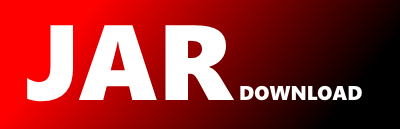
net.java.html.lib.Float64Array Maven / Gradle / Ivy
The newest version!
package net.java.html.lib;
public class Float64Array extends net.java.html.lib.Objs {
protected Float64Array(net.java.html.lib.Objs.Constructor> c, java.lang.Object js) {
super(c, js);
}
private static final class $Constructor extends net.java.html.lib.Objs.Constructor {
$Constructor() {
super(Float64Array.class);
}
@Override
public Float64Array create(java.lang.Object obj) {
return obj == null ? null : new Float64Array(this, obj);
}
@Override
public Float64Array create(java.lang.Object obj, java.lang.reflect.Type... typeParameters) {
return obj == null ? null : new Float64Array(this, obj);
}
};
private static final $Constructor $AS = new $Constructor();
public static Float64Array $as(java.lang.Object obj) {
return $AS.create(obj);
}
/**
* The size in bytes of each element in the array.
*/
public net.java.html.lib.Objs.Property BYTES_PER_ELEMENT = net.java.html.lib.Objs.Property.create(this, java.lang.Number.class, "BYTES_PER_ELEMENT");
public java.lang.Number BYTES_PER_ELEMENT() { return BYTES_PER_ELEMENT.get(); }
/**
* The ArrayBuffer instance referenced by the array.
*/
public net.java.html.lib.Objs.Property buffer = net.java.html.lib.Objs.Property.create(this, net.java.html.lib.ArrayBuffer.class, "buffer");
public net.java.html.lib.ArrayBuffer buffer() { return buffer.get(); }
/**
* The length in bytes of the array.
*/
public net.java.html.lib.Objs.Property byteLength = net.java.html.lib.Objs.Property.create(this, java.lang.Number.class, "byteLength");
public java.lang.Number byteLength() { return byteLength.get(); }
/**
* The offset in bytes of the array.
*/
public net.java.html.lib.Objs.Property byteOffset = net.java.html.lib.Objs.Property.create(this, java.lang.Number.class, "byteOffset");
public java.lang.Number byteOffset() { return byteOffset.get(); }
/**
* The length of the array.
*/
public net.java.html.lib.Objs.Property length = net.java.html.lib.Objs.Property.create(this, java.lang.Number.class, "length");
public java.lang.Number length() { return length.get(); }
public double $get(double index) {
return ((java.lang.Number)$Typings$.$get$189($js(this), index)).doubleValue();
}
/**
* Returns the this object after copying a section of the array identified by start and end
* to the same array starting at position target
* @param target If target is negative, it is treated as length+target where length is the
* length of the array.
* @param start If start is negative, it is treated as length+start. If end is negative, it
* is treated as length+end.
* @param end If not specified, length of the this object is used as its default value.
*/
public net.java.html.lib.Float64Array copyWithin(double target, double start, double end) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.copyWithin$190($js(this), target, start, end));
}
/**
* Returns the this object after copying a section of the array identified by start and end
* to the same array starting at position target
* @param target If target is negative, it is treated as length+target where length is the
* length of the array.
* @param start If start is negative, it is treated as length+start. If end is negative, it
* is treated as length+end.
* @param end If not specified, length of the this object is used as its default value.
*/
public net.java.html.lib.Float64Array copyWithin(double target, double start) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.copyWithin$191($js(this), target, start));
}
/**
* Determines whether all the members of an array satisfy the specified test.
* @param callbackfn A function that accepts up to three arguments. The every method calls
* the callbackfn function for each element in array1 until the callbackfn returns false,
* or until the end of the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public java.lang.Boolean every(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Boolean> callbackfn, java.lang.Object thisArg) {
return $Typings$.every$192($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), /* AnyKeyword*/$js(thisArg));
}
/**
* Determines whether all the members of an array satisfy the specified test.
* @param callbackfn A function that accepts up to three arguments. The every method calls
* the callbackfn function for each element in array1 until the callbackfn returns false,
* or until the end of the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public java.lang.Boolean every(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Boolean> callbackfn) {
return $Typings$.every$193($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})));
}
/**
* Returns the this object after filling the section identified by start and end with value
* @param value value to fill array section with
* @param start index to start filling the array at. If start is negative, it is treated as
* length+start where length is the length of the array.
* @param end index to stop filling the array at. If end is negative, it is treated as
* length+end.
*/
public net.java.html.lib.Float64Array fill(double value, double start, double end) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.fill$194($js(this), value, start, end));
}
/**
* Returns the this object after filling the section identified by start and end with value
* @param value value to fill array section with
* @param start index to start filling the array at. If start is negative, it is treated as
* length+start where length is the length of the array.
* @param end index to stop filling the array at. If end is negative, it is treated as
* length+end.
*/
public net.java.html.lib.Float64Array fill(double value) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.fill$195($js(this), value));
}
/**
* Returns the this object after filling the section identified by start and end with value
* @param value value to fill array section with
* @param start index to start filling the array at. If start is negative, it is treated as
* length+start where length is the length of the array.
* @param end index to stop filling the array at. If end is negative, it is treated as
* length+end.
*/
public net.java.html.lib.Float64Array fill(double value, double start) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.fill$196($js(this), value, start));
}
/**
* Returns the elements of an array that meet the condition specified in a callback function.
* @param callbackfn A function that accepts up to three arguments. The filter method calls
* the callbackfn function one time for each element in the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public net.java.html.lib.Float64Array filter(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Boolean> callbackfn, java.lang.Object thisArg) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.filter$197($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), /* AnyKeyword*/$js(thisArg)));
}
/**
* Returns the elements of an array that meet the condition specified in a callback function.
* @param callbackfn A function that accepts up to three arguments. The filter method calls
* the callbackfn function one time for each element in the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public net.java.html.lib.Float64Array filter(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Boolean> callbackfn) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.filter$198($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class}))));
}
/**
* Returns the value of the first element in the array where predicate is true, and undefined
* otherwise.
* @param predicate find calls predicate once for each element of the array, in ascending
* order, until it finds one where predicate returns true. If such an element is found, find
* immediately returns that element value. Otherwise, find returns undefined.
* @param thisArg If provided, it will be used as the this value for each invocation of
* predicate. If it is not provided, undefined is used instead.
*/
public double find(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Array,? extends java.lang.Boolean> predicate, java.lang.Object thisArg) {
return ((java.lang.Number)$Typings$.find$199($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(predicate, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Array.class})), /* AnyKeyword*/$js(thisArg))).doubleValue();
}
/**
* Returns the value of the first element in the array where predicate is true, and undefined
* otherwise.
* @param predicate find calls predicate once for each element of the array, in ascending
* order, until it finds one where predicate returns true. If such an element is found, find
* immediately returns that element value. Otherwise, find returns undefined.
* @param thisArg If provided, it will be used as the this value for each invocation of
* predicate. If it is not provided, undefined is used instead.
*/
public double find(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Array,? extends java.lang.Boolean> predicate) {
return ((java.lang.Number)$Typings$.find$200($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(predicate, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Array.class})))).doubleValue();
}
/**
* Returns the index of the first element in the array where predicate is true, and undefined
* otherwise.
* @param predicate find calls predicate once for each element of the array, in ascending
* order, until it finds one where predicate returns true. If such an element is found, find
* immediately returns that element value. Otherwise, find returns undefined.
* @param thisArg If provided, it will be used as the this value for each invocation of
* predicate. If it is not provided, undefined is used instead.
*/
public double findIndex(net.java.html.lib.Function.A1 super java.lang.Number,? extends java.lang.Boolean> predicate, java.lang.Object thisArg) {
return ((java.lang.Number)$Typings$.findIndex$201($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(predicate, new Class[] {java.lang.Number.class})), /* AnyKeyword*/$js(thisArg))).doubleValue();
}
/**
* Returns the index of the first element in the array where predicate is true, and undefined
* otherwise.
* @param predicate find calls predicate once for each element of the array, in ascending
* order, until it finds one where predicate returns true. If such an element is found, find
* immediately returns that element value. Otherwise, find returns undefined.
* @param thisArg If provided, it will be used as the this value for each invocation of
* predicate. If it is not provided, undefined is used instead.
*/
public double findIndex(net.java.html.lib.Function.A1 super java.lang.Number,? extends java.lang.Boolean> predicate) {
return ((java.lang.Number)$Typings$.findIndex$202($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(predicate, new Class[] {java.lang.Number.class})))).doubleValue();
}
/**
* Performs the specified action for each element in an array.
* @param callbackfn A function that accepts up to three arguments. forEach calls the
* callbackfn function one time for each element in the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public void forEach(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Void> callbackfn, java.lang.Object thisArg) {
$Typings$.forEach$203($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), /* AnyKeyword*/$js(thisArg));
}
/**
* Performs the specified action for each element in an array.
* @param callbackfn A function that accepts up to three arguments. forEach calls the
* callbackfn function one time for each element in the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public void forEach(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Void> callbackfn) {
$Typings$.forEach$204($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})));
}
/**
* Returns the index of the first occurrence of a value in an array.
* @param searchElement The value to locate in the array.
* @param fromIndex The array index at which to begin the search. If fromIndex is omitted, the
* search starts at index 0.
*/
public double indexOf(double searchElement, double fromIndex) {
return ((java.lang.Number)$Typings$.indexOf$205($js(this), searchElement, fromIndex)).doubleValue();
}
/**
* Returns the index of the first occurrence of a value in an array.
* @param searchElement The value to locate in the array.
* @param fromIndex The array index at which to begin the search. If fromIndex is omitted, the
* search starts at index 0.
*/
public double indexOf(double searchElement) {
return ((java.lang.Number)$Typings$.indexOf$206($js(this), searchElement)).doubleValue();
}
/**
* Adds all the elements of an array separated by the specified separator string.
* @param separator A string used to separate one element of an array from the next in the
* resulting String. If omitted, the array elements are separated with a comma.
*/
public java.lang.String join(java.lang.String separator) {
return $Typings$.join$207($js(this), separator);
}
/**
* Adds all the elements of an array separated by the specified separator string.
* @param separator A string used to separate one element of an array from the next in the
* resulting String. If omitted, the array elements are separated with a comma.
*/
public java.lang.String join() {
return $Typings$.join$208($js(this));
}
/**
* Returns the index of the last occurrence of a value in an array.
* @param searchElement The value to locate in the array.
* @param fromIndex The array index at which to begin the search. If fromIndex is omitted, the
* search starts at index 0.
*/
public double lastIndexOf(double searchElement, double fromIndex) {
return ((java.lang.Number)$Typings$.lastIndexOf$209($js(this), searchElement, fromIndex)).doubleValue();
}
/**
* Returns the index of the last occurrence of a value in an array.
* @param searchElement The value to locate in the array.
* @param fromIndex The array index at which to begin the search. If fromIndex is omitted, the
* search starts at index 0.
*/
public double lastIndexOf(double searchElement) {
return ((java.lang.Number)$Typings$.lastIndexOf$210($js(this), searchElement)).doubleValue();
}
/**
* Calls a defined callback function on each element of an array, and returns an array that
* contains the results.
* @param callbackfn A function that accepts up to three arguments. The map method calls the
* callbackfn function one time for each element in the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public net.java.html.lib.Float64Array map(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Number> callbackfn, java.lang.Object thisArg) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.map$211($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), /* AnyKeyword*/$js(thisArg)));
}
/**
* Calls a defined callback function on each element of an array, and returns an array that
* contains the results.
* @param callbackfn A function that accepts up to three arguments. The map method calls the
* callbackfn function one time for each element in the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public net.java.html.lib.Float64Array map(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Number> callbackfn) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.map$212($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class}))));
}
/**
* Calls the specified callback function for all the elements in an array. The return value of
* the callback function is the accumulated result, and is provided as an argument in the next
* call to the callback function.
* @param callbackfn A function that accepts up to four arguments. The reduce method calls the
* callbackfn function one time for each element in the array.
* @param initialValue If initialValue is specified, it is used as the initial value to start
* the accumulation. The first call to the callbackfn function provides this value as an argument
* instead of an array value.
*/
public double reduce(net.java.html.lib.Function.A4 super java.lang.Number,? super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Number> callbackfn, double initialValue) {
return ((java.lang.Number)$Typings$.reduce$213($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), initialValue)).doubleValue();
}
/**
* Calls the specified callback function for all the elements in an array. The return value of
* the callback function is the accumulated result, and is provided as an argument in the next
* call to the callback function.
* @param callbackfn A function that accepts up to four arguments. The reduce method calls the
* callbackfn function one time for each element in the array.
* @param initialValue If initialValue is specified, it is used as the initial value to start
* the accumulation. The first call to the callbackfn function provides this value as an argument
* instead of an array value.
*/
public double reduce(net.java.html.lib.Function.A4 super java.lang.Number,? super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Number> callbackfn) {
return ((java.lang.Number)$Typings$.reduce$214($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})))).doubleValue();
}
/**
* Calls the specified callback function for all the elements in an array. The return value of
* the callback function is the accumulated result, and is provided as an argument in the next
* call to the callback function.
* @param callbackfn A function that accepts up to four arguments. The reduce method calls the
* callbackfn function one time for each element in the array.
* @param initialValue If initialValue is specified, it is used as the initial value to start
* the accumulation. The first call to the callbackfn function provides this value as an argument
* instead of an array value.
*/
public U reduce(net.java.html.lib.Function.A4 super U,? super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends U> callbackfn, U initialValue) {
return (U)$Typings$.reduce$215($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {null, java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), /* FirstTypeNode*/$js(initialValue));
}
/**
* Calls the specified callback function for all the elements in an array, in descending order.
* The return value of the callback function is the accumulated result, and is provided as an
* argument in the next call to the callback function.
* @param callbackfn A function that accepts up to four arguments. The reduceRight method calls
* the callbackfn function one time for each element in the array.
* @param initialValue If initialValue is specified, it is used as the initial value to start
* the accumulation. The first call to the callbackfn function provides this value as an
* argument instead of an array value.
*/
public double reduceRight(net.java.html.lib.Function.A4 super java.lang.Number,? super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Number> callbackfn, double initialValue) {
return ((java.lang.Number)$Typings$.reduceRight$216($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), initialValue)).doubleValue();
}
/**
* Calls the specified callback function for all the elements in an array, in descending order.
* The return value of the callback function is the accumulated result, and is provided as an
* argument in the next call to the callback function.
* @param callbackfn A function that accepts up to four arguments. The reduceRight method calls
* the callbackfn function one time for each element in the array.
* @param initialValue If initialValue is specified, it is used as the initial value to start
* the accumulation. The first call to the callbackfn function provides this value as an
* argument instead of an array value.
*/
public double reduceRight(net.java.html.lib.Function.A4 super java.lang.Number,? super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Number> callbackfn) {
return ((java.lang.Number)$Typings$.reduceRight$217($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})))).doubleValue();
}
/**
* Calls the specified callback function for all the elements in an array, in descending order.
* The return value of the callback function is the accumulated result, and is provided as an
* argument in the next call to the callback function.
* @param callbackfn A function that accepts up to four arguments. The reduceRight method calls
* the callbackfn function one time for each element in the array.
* @param initialValue If initialValue is specified, it is used as the initial value to start
* the accumulation. The first call to the callbackfn function provides this value as an argument
* instead of an array value.
*/
public U reduceRight(net.java.html.lib.Function.A4 super U,? super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends U> callbackfn, U initialValue) {
return (U)$Typings$.reduceRight$218($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {null, java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), /* FirstTypeNode*/$js(initialValue));
}
/**
* Reverses the elements in an Array.
*/
public net.java.html.lib.Float64Array reverse() {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.reverse$219($js(this)));
}
/**
* Sets a value or an array of values.
* @param index The index of the location to set.
* @param value The value to set.
*/
public void set(double index, double value) {
$Typings$.set$220($js(this), index, value);
}
/**
* Sets a value or an array of values.
* @param array A typed or untyped array of values to set.
* @param offset The index in the current array at which the values are to be written.
*/
public void set(net.java.html.lib.ArrayLike array, double offset) {
$Typings$.set$221($js(this), /* FirstTypeNode*/$js(array), offset);
}
/**
* Sets a value or an array of values.
* @param array A typed or untyped array of values to set.
* @param offset The index in the current array at which the values are to be written.
*/
public void set(net.java.html.lib.ArrayLike array) {
$Typings$.set$222($js(this), /* FirstTypeNode*/$js(array));
}
/**
* Returns a section of an array.
* @param start The beginning of the specified portion of the array.
* @param end The end of the specified portion of the array.
*/
public net.java.html.lib.Float64Array slice(double start, double end) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.slice$223($js(this), start, end));
}
/**
* Returns a section of an array.
* @param start The beginning of the specified portion of the array.
* @param end The end of the specified portion of the array.
*/
public net.java.html.lib.Float64Array slice() {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.slice$224($js(this)));
}
/**
* Returns a section of an array.
* @param start The beginning of the specified portion of the array.
* @param end The end of the specified portion of the array.
*/
public net.java.html.lib.Float64Array slice(double start) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.slice$225($js(this), start));
}
/**
* Determines whether the specified callback function returns true for any element of an array.
* @param callbackfn A function that accepts up to three arguments. The some method calls the
* callbackfn function for each element in array1 until the callbackfn returns true, or until
* the end of the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public java.lang.Boolean some(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Boolean> callbackfn, java.lang.Object thisArg) {
return $Typings$.some$226($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})), /* AnyKeyword*/$js(thisArg));
}
/**
* Determines whether the specified callback function returns true for any element of an array.
* @param callbackfn A function that accepts up to three arguments. The some method calls the
* callbackfn function for each element in array1 until the callbackfn returns true, or until
* the end of the array.
* @param thisArg An object to which the this keyword can refer in the callbackfn function.
* If thisArg is omitted, undefined is used as the this value.
*/
public java.lang.Boolean some(net.java.html.lib.Function.A3 super java.lang.Number,? super java.lang.Number,? super net.java.html.lib.Float64Array,? extends java.lang.Boolean> callbackfn) {
return $Typings$.some$227($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(callbackfn, new Class[] {java.lang.Number.class, java.lang.Number.class, net.java.html.lib.Float64Array.class})));
}
/**
* Sorts an array.
* @param compareFn The name of the function used to determine the order of the elements. If
* omitted, the elements are sorted in ascending, ASCII character order.
*/
public net.java.html.lib.Float64Array sort(net.java.html.lib.Function.A2 super java.lang.Number,? super java.lang.Number,? extends java.lang.Number> compareFn) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.sort$228($js(this), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(compareFn, new Class[] {java.lang.Number.class, java.lang.Number.class}))));
}
/**
* Sorts an array.
* @param compareFn The name of the function used to determine the order of the elements. If
* omitted, the elements are sorted in ascending, ASCII character order.
*/
public net.java.html.lib.Float64Array sort() {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.sort$229($js(this)));
}
/**
* Gets a new Float64Array view of the ArrayBuffer store for this array, referencing the elements
* at begin, inclusive, up to end, exclusive.
* @param begin The index of the beginning of the array.
* @param end The index of the end of the array.
*/
public net.java.html.lib.Float64Array subarray(double begin, double end) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.subarray$230($js(this), begin, end));
}
/**
* Gets a new Float64Array view of the ArrayBuffer store for this array, referencing the elements
* at begin, inclusive, up to end, exclusive.
* @param begin The index of the beginning of the array.
* @param end The index of the end of the array.
*/
public net.java.html.lib.Float64Array subarray(double begin) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.subarray$231($js(this), begin));
}
/**
* Converts a number to a string by using the current locale.
*/
public java.lang.String toLocaleString() {
return $Typings$.toLocaleString$232($js(this));
}
/**
* Returns a string representation of an array.
*/
/* cannot generate toString */
// constructor Float64ArrayConstructor
public static net.java.html.lib.Float64Array prototype = (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.readStaticFields$233());
/**
* Returns a new array from a set of elements.
* @param items A set of elements to include in the new array object.
*/
public static net.java.html.lib.Float64Array of(double... items) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.of$234(/* ArrayType*/$js(items)));
}
/**
* Creates an array from an array-like or iterable object.
* @param arrayLike An array-like or iterable object to convert to an array.
* @param mapfn A mapping function to call on every element of the array.
* @param thisArg Value of 'this' used to invoke the mapfn.
*/
public static net.java.html.lib.Float64Array from(net.java.html.lib.ArrayLike arrayLike, net.java.html.lib.Function.A2 super java.lang.Number,? super java.lang.Number,? extends java.lang.Number> mapfn, java.lang.Object thisArg) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.from$235(/* FirstTypeNode*/$js(arrayLike), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(mapfn, new Class[] {java.lang.Number.class, java.lang.Number.class})), /* AnyKeyword*/$js(thisArg)));
}
/**
* Creates an array from an array-like or iterable object.
* @param arrayLike An array-like or iterable object to convert to an array.
* @param mapfn A mapping function to call on every element of the array.
* @param thisArg Value of 'this' used to invoke the mapfn.
*/
public static net.java.html.lib.Float64Array from(net.java.html.lib.ArrayLike arrayLike) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.from$236(/* FirstTypeNode*/$js(arrayLike)));
}
/**
* Creates an array from an array-like or iterable object.
* @param arrayLike An array-like or iterable object to convert to an array.
* @param mapfn A mapping function to call on every element of the array.
* @param thisArg Value of 'this' used to invoke the mapfn.
*/
public static net.java.html.lib.Float64Array from(net.java.html.lib.ArrayLike arrayLike, net.java.html.lib.Function.A2 super java.lang.Number,? super java.lang.Number,? extends java.lang.Number> mapfn) {
return (net.java.html.lib.Float64Array)net.java.html.lib.Float64Array.$as($Typings$.from$237(/* FirstTypeNode*/$js(arrayLike), net.java.html.lib.Objs.$js(net.java.html.lib.Function.newFunction(mapfn, new Class[] {java.lang.Number.class, java.lang.Number.class}))));
}
public Float64Array(double length) {
this(Float64Array.$AS, $Typings$.new$238(length));
}
public Float64Array(net.java.html.lib.ArrayBuffer buffer, double byteOffset, double length) {
this(Float64Array.$AS, $Typings$.new$239(/* FirstTypeNode*/$js(buffer), byteOffset, length));
}
public Float64Array(net.java.html.lib.ArrayBuffer buffer) {
this(Float64Array.$AS, $Typings$.new$240(/* FirstTypeNode*/$js(buffer)));
}
public Float64Array(net.java.html.lib.ArrayBuffer buffer, double byteOffset) {
this(Float64Array.$AS, $Typings$.new$241(/* FirstTypeNode*/$js(buffer), byteOffset));
}
public Float64Array(net.java.html.lib.ArrayLike array) {
this(Float64Array.$AS, $Typings$.new$242(/* FirstTypeNode*/$js(array)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy