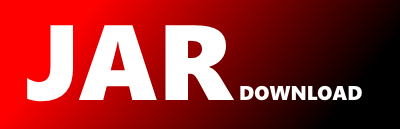
com.ea.orbit.concurrent.Task Maven / Gradle / Ivy
/*
Copyright (C) 2015 Electronic Arts Inc. All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions
are met:
1. Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
3. Neither the name of Electronic Arts, Inc. ("EA") nor the names of
its contributors may be used to endorse or promote products derived
from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY ELECTRONIC ARTS AND ITS CONTRIBUTORS "AS IS" AND ANY
EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL ELECTRONIC ARTS OR ITS CONTRIBUTORS BE LIABLE FOR ANY
DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.ea.orbit.concurrent;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.util.concurrent.Executor;
import java.util.concurrent.Future;
import java.util.concurrent.RejectedExecutionException;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.function.BiConsumer;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* Task are CompletableFutures were with a few changes.
*
*
* - complete and completeExceptionally are not publicly accessible.
* - few utility methods (thenRun, thenReturn)
* - TODO: all callbacks are async by default using the current executor
*
*
*
* @param the type of the returned object.
* @see java.util.concurrent.CompletableFuture
*/
public class Task extends CompletableFuture
{
private static Void NIL = null;
// TODO: make all callbacks async by default and using the current executor
// what "current executor' means will have to be defined.
// the idea is to use a framework supplied executor to serve
// single point to capture all activity derived from the execution
// of one application request.
// Including logs, stats, exception and timing information.
// TODO: add here or elsewhere a method to wrap a task with timeout
// example: Task result = aTask.timeout(60, TimeUnit.SECONDS);
// TODO: consider implementing CompletionStage instead of deriving from CompletableFuture.
// TODO: consider creating a public class CTask = "Completable Task"
/**
* Creates an already completed task from the given value.
*
* @param value the value to be wrapped with a task
*/
public static Task fromValue(T value)
{
final Task t = new Task();
t.internalComplete(value);
return t;
}
public static Task fromException(Throwable ex)
{
final Task t = new Task();
t.internalCompleteExceptionally(ex);
return t;
}
protected boolean internalComplete(T value)
{
return super.complete(value);
}
protected boolean internalCompleteExceptionally(Throwable ex)
{
return super.completeExceptionally(ex);
}
/**
* This completableFuture derived method is not available for Tasks.
*/
@Override
@Deprecated
public boolean complete(T value)
{
// TODO: throw an exception
return super.complete(value);
}
/**
* This completableFuture derived method is not available for Tasks.
*/
@Override
@Deprecated
public boolean completeExceptionally(Throwable ex)
{
// TODO: throw an exception
return super.completeExceptionally(ex);
}
/**
* Wraps a CompletionStage as a Task or just casts it if it is already a Task.
*
* @param stage the stage to be wrapped or casted to Task
* @return stage cast as Task of a new Task that is dependent on the completion of that stage.
*/
public static Task from(CompletionStage stage)
{
if (stage instanceof Task)
{
return (Task) stage;
}
final Task t = new Task();
stage.handle((T v, Throwable ex) -> {
if (ex != null)
{
t.internalCompleteExceptionally(ex);
}
else
{
t.internalComplete(v);
}
return null;
});
return t;
}
/**
* Wraps a Future as a Task or just casts it if it is already a Task.
* If future implements CompletionStage CompletionStage.handle will be used.
*
* If future is a plain old future, a runnable will executed using a default task pool.
* This runnable will wait on the future using Future.get(timeout,timeUnit), with a timeout of 5ms.
* Every time this task times out it will be rescheduled.
* Starvation of the pool is prevented by the rescheduling behaviour.
*
*
* @param future the future to be wrapped or casted to Task
* @return future cast as Task of a new Task that is dependent on the completion of that future.
*/
@SuppressWarnings("unchecked")
public static Task fromFuture(Future future)
{
if (future instanceof Task)
{
return (Task) future;
}
if (future instanceof CompletionStage)
{
return from((CompletionStage) future);
}
final Task t = new Task();
if (future.isDone())
{
try
{
t.internalComplete(future.get());
}
catch (Throwable ex)
{
t.internalCompleteExceptionally(ex);
}
return t;
}
// potentially very expensive
commonPool.execute(new TaskFutureAdapter(t, future, commonPool, 5, TimeUnit.MILLISECONDS));
return t;
}
private static Executor commonPool = ExecutorUtils.newScalingThreadPool(100);
static class TaskFutureAdapter implements Runnable
{
Task task;
Future future;
Executor executor;
long waitTimeout;
TimeUnit waitTimeoutUnit;
public TaskFutureAdapter(
final Task task,
final Future future,
final Executor executor,
long waitTimeout, TimeUnit waitTimeoutUnit)
{
this.task = task;
this.future = future;
this.executor = executor;
this.waitTimeout = waitTimeout;
this.waitTimeoutUnit = waitTimeoutUnit;
}
public void run()
{
try
{
while (!task.isDone())
{
try
{
task.internalComplete(future.get(waitTimeout, waitTimeoutUnit));
return;
}
catch (TimeoutException ex)
{
if (future.isDone())
{
// in this case something completed the future with a timeout exception.
try
{
task.internalComplete(future.get(waitTimeout, waitTimeoutUnit));
return;
}
catch (Throwable tex0)
{
task.internalCompleteExceptionally(tex0);
}
return;
}
try
{
// reschedule
// potentially very expensive, might limit request throughput
executor.execute(this);
return;
}
catch (RejectedExecutionException rex)
{
// ignoring and continuing.
// might potentially worsen an already starving system.
continue;
}
catch (Throwable tex)
{
task.internalCompleteExceptionally(tex);
return;
}
}
catch (Throwable ex)
{
task.internalCompleteExceptionally(ex);
return;
}
}
}
catch (Throwable ex)
{
task.internalCompleteExceptionally(ex);
}
}
}
public static Task done()
{
final Task task = new Task();
task.internalComplete(NIL);
return task;
}
@Override
public Task thenApply(final Function super T, ? extends U> fn)
{
return from(super.thenApply(fn));
}
@Override
public Task thenAccept(final Consumer super T> action)
{
return from(super.thenAccept(action));
}
@Override
public Task whenComplete(final BiConsumer super T, ? super Throwable> action)
{
return from(super.whenComplete(action));
}
/**
* Returns a new Task that is executed when this task completes normally.
* The result of the new Task will be the result of the Supplier passed as parameter.
*
* See the {@link CompletionStage} documentation for rules
* covering exceptional completion.
*
* @param supplier the Supplier that will provider the value
* the returned Task
* @param the supplier's return type
* @return the new Task
*/
public Task thenReturn(final Supplier supplier)
{
return from(super.thenApply(x -> supplier.get()));
}
public Task thenCompose(Function super T, ? extends CompletionStage> fn)
{
return Task.from(super.thenCompose(fn));
}
public Task thenCompose(Supplier extends CompletionStage> fn)
{
return Task.from(super.thenCompose((T x) -> fn.get()));
}
@Override
public Task handle(final BiFunction super T, Throwable, ? extends U> fn)
{
return from(super.handle(fn));
}
@Override
public Task exceptionally(final Function fn)
{
return from(super.exceptionally(fn));
}
@Override
public Task thenRun(final Runnable action)
{
return from(super.thenRun(action));
}
/**
* @throws NullPointerException if the array or any of its elements are
* {@code null}
*/
public static Task allOf(CompletableFuture>... cfs)
{
return from(CompletableFuture.allOf(cfs));
}
/**
* @throws NullPointerException if the collection or any of its elements are
* {@code null}
*/
public static , C extends Collection> Task allOf(C cfs)
{
return from(CompletableFuture.allOf(cfs.toArray(new CompletableFuture[cfs.size()]))
.thenApply(x -> cfs));
}
/**
* @throws NullPointerException if the stream or any of its elements are
* {@code null}
*/
public static > Task> allOf(Stream cfs)
{
final List futureList = cfs.collect(Collectors.toList());
@SuppressWarnings("rawtypes")
final CompletableFuture[] futureArray = futureList.toArray(new CompletableFuture[futureList.size()]);
return from(CompletableFuture.allOf(futureArray).thenApply(x -> futureList));
}
/**
* @throws NullPointerException if the array or any of its elements are
* {@code null}
*/
public static Task
© 2015 - 2025 Weber Informatics LLC | Privacy Policy