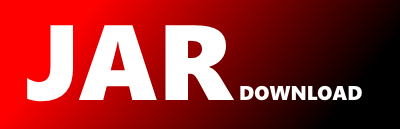
io.swagger.client.api.UsersApi Maven / Gradle / Ivy
The newest version!
/*
* Easemob REST APIs
* Easemob Server REST API Swagger is designated to provide better documentation and thorough interfaces for testing. For more details about server implementation, request rate limitation, etc, please visit [Easemob Server Integration](http://docs.easemob.com/im/100serverintegration/10intro). **Note:** `org_ID` is the unique ID of the organization created when you first registered [Easemob console](https://console.easemob.com/). `app_name` is the unique app ID created when you new application in [Easemob console](https://console.easemob.com/). `Authorization token` is required for most API requests as part of requesting header in the format `Bearer ${token}`. You can obtain the token via [/{org_name}/{app_name}/token](https://docs.hyphenate.io/docs/server-overview#section-request-authentication-token).
*
* OpenAPI spec version: 1.0.2
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package io.swagger.client.api;
import io.swagger.client.ApiCallback;
import io.swagger.client.ApiClient;
import io.swagger.client.ApiException;
import io.swagger.client.ApiResponse;
import io.swagger.client.Configuration;
import io.swagger.client.Pair;
import io.swagger.client.ProgressRequestBody;
import io.swagger.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.swagger.client.model.NewPassword;
import io.swagger.client.model.Nickname;
import io.swagger.client.model.RegisterUsers;
import io.swagger.client.model.UserNames;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class UsersApi {
private ApiClient apiClient;
public UsersApi() {
this(Configuration.getDefaultApiClient());
}
public UsersApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/* Build call for orgNameAppNameUsersDelete */
private com.squareup.okhttp.Call orgNameAppNameUsersDeleteCall(String orgName, String appName, String authorization, String limit, String cursor, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()));
List localVarQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "limit", limit));
if (cursor != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "cursor", cursor));
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersDeleteValidateBeforeCall(String orgName, String appName, String authorization, String limit, String cursor, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersDelete(Async)");
}
// verify the required parameter 'limit' is set
if (limit == null) {
throw new ApiException("Missing the required parameter 'limit' when calling orgNameAppNameUsersDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersDeleteCall(orgName, appName, authorization, limit, cursor, progressListener, progressRequestListener);
return call;
}
/**
* Delete Users in Batch
* Delete total number of N user accounts in batch. Recommend set N range 100~500 at a time to ensure the performance. Cannot specified user account in deletion, check the response to see which user accounts are deleted.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit Total number of user accounts to be deleted (required)
* @param cursor Cursor value from previous deletion (optional, default to )
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersDelete(String orgName, String appName, String authorization, String limit, String cursor) throws ApiException {
ApiResponse resp = orgNameAppNameUsersDeleteWithHttpInfo(orgName, appName, authorization, limit, cursor);
return resp.getData();
}
/**
* Delete Users in Batch
* Delete total number of N user accounts in batch. Recommend set N range 100~500 at a time to ensure the performance. Cannot specified user account in deletion, check the response to see which user accounts are deleted.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit Total number of user accounts to be deleted (required)
* @param cursor Cursor value from previous deletion (optional, default to )
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersDeleteWithHttpInfo(String orgName, String appName, String authorization, String limit, String cursor) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersDeleteValidateBeforeCall(orgName, appName, authorization, limit, cursor, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Delete Users in Batch (asynchronously)
* Delete total number of N user accounts in batch. Recommend set N range 100~500 at a time to ensure the performance. Cannot specified user account in deletion, check the response to see which user accounts are deleted.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit Total number of user accounts to be deleted (required)
* @param cursor Cursor value from previous deletion (optional, default to )
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersDeleteAsync(String orgName, String appName, String authorization, String limit, String cursor, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersDeleteValidateBeforeCall(orgName, appName, authorization, limit, cursor, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersGet */
private com.squareup.okhttp.Call orgNameAppNameUsersGetCall(String orgName, String appName, String authorization, String limit, String cursor, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()));
List localVarQueryParams = new ArrayList();
if (limit != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "limit", limit));
if (cursor != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "cursor", cursor));
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersGetValidateBeforeCall(String orgName, String appName, String authorization, String limit, String cursor, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersGetCall(orgName, appName, authorization, limit, cursor, progressListener, progressRequestListener);
return call;
}
/**
* Get Users in Batch
* Get a list of users
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit Get specified number of user by setting the batch limit with parameter \"limit\". Otherwise the API returns the 10 most recent created users by default. (optional, default to 3)
* @param cursor Pagination: If the query results more objects than value of limit, then the response will carry an extra attribute ¡°cursor¡±, the value points to the next page. There is No cursor on the last page or if the returning objects is less than batch limit value. (optional, default to LTgzNDAxMjM3OTpreS0yeXBSSkVlYWZZODl3bXppMTFn")
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersGet(String orgName, String appName, String authorization, String limit, String cursor) throws ApiException {
ApiResponse resp = orgNameAppNameUsersGetWithHttpInfo(orgName, appName, authorization, limit, cursor);
return resp.getData();
}
/**
* Get Users in Batch
* Get a list of users
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit Get specified number of user by setting the batch limit with parameter \"limit\". Otherwise the API returns the 10 most recent created users by default. (optional, default to 3)
* @param cursor Pagination: If the query results more objects than value of limit, then the response will carry an extra attribute ¡°cursor¡±, the value points to the next page. There is No cursor on the last page or if the returning objects is less than batch limit value. (optional, default to LTgzNDAxMjM3OTpreS0yeXBSSkVlYWZZODl3bXppMTFn")
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersGetWithHttpInfo(String orgName, String appName, String authorization, String limit, String cursor) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersGetValidateBeforeCall(orgName, appName, authorization, limit, cursor, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get Users in Batch (asynchronously)
* Get a list of users
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param limit Get specified number of user by setting the batch limit with parameter \"limit\". Otherwise the API returns the 10 most recent created users by default. (optional, default to 3)
* @param cursor Pagination: If the query results more objects than value of limit, then the response will carry an extra attribute ¡°cursor¡±, the value points to the next page. There is No cursor on the last page or if the returning objects is less than batch limit value. (optional, default to LTgzNDAxMjM3OTpreS0yeXBSSkVlYWZZODl3bXppMTFn")
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersGetAsync(String orgName, String appName, String authorization, String limit, String cursor, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersGetValidateBeforeCall(orgName, appName, authorization, limit, cursor, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDelete */
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDeleteCall(String orgName, String appName, String authorization, String ownerUsername, String blockedUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{owner_username}/blocks/users/{blocked_username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "owner_username" + "\\}", apiClient.escapeString(ownerUsername.toString()))
.replaceAll("\\{" + "blocked_username" + "\\}", apiClient.escapeString(blockedUsername.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDeleteValidateBeforeCall(String orgName, String appName, String authorization, String ownerUsername, String blockedUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDelete(Async)");
}
// verify the required parameter 'ownerUsername' is set
if (ownerUsername == null) {
throw new ApiException("Missing the required parameter 'ownerUsername' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDelete(Async)");
}
// verify the required parameter 'blockedUsername' is set
if (blockedUsername == null) {
throw new ApiException("Missing the required parameter 'blockedUsername' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDeleteCall(orgName, appName, authorization, ownerUsername, blockedUsername, progressListener, progressRequestListener);
return call;
}
/**
* Unblock User(s)
* Unblock one or multiple users by removing them from blacklist. Max 60 users at a time.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername (required)
* @param blockedUsername (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDelete(String orgName, String appName, String authorization, String ownerUsername, String blockedUsername) throws ApiException {
ApiResponse resp = orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDeleteWithHttpInfo(orgName, appName, authorization, ownerUsername, blockedUsername);
return resp.getData();
}
/**
* Unblock User(s)
* Unblock one or multiple users by removing them from blacklist. Max 60 users at a time.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername (required)
* @param blockedUsername (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDeleteWithHttpInfo(String orgName, String appName, String authorization, String ownerUsername, String blockedUsername) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDeleteValidateBeforeCall(orgName, appName, authorization, ownerUsername, blockedUsername, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Unblock User(s) (asynchronously)
* Unblock one or multiple users by removing them from blacklist. Max 60 users at a time.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername (required)
* @param blockedUsername (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDeleteAsync(String orgName, String appName, String authorization, String ownerUsername, String blockedUsername, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersBlockedUsernameDeleteValidateBeforeCall(orgName, appName, authorization, ownerUsername, blockedUsername, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersOwnerUsernameBlocksUsersGet */
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersGetCall(String orgName, String appName, String authorization, String ownerUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{owner_username}/blocks/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "owner_username" + "\\}", apiClient.escapeString(ownerUsername.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersGetValidateBeforeCall(String orgName, String appName, String authorization, String ownerUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersGet(Async)");
}
// verify the required parameter 'ownerUsername' is set
if (ownerUsername == null) {
throw new ApiException("Missing the required parameter 'ownerUsername' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersGetCall(orgName, appName, authorization, ownerUsername, progressListener, progressRequestListener);
return call;
}
/**
* Get a List of Blocked Users
* Get a List of Blocked Users by the user.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who is requesting blacklist (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersOwnerUsernameBlocksUsersGet(String orgName, String appName, String authorization, String ownerUsername) throws ApiException {
ApiResponse resp = orgNameAppNameUsersOwnerUsernameBlocksUsersGetWithHttpInfo(orgName, appName, authorization, ownerUsername);
return resp.getData();
}
/**
* Get a List of Blocked Users
* Get a List of Blocked Users by the user.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who is requesting blacklist (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersOwnerUsernameBlocksUsersGetWithHttpInfo(String orgName, String appName, String authorization, String ownerUsername) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersGetValidateBeforeCall(orgName, appName, authorization, ownerUsername, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a List of Blocked Users (asynchronously)
* Get a List of Blocked Users by the user.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who is requesting blacklist (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersGetAsync(String orgName, String appName, String authorization, String ownerUsername, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersGetValidateBeforeCall(orgName, appName, authorization, ownerUsername, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersOwnerUsernameBlocksUsersPost */
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersPostCall(String orgName, String appName, String authorization, String ownerUsername, UserNames usernames, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = usernames;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{owner_username}/blocks/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "owner_username" + "\\}", apiClient.escapeString(ownerUsername.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersPostValidateBeforeCall(String orgName, String appName, String authorization, String ownerUsername, UserNames usernames, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersPost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersPost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersPost(Async)");
}
// verify the required parameter 'ownerUsername' is set
if (ownerUsername == null) {
throw new ApiException("Missing the required parameter 'ownerUsername' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersPost(Async)");
}
// verify the required parameter 'usernames' is set
if (usernames == null) {
throw new ApiException("Missing the required parameter 'usernames' when calling orgNameAppNameUsersOwnerUsernameBlocksUsersPost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersPostCall(orgName, appName, authorization, ownerUsername, usernames, progressListener, progressRequestListener);
return call;
}
/**
* Block User(s)
* Block one or multiple users by adding to blacklist. Blocked user cannot send message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who initiated the blocking (required)
* @param usernames Users to be blocked. Use ',' to separate the usernames (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersOwnerUsernameBlocksUsersPost(String orgName, String appName, String authorization, String ownerUsername, UserNames usernames) throws ApiException {
ApiResponse resp = orgNameAppNameUsersOwnerUsernameBlocksUsersPostWithHttpInfo(orgName, appName, authorization, ownerUsername, usernames);
return resp.getData();
}
/**
* Block User(s)
* Block one or multiple users by adding to blacklist. Blocked user cannot send message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who initiated the blocking (required)
* @param usernames Users to be blocked. Use ',' to separate the usernames (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersOwnerUsernameBlocksUsersPostWithHttpInfo(String orgName, String appName, String authorization, String ownerUsername, UserNames usernames) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersPostValidateBeforeCall(orgName, appName, authorization, ownerUsername, usernames, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Block User(s) (asynchronously)
* Block one or multiple users by adding to blacklist. Blocked user cannot send message.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who initiated the blocking (required)
* @param usernames Users to be blocked. Use ',' to separate the usernames (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameBlocksUsersPostAsync(String orgName, String appName, String authorization, String ownerUsername, UserNames usernames, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameBlocksUsersPostValidateBeforeCall(orgName, appName, authorization, ownerUsername, usernames, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDelete */
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDeleteCall(String orgName, String appName, String authorization, String ownerUsername, String friendUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{owner_username}/contacts/users/{friend_username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "owner_username" + "\\}", apiClient.escapeString(ownerUsername.toString()))
.replaceAll("\\{" + "friend_username" + "\\}", apiClient.escapeString(friendUsername.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDeleteValidateBeforeCall(String orgName, String appName, String authorization, String ownerUsername, String friendUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDelete(Async)");
}
// verify the required parameter 'ownerUsername' is set
if (ownerUsername == null) {
throw new ApiException("Missing the required parameter 'ownerUsername' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDelete(Async)");
}
// verify the required parameter 'friendUsername' is set
if (friendUsername == null) {
throw new ApiException("Missing the required parameter 'friendUsername' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDeleteCall(orgName, appName, authorization, ownerUsername, friendUsername, progressListener, progressRequestListener);
return call;
}
/**
* Remove Contact from User
* Remove contact from user's contact list.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername user (required)
* @param friendUsername contact to be removed (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDelete(String orgName, String appName, String authorization, String ownerUsername, String friendUsername) throws ApiException {
ApiResponse resp = orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDeleteWithHttpInfo(orgName, appName, authorization, ownerUsername, friendUsername);
return resp.getData();
}
/**
* Remove Contact from User
* Remove contact from user's contact list.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername user (required)
* @param friendUsername contact to be removed (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDeleteWithHttpInfo(String orgName, String appName, String authorization, String ownerUsername, String friendUsername) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDeleteValidateBeforeCall(orgName, appName, authorization, ownerUsername, friendUsername, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Remove Contact from User (asynchronously)
* Remove contact from user's contact list.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername user (required)
* @param friendUsername contact to be removed (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDeleteAsync(String orgName, String appName, String authorization, String ownerUsername, String friendUsername, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernameDeleteValidateBeforeCall(orgName, appName, authorization, ownerUsername, friendUsername, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePost */
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePostCall(String orgName, String appName, String authorization, String ownerUsername, String friendUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{owner_username}/contacts/users/{friend_username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "owner_username" + "\\}", apiClient.escapeString(ownerUsername.toString()))
.replaceAll("\\{" + "friend_username" + "\\}", apiClient.escapeString(friendUsername.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePostValidateBeforeCall(String orgName, String appName, String authorization, String ownerUsername, String friendUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePost(Async)");
}
// verify the required parameter 'ownerUsername' is set
if (ownerUsername == null) {
throw new ApiException("Missing the required parameter 'ownerUsername' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePost(Async)");
}
// verify the required parameter 'friendUsername' is set
if (friendUsername == null) {
throw new ApiException("Missing the required parameter 'friendUsername' when calling orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePostCall(orgName, appName, authorization, ownerUsername, friendUsername, progressListener, progressRequestListener);
return call;
}
/**
* Add Contact for User
* Add contact for user. Contact to be added must under the same application as the user.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername user (required)
* @param friendUsername contact to be added (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePost(String orgName, String appName, String authorization, String ownerUsername, String friendUsername) throws ApiException {
ApiResponse resp = orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePostWithHttpInfo(orgName, appName, authorization, ownerUsername, friendUsername);
return resp.getData();
}
/**
* Add Contact for User
* Add contact for user. Contact to be added must under the same application as the user.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername user (required)
* @param friendUsername contact to be added (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePostWithHttpInfo(String orgName, String appName, String authorization, String ownerUsername, String friendUsername) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePostValidateBeforeCall(orgName, appName, authorization, ownerUsername, friendUsername, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Add Contact for User (asynchronously)
* Add contact for user. Contact to be added must under the same application as the user.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername user (required)
* @param friendUsername contact to be added (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePostAsync(String orgName, String appName, String authorization, String ownerUsername, String friendUsername, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersFriendUsernamePostValidateBeforeCall(orgName, appName, authorization, ownerUsername, friendUsername, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersOwnerUsernameContactsUsersGet */
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersGetCall(String orgName, String appName, String authorization, String ownerUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{owner_username}/contacts/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "owner_username" + "\\}", apiClient.escapeString(ownerUsername.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersGetValidateBeforeCall(String orgName, String appName, String authorization, String ownerUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersOwnerUsernameContactsUsersGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersOwnerUsernameContactsUsersGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersOwnerUsernameContactsUsersGet(Async)");
}
// verify the required parameter 'ownerUsername' is set
if (ownerUsername == null) {
throw new ApiException("Missing the required parameter 'ownerUsername' when calling orgNameAppNameUsersOwnerUsernameContactsUsersGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersGetCall(orgName, appName, authorization, ownerUsername, progressListener, progressRequestListener);
return call;
}
/**
* Get a List of Contacts
* Get user's contact list
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who is requesting contact list (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersOwnerUsernameContactsUsersGet(String orgName, String appName, String authorization, String ownerUsername) throws ApiException {
ApiResponse resp = orgNameAppNameUsersOwnerUsernameContactsUsersGetWithHttpInfo(orgName, appName, authorization, ownerUsername);
return resp.getData();
}
/**
* Get a List of Contacts
* Get user's contact list
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who is requesting contact list (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersOwnerUsernameContactsUsersGetWithHttpInfo(String orgName, String appName, String authorization, String ownerUsername) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersGetValidateBeforeCall(orgName, appName, authorization, ownerUsername, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a List of Contacts (asynchronously)
* Get user's contact list
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername The user who is requesting contact list (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameContactsUsersGetAsync(String orgName, String appName, String authorization, String ownerUsername, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameContactsUsersGetValidateBeforeCall(orgName, appName, authorization, ownerUsername, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersOwnerUsernameOfflineMsgCountGet */
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameOfflineMsgCountGetCall(String orgName, String appName, String authorization, String ownerUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{owner_username}/offline_msg_count".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "owner_username" + "\\}", apiClient.escapeString(ownerUsername.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameOfflineMsgCountGetValidateBeforeCall(String orgName, String appName, String authorization, String ownerUsername, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersOwnerUsernameOfflineMsgCountGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersOwnerUsernameOfflineMsgCountGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersOwnerUsernameOfflineMsgCountGet(Async)");
}
// verify the required parameter 'ownerUsername' is set
if (ownerUsername == null) {
throw new ApiException("Missing the required parameter 'ownerUsername' when calling orgNameAppNameUsersOwnerUsernameOfflineMsgCountGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameOfflineMsgCountGetCall(orgName, appName, authorization, ownerUsername, progressListener, progressRequestListener);
return call;
}
/**
* Get Offline Message Count
* Get the number of offline messages
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersOwnerUsernameOfflineMsgCountGet(String orgName, String appName, String authorization, String ownerUsername) throws ApiException {
ApiResponse resp = orgNameAppNameUsersOwnerUsernameOfflineMsgCountGetWithHttpInfo(orgName, appName, authorization, ownerUsername);
return resp.getData();
}
/**
* Get Offline Message Count
* Get the number of offline messages
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersOwnerUsernameOfflineMsgCountGetWithHttpInfo(String orgName, String appName, String authorization, String ownerUsername) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameOfflineMsgCountGetValidateBeforeCall(orgName, appName, authorization, ownerUsername, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get Offline Message Count (asynchronously)
* Get the number of offline messages
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param ownerUsername (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersOwnerUsernameOfflineMsgCountGetAsync(String orgName, String appName, String authorization, String ownerUsername, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersOwnerUsernameOfflineMsgCountGetValidateBeforeCall(orgName, appName, authorization, ownerUsername, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersPost */
private com.squareup.okhttp.Call orgNameAppNameUsersPostCall(String orgName, String appName, RegisterUsers body, String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersPostValidateBeforeCall(String orgName, String appName, RegisterUsers body, String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersPost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersPost(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling orgNameAppNameUsersPost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersPostCall(orgName, appName, body, authorization, progressListener, progressRequestListener);
return call;
}
/**
* Create a User
* Register an IM user account respects to org and app keys. Note: There're 2 types of registration, 'open' and 'authorized'. 'open' registration does not require admin authentication, but 'authorized' does. See the application -> \"overview\" -> \"User Registration Permission\" in Hyphenate console. 'Authorized' registration is recommended, which prevents malicious attempt to create junk user account from the ones obtained the URL.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param body To create multiple users at once: [ { \"username\": \"user1\", \"password\": \"123456\" }, { \"username\": \"user2\", \"password\": \"123456\" }] (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersPost(String orgName, String appName, RegisterUsers body, String authorization) throws ApiException {
ApiResponse resp = orgNameAppNameUsersPostWithHttpInfo(orgName, appName, body, authorization);
return resp.getData();
}
/**
* Create a User
* Register an IM user account respects to org and app keys. Note: There're 2 types of registration, 'open' and 'authorized'. 'open' registration does not require admin authentication, but 'authorized' does. See the application -> \"overview\" -> \"User Registration Permission\" in Hyphenate console. 'Authorized' registration is recommended, which prevents malicious attempt to create junk user account from the ones obtained the URL.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param body To create multiple users at once: [ { \"username\": \"user1\", \"password\": \"123456\" }, { \"username\": \"user2\", \"password\": \"123456\" }] (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersPostWithHttpInfo(String orgName, String appName, RegisterUsers body, String authorization) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersPostValidateBeforeCall(orgName, appName, body, authorization, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Create a User (asynchronously)
* Register an IM user account respects to org and app keys. Note: There're 2 types of registration, 'open' and 'authorized'. 'open' registration does not require admin authentication, but 'authorized' does. See the application -> \"overview\" -> \"User Registration Permission\" in Hyphenate console. 'Authorized' registration is recommended, which prevents malicious attempt to create junk user account from the ones obtained the URL.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param body To create multiple users at once: [ { \"username\": \"user1\", \"password\": \"123456\" }, { \"username\": \"user2\", \"password\": \"123456\" }] (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersPostAsync(String orgName, String appName, RegisterUsers body, String authorization, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersPostValidateBeforeCall(orgName, appName, body, authorization, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameActivatePost */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameActivatePostCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}/activate".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameActivatePostValidateBeforeCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameActivatePost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameActivatePost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameActivatePost(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameActivatePost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameActivatePostCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
return call;
}
/**
* Activate User Account
* Activate a deactivated user account.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameActivatePost(String orgName, String appName, String authorization, String username) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameActivatePostWithHttpInfo(orgName, appName, authorization, username);
return resp.getData();
}
/**
* Activate User Account
* Activate a deactivated user account.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameActivatePostWithHttpInfo(String orgName, String appName, String authorization, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameActivatePostValidateBeforeCall(orgName, appName, authorization, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Activate User Account (asynchronously)
* Activate a deactivated user account.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameActivatePostAsync(String orgName, String appName, String authorization, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameActivatePostValidateBeforeCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameDeactivatePost */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameDeactivatePostCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}/deactivate".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameDeactivatePostValidateBeforeCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameDeactivatePost(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameDeactivatePost(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameDeactivatePost(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameDeactivatePost(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDeactivatePostCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
return call;
}
/**
* Deactivate User Account
* Deactivate a user account. User will not be able to login.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameDeactivatePost(String orgName, String appName, String authorization, String username) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameDeactivatePostWithHttpInfo(orgName, appName, authorization, username);
return resp.getData();
}
/**
* Deactivate User Account
* Deactivate a user account. User will not be able to login.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameDeactivatePostWithHttpInfo(String orgName, String appName, String authorization, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDeactivatePostValidateBeforeCall(orgName, appName, authorization, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Deactivate User Account (asynchronously)
* Deactivate a user account. User will not be able to login.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameDeactivatePostAsync(String orgName, String appName, String authorization, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDeactivatePostValidateBeforeCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameDelete */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameDeleteCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameDeleteValidateBeforeCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameDelete(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameDelete(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameDelete(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameDelete(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDeleteCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
return call;
}
/**
* Delete a User
* Warning: Delete a user will also delete the group and chat room if the specified user is the admin of the group and chat room.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username user to be deleted (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameDelete(String orgName, String appName, String authorization, String username) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameDeleteWithHttpInfo(orgName, appName, authorization, username);
return resp.getData();
}
/**
* Delete a User
* Warning: Delete a user will also delete the group and chat room if the specified user is the admin of the group and chat room.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username user to be deleted (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameDeleteWithHttpInfo(String orgName, String appName, String authorization, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDeleteValidateBeforeCall(orgName, appName, authorization, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Delete a User (asynchronously)
* Warning: Delete a user will also delete the group and chat room if the specified user is the admin of the group and chat room.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username user to be deleted (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameDeleteAsync(String orgName, String appName, String authorization, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDeleteValidateBeforeCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameDisconnectGet */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameDisconnectGetCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}/disconnect".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameDisconnectGetValidateBeforeCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameDisconnectGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameDisconnectGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameDisconnectGet(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameDisconnectGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDisconnectGetCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
return call;
}
/**
* Logout User
* Force logout a user
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameDisconnectGet(String orgName, String appName, String authorization, String username) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameDisconnectGetWithHttpInfo(orgName, appName, authorization, username);
return resp.getData();
}
/**
* Logout User
* Force logout a user
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameDisconnectGetWithHttpInfo(String orgName, String appName, String authorization, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDisconnectGetValidateBeforeCall(orgName, appName, authorization, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Logout User (asynchronously)
* Force logout a user
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameDisconnectGetAsync(String orgName, String appName, String authorization, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameDisconnectGetValidateBeforeCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameGet */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameGetCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameGetValidateBeforeCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameGet(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameGetCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
return call;
}
/**
* Get a User
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameGet(String orgName, String appName, String authorization, String username) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameGetWithHttpInfo(orgName, appName, authorization, username);
return resp.getData();
}
/**
* Get a User
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameGetWithHttpInfo(String orgName, String appName, String authorization, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameGetValidateBeforeCall(orgName, appName, authorization, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a User (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameGetAsync(String orgName, String appName, String authorization, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameGetValidateBeforeCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameJoinedChatgroupsGet */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameJoinedChatgroupsGetCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}/joined_chatgroups".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameJoinedChatgroupsGetValidateBeforeCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameJoinedChatgroupsGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameJoinedChatgroupsGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameJoinedChatgroupsGet(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameJoinedChatgroupsGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameJoinedChatgroupsGetCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
return call;
}
/**
* Get a List of Groups of User Joined
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameJoinedChatgroupsGet(String orgName, String appName, String authorization, String username) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameJoinedChatgroupsGetWithHttpInfo(orgName, appName, authorization, username);
return resp.getData();
}
/**
* Get a List of Groups of User Joined
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameJoinedChatgroupsGetWithHttpInfo(String orgName, String appName, String authorization, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameJoinedChatgroupsGetValidateBeforeCall(orgName, appName, authorization, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get a List of Groups of User Joined (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameJoinedChatgroupsGetAsync(String orgName, String appName, String authorization, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameJoinedChatgroupsGetValidateBeforeCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameJoinedChatroomsGet */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameJoinedChatroomsGetCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}/joined_chatrooms".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameJoinedChatroomsGetValidateBeforeCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameJoinedChatroomsGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameJoinedChatroomsGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameJoinedChatroomsGet(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameJoinedChatroomsGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameJoinedChatroomsGetCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
return call;
}
/**
* Get All the Chat Rooms of User Joined
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameJoinedChatroomsGet(String orgName, String appName, String authorization, String username) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameJoinedChatroomsGetWithHttpInfo(orgName, appName, authorization, username);
return resp.getData();
}
/**
* Get All the Chat Rooms of User Joined
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameJoinedChatroomsGetWithHttpInfo(String orgName, String appName, String authorization, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameJoinedChatroomsGetValidateBeforeCall(orgName, appName, authorization, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get All the Chat Rooms of User Joined (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameJoinedChatroomsGetAsync(String orgName, String appName, String authorization, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameJoinedChatroomsGetValidateBeforeCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGet */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGetCall(String orgName, String appName, String authorization, String username, String msgId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}/offline_msg_status/{msg_id}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()))
.replaceAll("\\{" + "msg_id" + "\\}", apiClient.escapeString(msgId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put(" Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGetValidateBeforeCall(String orgName, String appName, String authorization, String username, String msgId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGet(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGet(Async)");
}
// verify the required parameter 'msgId' is set
if (msgId == null) {
throw new ApiException("Missing the required parameter 'msgId' when calling orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGetCall(orgName, appName, authorization, username, msgId, progressListener, progressRequestListener);
return call;
}
/**
* Get Offline Message Status
* Get offline message status via message ID. Get message ID via the method get message history.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param msgId Message ID (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGet(String orgName, String appName, String authorization, String username, String msgId) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGetWithHttpInfo(orgName, appName, authorization, username, msgId);
return resp.getData();
}
/**
* Get Offline Message Status
* Get offline message status via message ID. Get message ID via the method get message history.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param msgId Message ID (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGetWithHttpInfo(String orgName, String appName, String authorization, String username, String msgId) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGetValidateBeforeCall(orgName, appName, authorization, username, msgId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get Offline Message Status (asynchronously)
* Get offline message status via message ID. Get message ID via the method get message history.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param msgId Message ID (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGetAsync(String orgName, String appName, String authorization, String username, String msgId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameOfflineMsgStatusMsgIdGetValidateBeforeCall(orgName, appName, authorization, username, msgId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernamePasswordPut */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernamePasswordPutCall(String orgName, String appName, String username, NewPassword body, String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}/password".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernamePasswordPutValidateBeforeCall(String orgName, String appName, String username, NewPassword body, String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernamePasswordPut(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernamePasswordPut(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernamePasswordPut(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling orgNameAppNameUsersUsernamePasswordPut(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernamePasswordPutCall(orgName, appName, username, body, authorization, progressListener, progressRequestListener);
return call;
}
/**
* Reset User's Password
* To enhance security, we recommend update user's Hyphenate IM user account password if the user's app password on developer server is updated.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param username (required)
* @param body Set a new password by using key \"newpassword\" (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernamePasswordPut(String orgName, String appName, String username, NewPassword body, String authorization) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernamePasswordPutWithHttpInfo(orgName, appName, username, body, authorization);
return resp.getData();
}
/**
* Reset User's Password
* To enhance security, we recommend update user's Hyphenate IM user account password if the user's app password on developer server is updated.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param username (required)
* @param body Set a new password by using key \"newpassword\" (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernamePasswordPutWithHttpInfo(String orgName, String appName, String username, NewPassword body, String authorization) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernamePasswordPutValidateBeforeCall(orgName, appName, username, body, authorization, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Reset User's Password (asynchronously)
* To enhance security, we recommend update user's Hyphenate IM user account password if the user's app password on developer server is updated.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param username (required)
* @param body Set a new password by using key \"newpassword\" (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernamePasswordPutAsync(String orgName, String appName, String username, NewPassword body, String authorization, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernamePasswordPutValidateBeforeCall(orgName, appName, username, body, authorization, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernamePut */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernamePutCall(String orgName, String appName, String username, Nickname body, String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = body;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernamePutValidateBeforeCall(String orgName, String appName, String username, Nickname body, String authorization, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernamePut(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernamePut(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernamePut(Async)");
}
// verify the required parameter 'body' is set
if (body == null) {
throw new ApiException("Missing the required parameter 'body' when calling orgNameAppNameUsersUsernamePut(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernamePutCall(orgName, appName, username, body, authorization, progressListener, progressRequestListener);
return call;
}
/**
* Update User's APNs Display Name
* Update user's APNs display name for iOS push notification.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param username (required)
* @param body update APNs display name by key \"nickname\" (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernamePut(String orgName, String appName, String username, Nickname body, String authorization) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernamePutWithHttpInfo(orgName, appName, username, body, authorization);
return resp.getData();
}
/**
* Update User's APNs Display Name
* Update user's APNs display name for iOS push notification.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param username (required)
* @param body update APNs display name by key \"nickname\" (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernamePutWithHttpInfo(String orgName, String appName, String username, Nickname body, String authorization) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernamePutValidateBeforeCall(orgName, appName, username, body, authorization, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Update User's APNs Display Name (asynchronously)
* Update user's APNs display name for iOS push notification.
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param username (required)
* @param body update APNs display name by key \"nickname\" (required)
* @param authorization Bearer ${token} (optional, default to Bearer YWMtLU9T4JRGEea0-Vvai3EzjAAAAVkGz4dZKNSpsVdRvVix2OfSm42w5-IaUL4)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernamePutAsync(String orgName, String appName, String username, Nickname body, String authorization, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernamePutValidateBeforeCall(orgName, appName, username, body, authorization, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for orgNameAppNameUsersUsernameStatusGet */
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameStatusGetCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{org_name}/{app_name}/users/{username}/status".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "org_name" + "\\}", apiClient.escapeString(orgName.toString()))
.replaceAll("\\{" + "app_name" + "\\}", apiClient.escapeString(appName.toString()))
.replaceAll("\\{" + "username" + "\\}", apiClient.escapeString(username.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (authorization != null)
localVarHeaderParams.put("Authorization", apiClient.parameterToString(authorization));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call orgNameAppNameUsersUsernameStatusGetValidateBeforeCall(String orgName, String appName, String authorization, String username, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'orgName' is set
if (orgName == null) {
throw new ApiException("Missing the required parameter 'orgName' when calling orgNameAppNameUsersUsernameStatusGet(Async)");
}
// verify the required parameter 'appName' is set
if (appName == null) {
throw new ApiException("Missing the required parameter 'appName' when calling orgNameAppNameUsersUsernameStatusGet(Async)");
}
// verify the required parameter 'authorization' is set
if (authorization == null) {
throw new ApiException("Missing the required parameter 'authorization' when calling orgNameAppNameUsersUsernameStatusGet(Async)");
}
// verify the required parameter 'username' is set
if (username == null) {
throw new ApiException("Missing the required parameter 'username' when calling orgNameAppNameUsersUsernameStatusGet(Async)");
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameStatusGetCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
return call;
}
/**
* Get User Online Status
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String orgNameAppNameUsersUsernameStatusGet(String orgName, String appName, String authorization, String username) throws ApiException {
ApiResponse resp = orgNameAppNameUsersUsernameStatusGetWithHttpInfo(orgName, appName, authorization, username);
return resp.getData();
}
/**
* Get User Online Status
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse orgNameAppNameUsersUsernameStatusGetWithHttpInfo(String orgName, String appName, String authorization, String username) throws ApiException {
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameStatusGetValidateBeforeCall(orgName, appName, authorization, username, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Get User Online Status (asynchronously)
*
* @param orgName Organization ID (required)
* @param appName Application name (required)
* @param authorization Bearer ${token} (required)
* @param username (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call orgNameAppNameUsersUsernameStatusGetAsync(String orgName, String appName, String authorization, String username, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = orgNameAppNameUsersUsernameStatusGetValidateBeforeCall(orgName, appName, authorization, username, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy