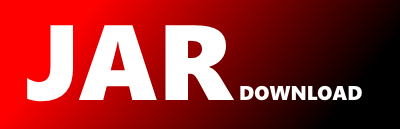
net.java.html.lib.RegExp Maven / Gradle / Ivy
package net.java.html.lib;
public class RegExp extends net.java.html.lib.Objs {
protected RegExp(net.java.html.lib.Objs.Constructor> c, java.lang.Object js) {
super(c, js);
}
private static final class $Constructor extends net.java.html.lib.Objs.Constructor {
$Constructor() {
super(RegExp.class);
}
@Override
public RegExp create(java.lang.Object obj) {
return obj == null ? null : new RegExp(this, obj);
}
@Override
public RegExp create(java.lang.Object obj, java.lang.reflect.Type... typeParameters) {
return obj == null ? null : new RegExp(this, obj);
}
};
private static final $Constructor $AS = new $Constructor();
public static RegExp $as(java.lang.Object obj) {
return $AS.create(obj);
}
/** Returns a copy of the text of the regular expression pattern. Read-only. The regExp argument is a Regular expression object. It can be a variable name or a literal. */
public net.java.html.lib.Objs.Property source = net.java.html.lib.Objs.Property.create(this, java.lang.String.class, "source");
public java.lang.String source() { return source.get(); }
/** Returns a Boolean value indicating the state of the global flag (g) used with a regular expression. Default is false. Read-only. */
public net.java.html.lib.Objs.Property global = net.java.html.lib.Objs.Property.create(this, java.lang.Boolean.class, "global");
public java.lang.Boolean global() { return global.get(); }
/** Returns a Boolean value indicating the state of the ignoreCase flag (i) used with a regular expression. Default is false. Read-only. */
public net.java.html.lib.Objs.Property ignoreCase = net.java.html.lib.Objs.Property.create(this, java.lang.Boolean.class, "ignoreCase");
public java.lang.Boolean ignoreCase() { return ignoreCase.get(); }
/** Returns a Boolean value indicating the state of the multiline flag (m) used with a regular expression. Default is false. Read-only. */
public net.java.html.lib.Objs.Property multiline = net.java.html.lib.Objs.Property.create(this, java.lang.Boolean.class, "multiline");
public java.lang.Boolean multiline() { return multiline.get(); }
public net.java.html.lib.Objs.Property lastIndex = net.java.html.lib.Objs.Property.create(this, java.lang.Number.class, "lastIndex");
public java.lang.Number lastIndex() { return lastIndex.get(); }
// Non-standard extensions
public net.java.html.lib.RegExp compile() {
return (net.java.html.lib.RegExp)net.java.html.lib.RegExp.$as($Typings$.compile$422($js(this)));
}
/**
* Executes a search on a string using a regular expression pattern, and returns an array containing the results of that search.
* @param string The String object or string literal on which to perform the search.
*/
public net.java.html.lib.RegExpExecArray exec(java.lang.String string) {
return (net.java.html.lib.RegExpExecArray)net.java.html.lib.RegExpExecArray.$as($Typings$.exec$423($js(this), string));
}
/**
* Returns a Boolean value that indicates whether or not a pattern exists in a searched string.
* @param string String on which to perform the search.
*/
public java.lang.Boolean test(java.lang.String string) {
return $Typings$.test$424($js(this), string);
}
// constructor RegExpConstructor
public static net.java.html.lib.RegExp prototype = (net.java.html.lib.RegExp)net.java.html.lib.RegExp.$as($Typings$.readStaticFields$425());
// Non-standard extensions
public static java.lang.String $1 = (java.lang.String)$Typings$.readStaticFields$426();
public static java.lang.String $2 = (java.lang.String)$Typings$.readStaticFields$427();
public static java.lang.String $3 = (java.lang.String)$Typings$.readStaticFields$428();
public static java.lang.String $4 = (java.lang.String)$Typings$.readStaticFields$429();
public static java.lang.String $5 = (java.lang.String)$Typings$.readStaticFields$430();
public static java.lang.String $6 = (java.lang.String)$Typings$.readStaticFields$431();
public static java.lang.String $7 = (java.lang.String)$Typings$.readStaticFields$432();
public static java.lang.String $8 = (java.lang.String)$Typings$.readStaticFields$433();
public static java.lang.String $9 = (java.lang.String)$Typings$.readStaticFields$434();
public static java.lang.String lastMatch = (java.lang.String)$Typings$.readStaticFields$435();
public RegExp(java.lang.String pattern, java.lang.String flags) {
this(RegExp.$AS, $Typings$.new$436(pattern, flags));
}
public RegExp(java.lang.String pattern) {
this(RegExp.$AS, $Typings$.new$437(pattern));
}
public static net.java.html.lib.RegExp newRegExp(java.lang.String pattern, java.lang.String flags) {
return (net.java.html.lib.RegExp)net.java.html.lib.RegExp.$as($Typings$.newRegExp$438(pattern, flags));
}
public static net.java.html.lib.RegExp newRegExp(java.lang.String pattern) {
return (net.java.html.lib.RegExp)net.java.html.lib.RegExp.$as($Typings$.newRegExp$439(pattern));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy