings$.UTC$117(year, month, date, hours, minutes)).doubleValue();
}
/**
* Returns the number of milliseconds between midnight, January 1, 1970 Universal Coordinated Time (UTC) (or GMT) and the specified date.
* @param year The full year designation is required for cross-century date accuracy. If year is between 0 and 99 is used, then year is assumed to be 1900 + year.
* @param month The month as an number between 0 and 11 (January to December).
* @param date The date as an number between 1 and 31.
* @param hours Must be supplied if minutes is supplied. An number from 0 to 23 (midnight to 11pm) that specifies the hour.
* @param minutes Must be supplied if seconds is supplied. An number from 0 to 59 that specifies the minutes.
* @param seconds Must be supplied if milliseconds is supplied. An number from 0 to 59 that specifies the seconds.
* @param ms An number from 0 to 999 that specifies the milliseconds.
*/
public static double UTC(double year, double month, double date, double hours, double minutes, double seconds) {
return ((java.lang.Number)$Typings$.UTC$118(year, month, date, hours, minutes, seconds)).doubleValue();
}
public static double now() {
return ((java.lang.Number)$Typings$.now$119()).doubleValue();
}
public Date() {
this(Date.$AS, $Typings$.new$120());
}
public Date(double value) {
this(Date.$AS, $Typings$.new$121(value));
}
public Date(double year, double month, double date, double hours, double minutes, double seconds, double ms) {
this(Date.$AS, $Typings$.new$122(year, month, date, hours, minutes, seconds, ms));
}
public Date(double year, double month) {
this(Date.$AS, $Typings$.new$123(year, month));
}
public Date(double year, double month, double date) {
this(Date.$AS, $Typings$.new$124(year, month, date));
}
public Date(double year, double month, double date, double hours) {
this(Date.$AS, $Typings$.new$125(year, month, date, hours));
}
public Date(double year, double month, double date, double hours, double minutes) {
this(Date.$AS, $Typings$.new$126(year, month, date, hours, minutes));
}
public Date(double year, double month, double date, double hours, double minutes, double seconds) {
this(Date.$AS, $Typings$.new$127(year, month, date, hours, minutes, seconds));
}
public Date(java.lang.String value) {
this(Date.$AS, $Typings$.new$128(value));
}
public static java.lang.String newDate() {
return $Typings$.newDate$129();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy