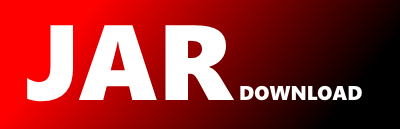
com.dyuproject.protostuff.json.InlineValue Maven / Gradle / Ivy
//========================================================================
//Copyright 2007-2008 David Yu [email protected]
//------------------------------------------------------------------------
//Licensed under the Apache License, Version 2.0 (the "License");
//you may not use this file except in compliance with the License.
//You may obtain a copy of the License at
//http://www.apache.org/licenses/LICENSE-2.0
//Unless required by applicable law or agreed to in writing, software
//distributed under the License is distributed on an "AS IS" BASIS,
//WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
//See the License for the specific language governing permissions and
//limitations under the License.
//========================================================================
package com.dyuproject.protostuff.json;
import java.io.IOException;
import java.lang.reflect.Method;
import org.codehaus.jackson.JsonGenerator;
import org.codehaus.jackson.JsonParser;
import org.codehaus.jackson.JsonToken;
import com.google.protobuf.ByteString;
import com.google.protobuf.Internal.EnumLite;
import com.google.protobuf.WireFormat.JavaType;
/**
* Represents scalar values from protobuf fields.
*
* @author David Yu
* @created Oct 1, 2009
*/
public abstract class InlineValue
{
public static InlineValue> getPrimitive(JavaType type)
{
switch(type)
{
case INT:
return INT_VALUE;
case LONG:
return LONG_VALUE;
case BOOLEAN:
return BOOLEAN_VALUE;
case FLOAT:
return FLOAT_VALUE;
case DOUBLE:
return DOUBLE_VALUE;
case STRING:
return STRING_VALUE;
case BYTE_STRING:
return BYTESTRING_VALUE;
default:
return null;
}
}
public static InlineValue INT_VALUE = new InlineValue()
{
public Integer readFrom(JsonParser parser) throws IOException
{
return parser.getIntValue();
}
public void writeTo(JsonGenerator generator, Integer value) throws IOException
{
generator.writeNumber(value);
}
public JavaType getJavaType()
{
return JavaType.INT;
}
};
public static InlineValue LONG_VALUE = new InlineValue()
{
public Long readFrom(JsonParser parser) throws IOException
{
return parser.getLongValue();
}
public void writeTo(JsonGenerator generator, Long value) throws IOException
{
generator.writeNumber(value);
}
public JavaType getJavaType()
{
return JavaType.LONG;
}
};
public static InlineValue BOOLEAN_VALUE = new InlineValue()
{
public Boolean readFrom(JsonParser parser) throws IOException
{
JsonToken jt = parser.getCurrentToken();
if(jt==JsonToken.VALUE_FALSE)
return false;
if(jt==JsonToken.VALUE_TRUE)
return true;
throw new IOException("Expected token: true/false but was " + jt);
}
public void writeTo(JsonGenerator generator, Boolean value) throws IOException
{
generator.writeBoolean(value);
}
public JavaType getJavaType()
{
return JavaType.BOOLEAN;
}
};
public static InlineValue FLOAT_VALUE = new InlineValue()
{
public Float readFrom(JsonParser parser) throws IOException
{
return parser.getFloatValue();
}
public void writeTo(JsonGenerator generator, Float value) throws IOException
{
generator.writeNumber(value);
}
public JavaType getJavaType()
{
return JavaType.FLOAT;
}
};
public static InlineValue DOUBLE_VALUE = new InlineValue()
{
public Double readFrom(JsonParser parser) throws IOException
{
return parser.getDoubleValue();
}
public void writeTo(JsonGenerator generator, Double value) throws IOException
{
generator.writeNumber(value);
}
public JavaType getJavaType()
{
return JavaType.DOUBLE;
}
};
public static InlineValue STRING_VALUE = new InlineValue()
{
public String readFrom(JsonParser parser) throws IOException
{
return parser.getText();
}
public void writeTo(JsonGenerator generator, String value) throws IOException
{
generator.writeString(value);
}
public JavaType getJavaType()
{
return JavaType.STRING;
}
};
public static InlineValue BYTESTRING_VALUE = new InlineValue()
{
public ByteString readFrom(JsonParser parser) throws IOException
{
return ByteString.copyFrom(parser.getBinaryValue());
//return ByteString.copyFromUtf8(parser.getText());
}
public void writeTo(JsonGenerator generator, ByteString value) throws IOException
{
generator.writeBinary(value.toByteArray());
//generator.writeString(value.toStringUtf8());
}
public JavaType getJavaType()
{
return JavaType.BYTE_STRING;
}
};
public abstract T readFrom(JsonParser parser) throws IOException;
public abstract void writeTo(JsonGenerator generator, T value) throws IOException;
public abstract JavaType getJavaType();
@SuppressWarnings("unchecked")
public void writeObjectTo(JsonGenerator generator, Object value) throws IOException
{
writeTo(generator, (T)value);
}
public static class EnumValue extends InlineValue
{
@SuppressWarnings("unchecked")
public static final EnumValue create(Class> enumClass)
{
if(!enumClass.isEnum())
throw new RuntimeException("Not an enum class: " + enumClass);
if(!EnumLite.class.isAssignableFrom(enumClass))
throw new RuntimeException("Enum not generated by protoc: " + enumClass);
return new EnumValue((Class)enumClass);
}
//private final Class _clazz;
private final Method _valueOf;
private EnumValue(Class clazz)
{
//_clazz = clazz;
try
{
_valueOf = clazz.getDeclaredMethod("valueOf", new Class[]{int.class});
}
catch (Exception e)
{
throw new RuntimeException(e);
}
}
@SuppressWarnings("unchecked")
public E readFrom(JsonParser parser) throws IOException
{
// read via int value
try
{
return (E)_valueOf.invoke(null, new Object[]{parser.getIntValue()});
}
catch (Exception e)
{
throw new RuntimeException(e);
}
//return Enum.valueOf(_clazz, parser.getText());
}
public void writeTo(JsonGenerator generator, E value) throws IOException
{
generator.writeNumber(value.getNumber());
//generator.writeString(value.name());
}
public JavaType getJavaType()
{
return JavaType.ENUM;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy