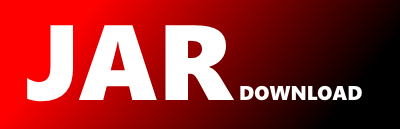
org.ibatis.persist.impl.function.AggregationFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jbatis Show documentation
Show all versions of jbatis Show documentation
The jBATIS persistence framework will help you to significantly reduce the amount of Java code that you normally need to access a relational database. iBATIS simply maps JavaBeans to SQL statements using a very simple XML descriptor.
The newest version!
package org.ibatis.persist.impl.function;
import org.ibatis.persist.criteria.Expression;
import org.ibatis.persist.impl.CriteriaBuilderImpl;
import org.ibatis.persist.impl.RenderingContext;
/**
* Models SQL aggregation functions (MIN, MAX, COUNT, etc).
*/
public class AggregationFunction
extends ParameterizedFunctionExpression {
/**
* Constructs an aggregation function with a single literal argument.
*
* @param criteriaBuilder The query builder instance.
* @param returnType The function return type.
* @param functionName The name of the function.
* @param argument The argument
*/
public AggregationFunction(
CriteriaBuilderImpl criteriaBuilder,
Class returnType,
String functionName,
Expression> argument) {
super( criteriaBuilder, returnType, functionName, argument );
}
@Override
public boolean isAggregation() {
return true;
}
@Override
protected boolean isStandardJpaFunction() {
return true;
}
/**
* Implementation of a COUNT function providing convenience in construction.
*
* Parameterized as {@link Long} because thats what JPA states
* that the return from COUNT should be.
*/
public static class COUNT extends AggregationFunction {
public static final String NAME = "count";
private final boolean distinct;
public COUNT(CriteriaBuilderImpl criteriaBuilder, Expression> expression, boolean distinct) {
super( criteriaBuilder, Long.class, NAME , expression );
this.distinct = distinct;
}
@Override
protected void renderArguments(RenderingContext rc) {
if ( isDistinct() ) {
rc.append("distinct ");
}
super.renderArguments(rc);
}
public boolean isDistinct() {
return distinct;
}
}
/**
* Implementation of a AVG function providing convenience in construction.
*
* Parameterized as {@link Double} because thats what JPA states that the return from AVG should be.
*/
public static class AVG extends AggregationFunction {
public static final String NAME = "avg";
public AVG(CriteriaBuilderImpl criteriaBuilder, Expression extends Number> expression) {
super( criteriaBuilder, Double.class, NAME, expression );
}
}
/**
* Implementation of a SUM function providing convenience in construction.
*
* Parameterized as {@link Number N extends Number} because thats what JPA states
* that the return from SUM should be.
*/
public static class SUM extends AggregationFunction {
public static final String NAME = "sum";
@SuppressWarnings({ "unchecked" })
public SUM(CriteriaBuilderImpl criteriaBuilder, Expression expression) {
super( criteriaBuilder, (Class)expression.getJavaType(), NAME , expression);
// force the use of a ValueHandler
setJavaType( expression.getJavaType() );
}
public SUM(CriteriaBuilderImpl criteriaBuilder, Expression extends Number> expression, Class returnType) {
super( criteriaBuilder, returnType, NAME , expression);
// force the use of a ValueHandler
setJavaType( returnType );
}
}
/**
* Implementation of a MIN function providing convenience in construction.
*
* Parameterized as {@link Number N extends Number} because thats what JPA states
* that the return from MIN should be.
*/
public static class MIN extends AggregationFunction {
public static final String NAME = "min";
@SuppressWarnings({ "unchecked" })
public MIN(CriteriaBuilderImpl criteriaBuilder, Expression expression) {
super( criteriaBuilder, ( Class ) expression.getJavaType(), NAME , expression);
}
}
/**
* Implementation of a MAX function providing convenience in construction.
*
* Parameterized as {@link Number N extends Number} because thats what JPA states
* that the return from MAX should be.
*/
public static class MAX extends AggregationFunction {
public static final String NAME = "max";
@SuppressWarnings({ "unchecked" })
public MAX(CriteriaBuilderImpl criteriaBuilder, Expression expression) {
super( criteriaBuilder, ( Class ) expression.getJavaType(), NAME , expression);
}
}
/**
* Models the MIN function in terms of non-numeric expressions.
*
* @see MIN
*/
public static class LEAST> extends AggregationFunction {
public static final String NAME = "min";
@SuppressWarnings({ "unchecked" })
public LEAST(CriteriaBuilderImpl criteriaBuilder, Expression expression) {
super( criteriaBuilder, ( Class ) expression.getJavaType(), NAME , expression);
}
}
/**
* Models the MAX function in terms of non-numeric expressions.
*
* @see MAX
*/
public static class GREATEST> extends AggregationFunction {
public static final String NAME = "max";
@SuppressWarnings({ "unchecked" })
public GREATEST(CriteriaBuilderImpl criteriaBuilder, Expression expression) {
super( criteriaBuilder, ( Class ) expression.getJavaType(), NAME , expression);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy