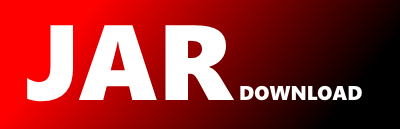
net.java.html.lib.Math Maven / Gradle / Ivy
The newest version!
package net.java.html.lib;
public class Math extends net.java.html.lib.Objs {
protected Math(net.java.html.lib.Objs.Constructor> c, java.lang.Object js) {
super(c, js);
}
private static final class $Constructor extends net.java.html.lib.Objs.Constructor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy