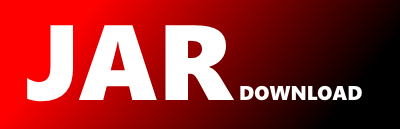
com.easy.query.api4j.update.ExpressionUpdatable Maven / Gradle / Ivy
package com.easy.query.api4j.update;
import com.easy.query.api4j.sql.SQLWherePredicate;
import com.easy.query.api4j.sql.impl.SQLWherePredicateImpl;
import com.easy.query.api4j.sql.scec.SQLNativeLambdaExpressionContext;
import com.easy.query.api4j.sql.scec.SQLNativeLambdaExpressionContextImpl;
import com.easy.query.api4j.util.EasyLambdaUtil;
import com.easy.query.core.basic.api.internal.ConfigureVersionable;
import com.easy.query.core.basic.api.internal.WithVersionable;
import com.easy.query.core.basic.api.update.ClientExpressionUpdatable;
import com.easy.query.core.basic.api.update.Updatable;
import com.easy.query.core.basic.jdbc.parameter.ToSQLContext;
import com.easy.query.core.expression.lambda.Property;
import com.easy.query.core.expression.lambda.SQLExpression1;
import com.easy.query.core.expression.sql.builder.ExpressionContext;
import java.util.Collection;
/**
* @author xuejiaming
* @FileName: ExpressionUpdatable.java
* @Description: 文件说明
* @Date: 2023/2/24 23:21
*/
public interface ExpressionUpdatable extends Updatable>, WithVersionable>, ConfigureVersionable> {
ClientExpressionUpdatable getClientUpdate();
default ExpressionUpdatable set(Property column, Object val) {
getClientUpdate().set(EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable set(boolean condition, Property column, Object val) {
getClientUpdate().set(condition, EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setWithColumn(Property column1, Property column2) {
getClientUpdate().setWithColumn(EasyLambdaUtil.getPropertyName(column1), EasyLambdaUtil.getPropertyName(column2));
return this;
}
default ExpressionUpdatable setWithColumn(boolean condition, Property column1, Property column2) {
getClientUpdate().setWithColumn(condition, EasyLambdaUtil.getPropertyName(column1), EasyLambdaUtil.getPropertyName(column2));
return this;
}
// region 列++ --
default ExpressionUpdatable setIncrement(Property column) {
getClientUpdate().setIncrement(EasyLambdaUtil.getPropertyName(column));
return this;
}
default ExpressionUpdatable setIncrement(boolean condition, Property column) {
getClientUpdate().setIncrement(condition, EasyLambdaUtil.getPropertyName(column));
return this;
}
default ExpressionUpdatable setIncrement(Property column, int val) {
getClientUpdate().setIncrement(EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setIncrement(boolean condition, Property column, int val) {
getClientUpdate().setIncrement(condition, EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setIncrement(Property column, long val) {
getClientUpdate().setIncrement(EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setIncrement(boolean condition, Property column, long val) {
getClientUpdate().setIncrement(condition, EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setIncrement(Property column, Number val) {
getClientUpdate().setIncrement(EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setIncrementNumber(boolean condition, Property column, Number val) {
getClientUpdate().setIncrementNumber(condition, EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setDecrement(Property column) {
getClientUpdate().setDecrement(EasyLambdaUtil.getPropertyName(column));
return this;
}
default ExpressionUpdatable setDecrement(boolean condition, Property column) {
getClientUpdate().setDecrement(condition, EasyLambdaUtil.getPropertyName(column));
return this;
}
default ExpressionUpdatable setDecrement(Property column, int val) {
getClientUpdate().setDecrement(EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setDecrement(boolean condition, Property column, int val) {
getClientUpdate().setDecrement(condition, EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setDecrement(Property column, long val) {
getClientUpdate().setDecrement(EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setDecrement(boolean condition, Property column, long val) {
getClientUpdate().setDecrement(condition, EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setDecrement(Property column, Number val) {
getClientUpdate().setDecrement(EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setDecrementNumber(boolean condition, Property column, Number val) {
getClientUpdate().setDecrementNumber(condition, EasyLambdaUtil.getPropertyName(column), val);
return this;
}
default ExpressionUpdatable setSQLSegment(Property property, String sqlSegment, SQLExpression1> contextConsume) {
return setSQLSegment(true, property, sqlSegment, contextConsume);
}
default ExpressionUpdatable setSQLSegment(boolean condition, Property property, String sqlSegment, SQLExpression1> contextConsume) {
if (condition) {
getClientUpdate().setSQLSegment(EasyLambdaUtil.getPropertyName(property), sqlSegment, (context) -> {
contextConsume.apply(new SQLNativeLambdaExpressionContextImpl<>(context));
});
}
return this;
}
// endregion
default ExpressionUpdatable where(SQLExpression1> whereExpression) {
getClientUpdate().where(where -> {
whereExpression.apply(new SQLWherePredicateImpl<>(where));
});
return this;
}
default ExpressionUpdatable where(boolean condition, SQLExpression1> whereExpression) {
getClientUpdate().where(condition, where -> {
whereExpression.apply(new SQLWherePredicateImpl<>(where));
});
return this;
}
default ExpressionUpdatable whereById(Object id) {
getClientUpdate().whereById(id);
return this;
}
default ExpressionUpdatable whereById(boolean condition, Object id) {
getClientUpdate().whereById(condition, id);
return this;
}
default ExpressionUpdatable whereByIds(Collection ids) {
getClientUpdate().whereByIds(ids);
return this;
}
default ExpressionUpdatable whereByIds(boolean condition, Collection ids) {
getClientUpdate().whereByIds(condition, ids);
return this;
}
default ExpressionContext getExpressionContext() {
return getClientUpdate().getExpressionContext();
}
default String toSQL() {
return getClientUpdate().toSQL();
}
default String toSQL(ToSQLContext toSQLContext) {
return getClientUpdate().toSQL(toSQLContext);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy