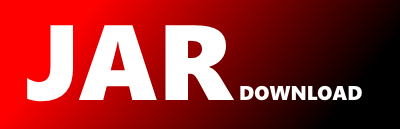
com.easy.query.api4j.select.extension.queryable7.SQLJoinable7 Maven / Gradle / Ivy
package com.easy.query.api4j.select.extension.queryable7;
import com.easy.query.api4j.select.Queryable;
import com.easy.query.api4j.select.Queryable8;
import com.easy.query.api4j.select.impl.EasyQueryable8;
import com.easy.query.api4j.sql.SQLWherePredicate;
import com.easy.query.api4j.sql.impl.SQLWherePredicateImpl;
import com.easy.query.core.basic.api.select.ClientQueryable8;
import com.easy.query.core.common.tuple.Tuple8;
import com.easy.query.core.expression.lambda.SQLExpression1;
import com.easy.query.core.expression.lambda.SQLExpression8;
/**
* create time 2023/8/18 13:14
* 文件说明
*
* @author xuejiaming
*/
public interface SQLJoinable7 extends ClientQueryable7Available {
default Queryable8 leftJoin(Class joinClass, SQLExpression8, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate> on) {
ClientQueryable8 entityQueryable7 = getClientQueryable7().leftJoin(joinClass, (where1, where2, where3, where4, where5, where6, where7, where8) -> {
on.apply(new SQLWherePredicateImpl<>(where1), new SQLWherePredicateImpl<>(where2), new SQLWherePredicateImpl<>(where3), new SQLWherePredicateImpl<>(where4), new SQLWherePredicateImpl<>(where5), new SQLWherePredicateImpl<>(where6), new SQLWherePredicateImpl<>(where7), new SQLWherePredicateImpl<>(where8));
});
return new EasyQueryable8<>(entityQueryable7);
}
default Queryable8 leftJoin(Queryable joinQueryable, SQLExpression8, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate> on) {
ClientQueryable8 entityQueryable7 = getClientQueryable7().leftJoin(joinQueryable.getClientQueryable(), (where1, where2, where3, where4, where5, where6, where7, where8) -> {
on.apply(new SQLWherePredicateImpl<>(where1), new SQLWherePredicateImpl<>(where2), new SQLWherePredicateImpl<>(where3), new SQLWherePredicateImpl<>(where4), new SQLWherePredicateImpl<>(where5), new SQLWherePredicateImpl<>(where6), new SQLWherePredicateImpl<>(where7), new SQLWherePredicateImpl<>(where8));
});
return new EasyQueryable8<>(entityQueryable7);
}
default Queryable8 rightJoin(Class joinClass, SQLExpression8, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate> on) {
ClientQueryable8 entityQueryable7 = getClientQueryable7().rightJoin(joinClass, (where1, where2, where3, where4, where5, where6, where7, where8) -> {
on.apply(new SQLWherePredicateImpl<>(where1), new SQLWherePredicateImpl<>(where2), new SQLWherePredicateImpl<>(where3), new SQLWherePredicateImpl<>(where4), new SQLWherePredicateImpl<>(where5), new SQLWherePredicateImpl<>(where6), new SQLWherePredicateImpl<>(where7), new SQLWherePredicateImpl<>(where8));
});
return new EasyQueryable8<>(entityQueryable7);
}
default Queryable8 rightJoin(Queryable joinQueryable, SQLExpression8, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate> on) {
ClientQueryable8 entityQueryable7 = getClientQueryable7().rightJoin(joinQueryable.getClientQueryable(), (where1, where2, where3, where4, where5, where6, where7, where8) -> {
on.apply(new SQLWherePredicateImpl<>(where1), new SQLWherePredicateImpl<>(where2), new SQLWherePredicateImpl<>(where3), new SQLWherePredicateImpl<>(where4), new SQLWherePredicateImpl<>(where5), new SQLWherePredicateImpl<>(where6), new SQLWherePredicateImpl<>(where7), new SQLWherePredicateImpl<>(where8));
});
return new EasyQueryable8<>(entityQueryable7);
}
default Queryable8 innerJoin(Class joinClass, SQLExpression8, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate> on) {
ClientQueryable8 entityQueryable7 = getClientQueryable7().innerJoin(joinClass, (where1, where2, where3, where4, where5, where6, where7, where8) -> {
on.apply(new SQLWherePredicateImpl<>(where1), new SQLWherePredicateImpl<>(where2), new SQLWherePredicateImpl<>(where3), new SQLWherePredicateImpl<>(where4), new SQLWherePredicateImpl<>(where5), new SQLWherePredicateImpl<>(where6), new SQLWherePredicateImpl<>(where7), new SQLWherePredicateImpl<>(where8));
});
return new EasyQueryable8<>(entityQueryable7);
}
default Queryable8 innerJoin(Queryable joinQueryable, SQLExpression8, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate> on) {
ClientQueryable8 entityQueryable7 = getClientQueryable7().innerJoin(joinQueryable.getClientQueryable(), (where1, where2, where3, where4, where5, where6, where7, where8) -> {
on.apply(new SQLWherePredicateImpl<>(where1), new SQLWherePredicateImpl<>(where2), new SQLWherePredicateImpl<>(where3), new SQLWherePredicateImpl<>(where4), new SQLWherePredicateImpl<>(where5), new SQLWherePredicateImpl<>(where6), new SQLWherePredicateImpl<>(where7), new SQLWherePredicateImpl<>(where8));
});
return new EasyQueryable8<>(entityQueryable7);
}
default Queryable8 leftJoinMerge(Class joinClass, SQLExpression1, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate>> on) {
return leftJoin(joinClass, (t1, t2, t3, t4, t5, t6, t7, t8) -> {
on.apply(new Tuple8<>(t1, t2, t3, t4, t5, t6, t7, t8));
});
}
default Queryable8 leftJoinMerge(Queryable joinQueryable, SQLExpression1, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate>> on) {
return leftJoin(joinQueryable, (t1, t2, t3, t4, t5, t6, t7, t8) -> {
on.apply(new Tuple8<>(t1, t2, t3, t4, t5, t6, t7, t8));
});
}
default Queryable8 rightJoinMerge(Class joinClass, SQLExpression1, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate>> on) {
return rightJoin(joinClass, (t1, t2, t3, t4, t5, t6, t7, t8) -> {
on.apply(new Tuple8<>(t1, t2, t3, t4, t5, t6, t7, t8));
});
}
default Queryable8 rightJoinMerge(Queryable joinQueryable, SQLExpression1, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate>> on) {
return rightJoin(joinQueryable, (t1, t2, t3, t4, t5, t6, t7, t8) -> {
on.apply(new Tuple8<>(t1, t2, t3, t4, t5, t6, t7, t8));
});
}
default Queryable8 innerJoinMerge(Class joinClass, SQLExpression1, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate>> on) {
return innerJoin(joinClass, (t1, t2, t3, t4, t5, t6, t7, t8) -> {
on.apply(new Tuple8<>(t1, t2, t3, t4, t5, t6, t7, t8));
});
}
default Queryable8 innerJoinMerge(Queryable joinQueryable, SQLExpression1, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate, SQLWherePredicate>> on) {
return innerJoin(joinQueryable, (t1, t2, t3, t4, t5, t6, t7, t8) -> {
on.apply(new Tuple8<>(t1, t2, t3, t4, t5, t6, t7, t8));
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy