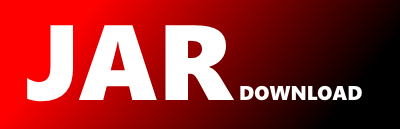
org.ibatis.persist.impl.CriteriaUpdateImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbatis Show documentation
Show all versions of jbatis Show documentation
The jBATIS persistence framework will help you to significantly reduce the amount of Java code that you normally need to access a relational database. iBATIS simply maps JavaBeans to SQL statements using a very simple XML descriptor.
The newest version!
package org.ibatis.persist.impl;
import java.util.ArrayList;
import java.util.List;
import org.ibatis.persist.Parameter;
import org.ibatis.persist.criteria.CriteriaUpdate;
import org.ibatis.persist.criteria.Expression;
import org.ibatis.persist.criteria.Path;
import org.ibatis.persist.criteria.Predicate;
import org.ibatis.persist.impl.path.AttributePathImpl;
import org.ibatis.persist.impl.util.GetterInterceptor;
@SuppressWarnings("unchecked")
public class CriteriaUpdateImpl extends CriteriaManipulation implements CriteriaUpdate {
private List assignments = new ArrayList();
public CriteriaUpdateImpl(CriteriaBuilderImpl criteriaBuilder, Class targetEntity) {
super(criteriaBuilder, targetEntity);
}
@Override
public CriteriaUpdate set(Path attributePath, X value) {
final Expression valueExpression = value == null ? criteriaBuilder().nullLiteral(attributePath.getJavaType())
: criteriaBuilder().literal(value);
addAssignment(attributePath, valueExpression);
return this;
}
@Override
public CriteriaUpdate set(Path attributePath, Expression extends Y> value) {
addAssignment(attributePath, value);
return this;
}
@Override
public CriteriaUpdate set(Y attribute, Y value) {
String attributeName = GetterInterceptor.take();
final Path attributePath = getRoot().get(attributeName);
final Expression valueExpression = value == null ? criteriaBuilder().nullLiteral(attributePath.getJavaType())
: criteriaBuilder().literal(value);
addAssignment(attributePath, valueExpression);
return this;
}
@Override
public CriteriaUpdate set(Y attribute, Expression extends Y> value) {
String attributeName = GetterInterceptor.take();
final Path attributePath = getRoot().get(attributeName);
addAssignment(attributePath, value);
return this;
}
protected void addAssignment(Path attributePath, Expression extends Y> value) {
if (value == null) {
throw new IllegalArgumentException(
"Assignment value expression cannot be null. Did you mean to pass null as a literal?");
}
assignments.add(new Assignment((AttributePathImpl) attributePath, value));
}
@Override
public CriteriaUpdate where(Expression restriction) {
setRestriction(restriction);
return this;
}
@Override
public CriteriaUpdate where(Predicate... restrictions) {
setRestriction(restrictions);
return this;
}
@Override
public void validate() {
super.validate();
if (assignments.isEmpty()) {
throw new IllegalStateException("No assignments specified as part of UPDATE criteria");
}
}
@Override
protected void renderQuery(RenderingContext rc) {
rc.append("update ");
renderRoot( rc);
renderAssignments( rc);
renderRestrictions( rc);
}
private void renderAssignments(RenderingContext rc) {
rc.append(" set ");
boolean first = true;
for (Assignment assignment : assignments) {
if (!first) {
rc.append(", ");
}
assignment.attributePath.render(rc);
rc.append(" = ");
assignment.value.render(rc);
first = false;
}
}
private class Assignment {
private final AttributePathImpl attributePath;
private final ExpressionImplementor extends A> value;
private Assignment(AttributePathImpl attributePath, Expression extends A> value) {
this.attributePath = attributePath;
this.value = (ExpressionImplementor) value;
}
}
@Override
public CriteriaUpdate setParameter(Parameter param, R value) {
ParameterInfo pi = (ParameterInfo) param;
pi.setParameterValue(value);
return this;
}
@Override
public CriteriaUpdate setParameter(String name, R value) {
return setParameter((Parameter) getParameter(name), value);
}
@Override
public CriteriaUpdate setParameter(int position, R value) {
for (Parameter> p : getParameters()) {
if (p.getPosition() != null && p.getPosition() == position) {
setParameter((Parameter) p, value);
return this;
}
}
throw new IllegalArgumentException("" + position);
}
@Override
public Class> getResultType() {
return Integer.class;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy