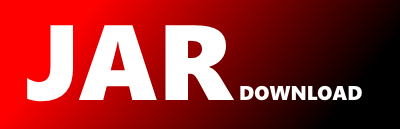
com.easy.query.api.proxy.entity.update.abstraction.AbstractExpressionUpdatable Maven / Gradle / Ivy
package com.easy.query.api.proxy.entity.update.abstraction;
import com.easy.query.api.proxy.entity.update.ExpressionUpdatable;
import com.easy.query.core.basic.api.update.ClientExpressionUpdatable;
import com.easy.query.core.expression.lambda.SQLExpression1;
import com.easy.query.core.expression.sql.builder.EntityUpdateExpressionBuilder;
import com.easy.query.core.expression.sql.builder.internal.ContextConfigurer;
import com.easy.query.core.expression.sql.builder.internal.EasyBehavior;
import com.easy.query.core.proxy.ProxyEntity;
import java.util.function.BiFunction;
import java.util.function.Function;
/**
* @author xuejiaming
* @FileName: AbstractExpressionUpdate.java
* @Description: 文件说明
* @Date: 2023/2/25 08:24
*/
public abstract class AbstractExpressionUpdatable, T> implements ExpressionUpdatable {
private final TProxy tProxy;
protected final ClientExpressionUpdatable clientExpressionUpdatable;
public AbstractExpressionUpdatable(TProxy tProxy,ClientExpressionUpdatable clientExpressionUpdatable) {
this.clientExpressionUpdatable = clientExpressionUpdatable;
this.tProxy = tProxy.create(clientExpressionUpdatable.getUpdateExpressionBuilder().getTable(0).getEntityTable(),clientExpressionUpdatable.getUpdateExpressionBuilder(), getExpressionContext().getRuntimeContext());
}
@Override
public EntityUpdateExpressionBuilder getUpdateExpressionBuilder() {
return clientExpressionUpdatable.getUpdateExpressionBuilder();
}
@Override
public TProxy getProxy() {
return tProxy;
}
@Override
public ClientExpressionUpdatable getClientUpdate() {
return clientExpressionUpdatable;
}
@Override
public long executeRows() {
return clientExpressionUpdatable.executeRows();
}
@Override
public ExpressionUpdatable withVersion(boolean condition, Object versionValue) {
if (condition) {
clientExpressionUpdatable.withVersion(versionValue);
}
return this;
}
@Override
public ExpressionUpdatable asTable(Function tableNameAs) {
clientExpressionUpdatable.asTable(tableNameAs);
return this;
}
@Override
public ExpressionUpdatable asSchema(Function schemaAs) {
clientExpressionUpdatable.asSchema(schemaAs);
return this;
}
@Override
public ExpressionUpdatable asAlias(String alias) {
clientExpressionUpdatable.asAlias(alias);
return this;
}
@Override
public ExpressionUpdatable noInterceptor() {
clientExpressionUpdatable.noInterceptor();
return this;
}
@Override
public ExpressionUpdatable useInterceptor(String name) {
clientExpressionUpdatable.useInterceptor(name);
return this;
}
@Override
public ExpressionUpdatable noInterceptor(String name) {
clientExpressionUpdatable.noInterceptor(name);
return this;
}
@Override
public ExpressionUpdatable useInterceptor() {
clientExpressionUpdatable.useInterceptor();
return this;
}
@Override
public ExpressionUpdatable useLogicDelete(boolean enable) {
clientExpressionUpdatable.useLogicDelete(enable);
return this;
}
@Override
public ExpressionUpdatable configure(SQLExpression1 configurer) {
clientExpressionUpdatable.configure(configurer);
return this;
}
@Override
public void executeRows(long expectRows, String msg, String code) {
clientExpressionUpdatable.executeRows(expectRows, msg, code);
}
@Override
public ExpressionUpdatable ignoreVersion(boolean ignored) {
clientExpressionUpdatable.ignoreVersion(ignored);
return this;
}
@Override
public ExpressionUpdatable batch(boolean use) {
clientExpressionUpdatable.batch(use);
return this;
}
@Override
public ExpressionUpdatable asTableLink(Function linkAs) {
clientExpressionUpdatable.asTableLink(linkAs);
return this;
}
@Override
public ExpressionUpdatable asTableSegment(BiFunction segmentAs) {
clientExpressionUpdatable.asTableSegment(segmentAs);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy