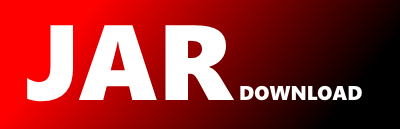
org.ibatis.persist.impl.CriteriaSubqueryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbatis Show documentation
Show all versions of jbatis Show documentation
The jBATIS persistence framework will help you to significantly reduce the amount of Java code that you normally need to access a relational database. iBATIS simply maps JavaBeans to SQL statements using a very simple XML descriptor.
The newest version!
package org.ibatis.persist.impl;
import java.util.List;
import java.util.Set;
import org.ibatis.persist.criteria.AbstractQuery;
import org.ibatis.persist.criteria.CommonAbstractCriteria;
import org.ibatis.persist.criteria.Expression;
import org.ibatis.persist.criteria.Predicate;
import org.ibatis.persist.criteria.Root;
import org.ibatis.persist.criteria.Subquery;
import org.ibatis.persist.impl.expression.DelegatedExpressionImpl;
import org.ibatis.persist.impl.expression.ExpressionImpl;
import org.ibatis.persist.meta.EntityType;
/**
* The Hibernate implementation of the JPA {@link Subquery} contract. Mostlty a set of delegation to its internal
* {@link QueryStructure}.
*/
@SuppressWarnings("unchecked")
public class CriteriaSubqueryImpl extends ExpressionImpl implements Subquery {
private final CommonAbstractCriteria parent;
private final QueryStructure queryStructure;
public CriteriaSubqueryImpl(
CriteriaBuilderImpl criteriaBuilder,
Class javaType,
CommonAbstractCriteria parent) {
super( criteriaBuilder, javaType);
this.parent = parent;
this.queryStructure = new QueryStructure( this, criteriaBuilder, javaType);
}
@Override
public AbstractQuery> getParent() {
if ( ! AbstractQuery.class.isInstance( parent ) ) {
throw new IllegalStateException( "Cannot call getParent on update/delete criterias" );
}
return (AbstractQuery>) parent;
}
@Override
public CommonAbstractCriteria getContainingQuery() {
return parent;
}
@Override
public Class getResultType() {
return getJavaType();
}
// ROOTS ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
@Override
public Set> getRoots() {
return queryStructure.getRoots();
}
@Override
public Root from(Class entityClass) {
return queryStructure.from( entityClass );
}
@Override
public Root from(EntityType entityType) {
return queryStructure.from( entityType );
}
// SELECTION ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
@Override
public Subquery distinct(boolean applyDistinction) {
queryStructure.setDistinct( applyDistinction );
return this;
}
@Override
public boolean isDistinct() {
return queryStructure.isDistinct();
}
private Expression wrappedSelection;
@Override
public Expression getSelection() {
if ( wrappedSelection == null ) {
if ( queryStructure.getSelection() == null ) {
return null;
}
wrappedSelection = new SubquerySelection( (ExpressionImpl) queryStructure.getSelection(), this );
}
return wrappedSelection;
}
@Override
public Subquery select(Expression expression) {
queryStructure.setSelection( expression );
return this;
}
public static class SubquerySelection extends DelegatedExpressionImpl {
private final CriteriaSubqueryImpl subQuery;
public SubquerySelection(ExpressionImpl wrapped, CriteriaSubqueryImpl subQuery) {
super( wrapped );
this.subQuery = subQuery;
}
@Override
public void render(RenderingContext rc) {
subQuery.render(rc);
}
@Override
public void renderProjection(RenderingContext rc) {
render(rc);
}
}
// RESTRICTION ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
@Override
public Predicate getRestriction() {
return queryStructure.getRestriction();
}
@Override
public Subquery where(Expression expression) {
queryStructure.setRestriction( criteriaBuilder().wrap( expression ) );
return this;
}
@Override
public Subquery where(Predicate... predicates) {
// TODO : assuming this should be a conjuntion, but the spec does not say specifically...
queryStructure.setRestriction( criteriaBuilder().and( predicates ) );
return this;
}
// GROUPING ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
@Override
public List> getGroupList() {
return queryStructure.getGroupings();
}
@Override
public Subquery groupBy(Expression>... groupings) {
queryStructure.setGroupings( groupings );
return this;
}
@Override
public Subquery groupBy(List> groupings) {
queryStructure.setGroupings( groupings );
return this;
}
@Override
public Predicate getGroupRestriction() {
return queryStructure.getHaving();
}
@Override
public Subquery having(Expression expression) {
queryStructure.setHaving( criteriaBuilder().wrap( expression ) );
return this;
}
@Override
public Subquery having(Predicate... predicates) {
queryStructure.setHaving( criteriaBuilder().and( predicates ) );
return this;
}
// CORRELATIONS ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
@Override
public Subquery subquery(Class subqueryType) {
return queryStructure.subquery( subqueryType );
}
// rendering ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
@Override
public void render(RenderingContext rc) {
boolean query = rc.isQuery();
rc.append("(");
rc.setQuery(true);
queryStructure.render(rc);
rc.setQuery(query);
rc.append(')');
}
@Override
public void renderProjection(RenderingContext rc) {
throw new IllegalStateException( "Subquery cannot occur in select clause" );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy