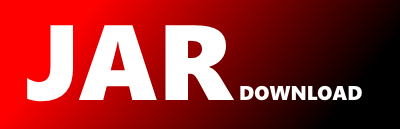
org.ibatis.persist.EntityManager Maven / Gradle / Ivy
Show all versions of jbatis Show documentation
package org.ibatis.persist;
import java.sql.SQLException;
import java.util.List;
import org.ibatis.persist.criteria.CriteriaBuilder;
import org.ibatis.persist.criteria.CriteriaQuery;
import org.ibatis.persist.criteria.CriteriaUpdate;
import org.ibatis.persist.meta.EntityType;
import org.ibatis.persist.criteria.CriteriaDelete;
/**
* EntityManager
*
* Date: 2014-10-23,13:05:21 +0800
*
* @see CriteriaQuery
* @author Song Sun
* @version 1.0
*
* @since iBatis Persistence 1.0
*/
public interface EntityManager {
/**
* Init the entity class
*
* @param entityClass
* the entity class object with annotation {@link Entity}
*
* @throws IllegalArgumentException
*/
public EntityType initEntityClass(Class entityClass);
/**
* Insert an entity object and return the entity object maybe filled with its key.
*
* @param cls
* the entity class object with annotation {@link Entity}
* @param entity
* the entity object
* @return the entity itself.
* @throws SQLException
*/
E insertEntity(Class cls, E entity) throws SQLException;
/**
* Update an entity object with primary keys.
*
* @param cls
* the entity class object with annotation {@link Entity}
* @param entity
* the entity object
* @param key
* the key of the entity.
* @return the count of rows updated.
* @throws SQLException
*/
int updateEntity(Class cls, E entity) throws SQLException;
/**
* Delete an entity by primary keys.
*
* @param cls
* the entity class object with annotation {@link Entity}
* @param key
* the keys of the entity.
* @return the count of rows deleted.
* @throws SQLException
*/
int deleteEntity(Class cls, K key) throws SQLException;
/**
* Find an entity object by primary keys.
*
* @param cls
* the entity class object with annotation {@link Entity}
* @param keys
* the keys of the entity.
* @return the count of rows updated.
* @throws SQLException
*/
E findEntity(Class cls, K key) throws SQLException;
/**
* Query the first object by the CriteriaQuery object.
*
* @param criteriaQuery
* the CriteriaQuery object.
* @return the first result object or null.
*/
public T executeQueryObject(CriteriaQuery criteriaQuery);
/**
* Query the object list by the CriteriaQuery object.
*
* @param criteriaQuery
* the CriteriaQuery object.
* @return the object list or empty list.
*/
public List executeQuery(CriteriaQuery criteriaQuery);
/**
* Query the object list by the CriteriaQuery object.
*
* @param criteriaQuery
* the CriteriaQuery object.
* @param startPosition
* position of the first result, numbered from 0
* @param maxResult
* maximum number of results to retrieve
* @return the object list or empty list.
*/
public List executeQuery(CriteriaQuery criteriaQuery, int startPosition, int maxResult);
/**
* Query the object list by the CriteriaQuery object and fill into page
*
* @param criteriaQuery
* the CriteriaQuery object.
* @param page
* the page container
* @param startPosition
* position of the first result, numbered from 0
* @param maxResult
* maximum number of results to retrieve
* @return the total rows
*/
public int executeQueryPage(CriteriaQuery criteriaQuery, List page, int startPosition, int maxResult);
/**
* Update entities by the CriteriaUpdate object.
*
* @param updateQuery
* the CriteriaUpdate object.
* @return the count of rows updated.
*/
public int executeUpdate(CriteriaUpdate updateQuery);
/**
* Delete entities by the CriteriaUpdate object.
*
* @param deleteQuery
* the CriteriaDelete object.
* @return the count of rows deleted.
*/
public int executeDelete(CriteriaDelete deleteQuery);
/**
* Return an instance of CriteriaBuilder
for the creation of CriteriaQuery
,
* CriteriaUpdate
or CriteriaDelete
objects.
*
* @return CriteriaBuilder instance
*/
public CriteriaBuilder getCriteriaBuilder();
}