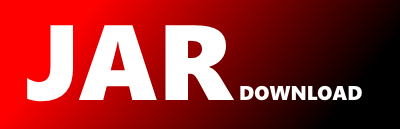
io.swagger.client.api.GroupsApi Maven / Gradle / Ivy
The newest version!
/*
* Easemob REST APIs
* Easemob Server REST API Swagger is designated to provide better documentation and thorough interfaces for testing. For more details about server implementation, request rate limitation, etc, please visit [Easemob Server Integration](http://docs.easemob.com/im/100serverintegration/10intro). **Note:** `org_ID` is the unique ID of the organization created when you first registered [Easemob console](https://console.easemob.com/). `app_name` is the unique app ID created when you new application in [Easemob console](https://console.easemob.com/). `Authorization token` is required for most API requests as part of requesting header in the format `Bearer ${token}`. You can obtain the token via [/{org_name}/{app_name}/token](https://docs.hyphenate.io/docs/server-overview#section-request-authentication-token).
*
* OpenAPI spec version: 1.0.2
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.api;
import io.swagger.client.ApiCallback;
import io.swagger.client.ApiClient;
import io.swagger.client.ApiException;
import io.swagger.client.ApiResponse;
import io.swagger.client.Configuration;
import io.swagger.client.Pair;
import io.swagger.client.ProgressRequestBody;
import io.swagger.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.swagger.client.model.Group;
import io.swagger.client.model.ModifyGroup;
import io.swagger.client.model.NewOwner;
import io.swagger.client.model.UserNames;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class GroupsApi {
private ApiClient apiClient;
public GroupsApi() {
this(Configuration.getDefaultApiClient());
}
public GroupsApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/* Build call for orgNameAppNameChatgroupsGet */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGetCall(String orgName, String appName, String authorization, String limit, String cursor, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()));
List localVarQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "limit", limit));
if (cursor != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "cursor", cursor));
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGetValidateBeforeCall(String orgName, String appName, String authorization, String limit, String cursor, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGetCall(orgName, appName, authorization, limit, cursor, progressListener, progressRequestListener);
return call;
}
/**
* Get All the Groups
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit total number of messages per page by pagination at a time (optional, default to )
* @param cursor Get messages by pagination. Obtained \"cursor\" from the previous GET messages call response. (optional, default to )
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGet(String orgName, String appName, String authorization, String limit, String cursor) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGetWithHttpInfo(orgName, appName, authorization, limit, cursor);
return resp.getData();
}
/**
* Get All the Groups
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit total number of messages per page by pagination at a time (optional, default to )
* @param cursor Get messages by pagination. Obtained \"cursor\" from the previous GET messages call response. (optional, default to )
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGetWithHttpInfo(String orgName, String appName, String authorization, String limit, String cursor) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGetValidateBeforeCall(orgName, appName, authorization, limit, cursor, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get All the Groups (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit total number of messages per page by pagination at a time (optional, default to )
* @param cursor Get messages by pagination. Obtained \"cursor\" from the previous GET messages call response. (optional, default to )
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGetAsync(String orgName, String appName, String authorization, String limit, String cursor, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGetValidateBeforeCall(orgName, appName, authorization, limit, cursor, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdBlocksUsersGet */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersGetCall(String orgName, String appName, String authorization, String groupId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/blocks/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersGetValidateBeforeCall(String orgName, String appName, String authorization, String groupId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersGet(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersGetCall(orgName, appName, authorization, groupId, progressListener, progressRequestListener);
return call;
}
/**
* Get Group Blocked Users
* Get the blacklist of blocked users. Blocked user cannot see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdBlocksUsersGet(String orgName, String appName, String authorization, String groupId) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdBlocksUsersGetWithHttpInfo(orgName, appName, authorization, groupId);
return resp.getData();
}
/**
* Get Group Blocked Users
* Get the blacklist of blocked users. Blocked user cannot see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdBlocksUsersGetWithHttpInfo(String orgName, String appName, String authorization, String groupId) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersGetValidateBeforeCall(orgName, appName, authorization, groupId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get Group Blocked Users (asynchronously)
* Get the blacklist of blocked users. Blocked user cannot see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersGetAsync(String orgName, String appName, String authorization, String groupId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersGetValidateBeforeCall(orgName, appName, authorization, groupId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdBlocksUsersPost */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersPostCall(String orgName, String appName, String authorization, String groupId, UserNames body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/blocks/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersPostValidateBeforeCall(String orgName, String appName, String authorization, String groupId, UserNames body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersPost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersPost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersPost(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersPost(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersPost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersPostCall(orgName, appName, authorization, groupId, body, progressListener, progressRequestListener);
return call;
}
/**
* Block Group Members in Batch
* Block multiple group members by adding the users to the group blacklist. Max 60 users at a time. Blocked users will receive an event, \"You are kicked out of the group {groupid}\". Blocked user will not able to see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body Separate usernames by ',' (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdBlocksUsersPost(String orgName, String appName, String authorization, String groupId, UserNames body) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdBlocksUsersPostWithHttpInfo(orgName, appName, authorization, groupId, body);
return resp.getData();
}
/**
* Block Group Members in Batch
* Block multiple group members by adding the users to the group blacklist. Max 60 users at a time. Blocked users will receive an event, \"You are kicked out of the group {groupid}\". Blocked user will not able to see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body Separate usernames by ',' (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdBlocksUsersPostWithHttpInfo(String orgName, String appName, String authorization, String groupId, UserNames body) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersPostValidateBeforeCall(orgName, appName, authorization, groupId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Block Group Members in Batch (asynchronously)
* Block multiple group members by adding the users to the group blacklist. Max 60 users at a time. Blocked users will receive an event, \"You are kicked out of the group {groupid}\". Blocked user will not able to see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body Separate usernames by ',' (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersPostAsync(String orgName, String appName, String authorization, String groupId, UserNames body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersPostValidateBeforeCall(orgName, appName, authorization, groupId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDelete */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDeleteCall(String orgName, String appName, String authorization, String groupId, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/blocks/users/{username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDeleteValidateBeforeCall(String orgName, String appName, String authorization, String groupId, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDelete(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDelete(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDeleteCall(orgName, appName, authorization, groupId, username, progressListener, progressRequestListener);
return call;
}
/**
* Unblock a Group Member
* Unblock group member by removing the user from group blacklist.
* @param orgName Organization ID (required)
* @param appName testapp (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDelete(String orgName, String appName, String authorization, String groupId, String username) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDeleteWithHttpInfo(orgName, appName, authorization, groupId, username);
return resp.getData();
}
/**
* Unblock a Group Member
* Unblock group member by removing the user from group blacklist.
* @param orgName Organization ID (required)
* @param appName testapp (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDeleteWithHttpInfo(String orgName, String appName, String authorization, String groupId, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDeleteValidateBeforeCall(orgName, appName, authorization, groupId, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Unblock a Group Member (asynchronously)
* Unblock group member by removing the user from group blacklist.
* @param orgName Organization ID (required)
* @param appName testapp (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDeleteAsync(String orgName, String appName, String authorization, String groupId, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernameDeleteValidateBeforeCall(orgName, appName, authorization, groupId, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePost */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePostCall(String orgName, String appName, String authorization, String groupId, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/blocks/users/{username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePostValidateBeforeCall(String orgName, String appName, String authorization, String groupId, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePost(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePost(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePostCall(orgName, appName, authorization, groupId, username, progressListener, progressRequestListener);
return call;
}
/**
* Block a Group Member
* Block a group member by adding the user to the group blacklist. Blocked user will receive an event, \"You are kicked out of the group {groupid}\". Blocked user will not able to see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePost(String orgName, String appName, String authorization, String groupId, String username) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePostWithHttpInfo(orgName, appName, authorization, groupId, username);
return resp.getData();
}
/**
* Block a Group Member
* Block a group member by adding the user to the group blacklist. Blocked user will receive an event, \"You are kicked out of the group {groupid}\". Blocked user will not able to see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePostWithHttpInfo(String orgName, String appName, String authorization, String groupId, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePostValidateBeforeCall(orgName, appName, authorization, groupId, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Block a Group Member (asynchronously)
* Block a group member by adding the user to the group blacklist. Blocked user will receive an event, \"You are kicked out of the group {groupid}\". Blocked user will not able to see nor receive group message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePostAsync(String orgName, String appName, String authorization, String groupId, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamePostValidateBeforeCall(orgName, appName, authorization, groupId, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDelete */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDeleteCall(String orgName, String appName, String authorization, String groupId, String usernames, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/blocks/users/{usernames}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "usernames" + "\\}", apiClient.escapeString(usernames.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDeleteValidateBeforeCall(String orgName, String appName, String authorization, String groupId, String usernames, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDelete(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDelete(Async)");
}
// verify the required parameter 'usernames' is set
if (usernames == null) {
throw new ApiException("Missing the required parameter 'usernames' when calling orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDeleteCall(orgName, appName, authorization, groupId, usernames, progressListener, progressRequestListener);
return call;
}
/**
* Unblock Group Members in Batch
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param usernames Separate usernames by ',' (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDelete(String orgName, String appName, String authorization, String groupId, String usernames) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDeleteWithHttpInfo(orgName, appName, authorization, groupId, usernames);
return resp.getData();
}
/**
* Unblock Group Members in Batch
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param usernames Separate usernames by ',' (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDeleteWithHttpInfo(String orgName, String appName, String authorization, String groupId, String usernames) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDeleteValidateBeforeCall(orgName, appName, authorization, groupId, usernames, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Unblock Group Members in Batch (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param usernames Separate usernames by ',' (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDeleteAsync(String orgName, String appName, String authorization, String groupId, String usernames, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdBlocksUsersUsernamesDeleteValidateBeforeCall(orgName, appName, authorization, groupId, usernames, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdDelete */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdDeleteCall(String orgName, String appName, String authorization, String groupId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdDeleteValidateBeforeCall(String orgName, String appName, String authorization, String groupId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdDelete(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdDeleteCall(orgName, appName, authorization, groupId, progressListener, progressRequestListener);
return call;
}
/**
* Delete a Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdDelete(String orgName, String appName, String authorization, String groupId) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdDeleteWithHttpInfo(orgName, appName, authorization, groupId);
return resp.getData();
}
/**
* Delete a Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdDeleteWithHttpInfo(String orgName, String appName, String authorization, String groupId) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdDeleteValidateBeforeCall(orgName, appName, authorization, groupId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Delete a Group (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdDeleteAsync(String orgName, String appName, String authorization, String groupId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdDeleteValidateBeforeCall(orgName, appName, authorization, groupId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdPut */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdPutCall(String orgName, String appName, String authorization, String groupId, ModifyGroup body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdPutValidateBeforeCall(String orgName, String appName, String authorization, String groupId, ModifyGroup body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdPut(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdPut(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdPut(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdPut(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling orgNameAppNameChatgroupsGroupIdPut(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdPutCall(orgName, appName, authorization, groupId, body, progressListener, progressRequestListener);
return call;
}
/**
* Update Group Details
* The message body only allows groupname, description, and maxusers. Note: Use '+' to replace space if modifying groupname ?and description. E.g., use \"test+group\" instead of \"test group\". Warning: If group cannot be found or operation failed, then the response will still return HTTP code 200, but key-value are \"maxusers\"=false, \"groupname\"=false, and \"description\"=false.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdPut(String orgName, String appName, String authorization, String groupId, ModifyGroup body) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdPutWithHttpInfo(orgName, appName, authorization, groupId, body);
return resp.getData();
}
/**
* Update Group Details
* The message body only allows groupname, description, and maxusers. Note: Use '+' to replace space if modifying groupname ?and description. E.g., use \"test+group\" instead of \"test group\". Warning: If group cannot be found or operation failed, then the response will still return HTTP code 200, but key-value are \"maxusers\"=false, \"groupname\"=false, and \"description\"=false.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdPutWithHttpInfo(String orgName, String appName, String authorization, String groupId, ModifyGroup body) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdPutValidateBeforeCall(orgName, appName, authorization, groupId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update Group Details (asynchronously)
* The message body only allows groupname, description, and maxusers. Note: Use '+' to replace space if modifying groupname ?and description. E.g., use \"test+group\" instead of \"test group\". Warning: If group cannot be found or operation failed, then the response will still return HTTP code 200, but key-value are \"maxusers\"=false, \"groupname\"=false, and \"description\"=false.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdPutAsync(String orgName, String appName, String authorization, String groupId, ModifyGroup body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdPutValidateBeforeCall(orgName, appName, authorization, groupId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdUsersGet */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersGetCall(String orgName, String appName, String authorization, String groupId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersGetValidateBeforeCall(String orgName, String appName, String authorization, String groupId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdUsersGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdUsersGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdUsersGet(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdUsersGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersGetCall(orgName, appName, authorization, groupId, progressListener, progressRequestListener);
return call;
}
/**
* Get a List of Members of Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdUsersGet(String orgName, String appName, String authorization, String groupId) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdUsersGetWithHttpInfo(orgName, appName, authorization, groupId);
return resp.getData();
}
/**
* Get a List of Members of Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdUsersGetWithHttpInfo(String orgName, String appName, String authorization, String groupId) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersGetValidateBeforeCall(orgName, appName, authorization, groupId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a List of Members of Group (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersGetAsync(String orgName, String appName, String authorization, String groupId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersGetValidateBeforeCall(orgName, appName, authorization, groupId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdUsersMembersDelete */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersMembersDeleteCall(String orgName, String appName, String authorization, String groupId, String members, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/users/{members}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "members" + "\\}", apiClient.escapeString(members.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersMembersDeleteValidateBeforeCall(String orgName, String appName, String authorization, String groupId, String members, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdUsersMembersDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdUsersMembersDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdUsersMembersDelete(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdUsersMembersDelete(Async)");
}
// verify the required parameter 'members' is set
if (members == null) {
throw new ApiException("Missing the required parameter 'members' when calling orgNameAppNameChatgroupsGroupIdUsersMembersDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersMembersDeleteCall(orgName, appName, authorization, groupId, members, progressListener, progressRequestListener);
return call;
}
/**
* Remove multiple Member from the Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token (required)
* @param groupId (required)
* @param members Use ',' to separate usernames (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdUsersMembersDelete(String orgName, String appName, String authorization, String groupId, String members) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdUsersMembersDeleteWithHttpInfo(orgName, appName, authorization, groupId, members);
return resp.getData();
}
/**
* Remove multiple Member from the Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token (required)
* @param groupId (required)
* @param members Use ',' to separate usernames (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdUsersMembersDeleteWithHttpInfo(String orgName, String appName, String authorization, String groupId, String members) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersMembersDeleteValidateBeforeCall(orgName, appName, authorization, groupId, members, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Remove multiple Member from the Group (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token (required)
* @param groupId (required)
* @param members Use ',' to separate usernames (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersMembersDeleteAsync(String orgName, String appName, String authorization, String groupId, String members, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersMembersDeleteValidateBeforeCall(orgName, appName, authorization, groupId, members, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdUsersPost */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersPostCall(String orgName, String appName, String authorization, String groupId, UserNames body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersPostValidateBeforeCall(String orgName, String appName, String authorization, String groupId, UserNames body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdUsersPost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdUsersPost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdUsersPost(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdUsersPost(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling orgNameAppNameChatgroupsGroupIdUsersPost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersPostCall(orgName, appName, authorization, groupId, body, progressListener, progressRequestListener);
return call;
}
/**
* Add Multiple Members to Group
* Warning: Add max 60 members once at a time.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body Separate usernames by ',' (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdUsersPost(String orgName, String appName, String authorization, String groupId, UserNames body) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdUsersPostWithHttpInfo(orgName, appName, authorization, groupId, body);
return resp.getData();
}
/**
* Add Multiple Members to Group
* Warning: Add max 60 members once at a time.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body Separate usernames by ',' (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdUsersPostWithHttpInfo(String orgName, String appName, String authorization, String groupId, UserNames body) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersPostValidateBeforeCall(orgName, appName, authorization, groupId, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add Multiple Members to Group (asynchronously)
* Warning: Add max 60 members once at a time.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param body Separate usernames by ',' (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersPostAsync(String orgName, String appName, String authorization, String groupId, UserNames body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersPostValidateBeforeCall(orgName, appName, authorization, groupId, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdUsersUsernameDelete */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersUsernameDeleteCall(String orgName, String appName, String authorization, String groupId, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/users/{username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersUsernameDeleteValidateBeforeCall(String orgName, String appName, String authorization, String groupId, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdUsersUsernameDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdUsersUsernameDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdUsersUsernameDelete(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdUsersUsernameDelete(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameChatgroupsGroupIdUsersUsernameDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersUsernameDeleteCall(orgName, appName, authorization, groupId, username, progressListener, progressRequestListener);
return call;
}
/**
* Remove a Member from the Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdUsersUsernameDelete(String orgName, String appName, String authorization, String groupId, String username) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdUsersUsernameDeleteWithHttpInfo(orgName, appName, authorization, groupId, username);
return resp.getData();
}
/**
* Remove a Member from the Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdUsersUsernameDeleteWithHttpInfo(String orgName, String appName, String authorization, String groupId, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersUsernameDeleteValidateBeforeCall(orgName, appName, authorization, groupId, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Remove a Member from the Group (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersUsernameDeleteAsync(String orgName, String appName, String authorization, String groupId, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersUsernameDeleteValidateBeforeCall(orgName, appName, authorization, groupId, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdUsersUsernamePost */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersUsernamePostCall(String orgName, String appName, String authorization, String groupId, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_id}/users/{username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_id" + "\\}", apiClient.escapeString(groupId.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersUsernamePostValidateBeforeCall(String orgName, String appName, String authorization, String groupId, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdUsersUsernamePost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdUsersUsernamePost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdUsersUsernamePost(Async)");
}
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling orgNameAppNameChatgroupsGroupIdUsersUsernamePost(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameChatgroupsGroupIdUsersUsernamePost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersUsernamePostCall(orgName, appName, authorization, groupId, username, progressListener, progressRequestListener);
return call;
}
/**
* Add a Member to Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdUsersUsernamePost(String orgName, String appName, String authorization, String groupId, String username) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdUsersUsernamePostWithHttpInfo(orgName, appName, authorization, groupId, username);
return resp.getData();
}
/**
* Add a Member to Group
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdUsersUsernamePostWithHttpInfo(String orgName, String appName, String authorization, String groupId, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersUsernamePostValidateBeforeCall(orgName, appName, authorization, groupId, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add a Member to Group (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupId (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdUsersUsernamePostAsync(String orgName, String appName, String authorization, String groupId, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdUsersUsernamePostValidateBeforeCall(orgName, appName, authorization, groupId, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupIdsGet */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdsGetCall(String orgName, String appName, String authorization, String groupIds, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{group_ids}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "group_ids" + "\\}", apiClient.escapeString(groupIds.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdsGetValidateBeforeCall(String orgName, String appName, String authorization, String groupIds, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupIdsGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupIdsGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupIdsGet(Async)");
}
// verify the required parameter 'groupIds' is set
if (groupIds == null) {
throw new ApiException("Missing the required parameter 'groupIds' when calling orgNameAppNameChatgroupsGroupIdsGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdsGetCall(orgName, appName, authorization, groupIds, progressListener, progressRequestListener);
return call;
}
/**
* Get Group(s) Details
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupIds Separate group ID by ','. e.g. {group_id1},{group_id2} (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupIdsGet(String orgName, String appName, String authorization, String groupIds) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupIdsGetWithHttpInfo(orgName, appName, authorization, groupIds);
return resp.getData();
}
/**
* Get Group(s) Details
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupIds Separate group ID by ','. e.g. {group_id1},{group_id2} (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupIdsGetWithHttpInfo(String orgName, String appName, String authorization, String groupIds) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdsGetValidateBeforeCall(orgName, appName, authorization, groupIds, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get Group(s) Details (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupIds Separate group ID by ','. e.g. {group_id1},{group_id2} (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupIdsGetAsync(String orgName, String appName, String authorization, String groupIds, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupIdsGetValidateBeforeCall(orgName, appName, authorization, groupIds, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsGroupidPut */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupidPutCall(String orgName, String appName, String authorization, String groupid, NewOwner body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups/{groupid}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "groupid" + "\\}", apiClient.escapeString(groupid.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupidPutValidateBeforeCall(String orgName, String appName, String authorization, String groupid, NewOwner body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsGroupidPut(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsGroupidPut(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsGroupidPut(Async)");
}
// verify the required parameter 'groupid' is set
if (groupid == null) {
throw new ApiException("Missing the required parameter 'groupid' when calling orgNameAppNameChatgroupsGroupidPut(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling orgNameAppNameChatgroupsGroupidPut(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupidPutCall(orgName, appName, authorization, groupid, body, progressListener, progressRequestListener);
return call;
}
/**
* Update Group Owner
* Transfer group ownership by changing owner.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupid (required)
* @param body Use the key \"newowner\" and ${new_owner_user} is the username of new group owner (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsGroupidPut(String orgName, String appName, String authorization, String groupid, NewOwner body) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsGroupidPutWithHttpInfo(orgName, appName, authorization, groupid, body);
return resp.getData();
}
/**
* Update Group Owner
* Transfer group ownership by changing owner.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupid (required)
* @param body Use the key \"newowner\" and ${new_owner_user} is the username of new group owner (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsGroupidPutWithHttpInfo(String orgName, String appName, String authorization, String groupid, NewOwner body) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupidPutValidateBeforeCall(orgName, appName, authorization, groupid, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update Group Owner (asynchronously)
* Transfer group ownership by changing owner.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param groupid (required)
* @param body Use the key \"newowner\" and ${new_owner_user} is the username of new group owner (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsGroupidPutAsync(String orgName, String appName, String authorization, String groupid, NewOwner body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsGroupidPutValidateBeforeCall(orgName, appName, authorization, groupid, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameChatgroupsPost */
private com.squareup.okhttp.Call orgNameAppNameChatgroupsPostCall(String orgName, String appName, String authorization, Group body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/chatgroups".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameChatgroupsPostValidateBeforeCall(String orgName, String appName, String authorization, Group body, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameChatgroupsPost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameChatgroupsPost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameChatgroupsPost(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling orgNameAppNameChatgroupsPost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsPostCall(orgName, appName, authorization, body, progressListener, progressRequestListener);
return call;
}
/**
* Create a Group
* Group settings: 1. Group name 2. Group description 3. Public vs. Private Group 4. Max number of member (including admin) 5. If public group, allow join freely vs. require permission from admin 6. allowinvites property. If public group, then allowinvites is false. If private group, then allowinvites is true.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param body \"desc\": group description. Note: Use key \"desc\". public vs. private: group type. maxusers: (Optional Attribute, default is 200). The max number of group members. approval: (Optional Attribute, default is true). Does user need permission to join the group? owner: The owner (admin) of the group. members: (Optional Attribute) Group members. Ignore the attribute if no member to be added. The group owner does not represent as a group member. (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameChatgroupsPost(String orgName, String appName, String authorization, Group body) throws ApiException {
ApiResponse resp = orgNameAppNameChatgroupsPostWithHttpInfo(orgName, appName, authorization, body);
return resp.getData();
}
/**
* Create a Group
* Group settings: 1. Group name 2. Group description 3. Public vs. Private Group 4. Max number of member (including admin) 5. If public group, allow join freely vs. require permission from admin 6. allowinvites property. If public group, then allowinvites is false. If private group, then allowinvites is true.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param body \"desc\": group description. Note: Use key \"desc\". public vs. private: group type. maxusers: (Optional Attribute, default is 200). The max number of group members. approval: (Optional Attribute, default is true). Does user need permission to join the group? owner: The owner (admin) of the group. members: (Optional Attribute) Group members. Ignore the attribute if no member to be added. The group owner does not represent as a group member. (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameChatgroupsPostWithHttpInfo(String orgName, String appName, String authorization, Group body) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsPostValidateBeforeCall(orgName, appName, authorization, body, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a Group (asynchronously)
* Group settings: 1. Group name 2. Group description 3. Public vs. Private Group 4. Max number of member (including admin) 5. If public group, allow join freely vs. require permission from admin 6. allowinvites property. If public group, then allowinvites is false. If private group, then allowinvites is true.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param body \"desc\": group description. Note: Use key \"desc\". public vs. private: group type. maxusers: (Optional Attribute, default is 200). The max number of group members. approval: (Optional Attribute, default is true). Does user need permission to join the group? owner: The owner (admin) of the group. members: (Optional Attribute) Group members. Ignore the attribute if no member to be added. The group owner does not represent as a group member. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameChatgroupsPostAsync(String orgName, String appName, String authorization, Group body, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameChatgroupsPostValidateBeforeCall(orgName, appName, authorization, body, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy