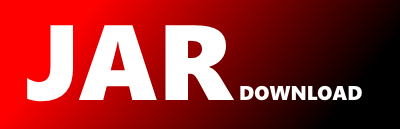
com.easy.query.api4j.sql.SQLWhereAggregatePredicate Maven / Gradle / Ivy
package com.easy.query.api4j.sql;
import com.easy.query.api4j.sql.core.SQLLambdaNative;
import com.easy.query.api4j.sql.core.available.LambdaSQLFuncAvailable;
import com.easy.query.api4j.util.EasyLambdaUtil;
import com.easy.query.core.context.QueryRuntimeContext;
import com.easy.query.core.enums.AggregatePredicateCompare;
import com.easy.query.core.enums.SQLPredicateCompare;
import com.easy.query.core.expression.func.ColumnFunction;
import com.easy.query.core.expression.lambda.Property;
import com.easy.query.core.expression.lambda.SQLExpression1;
import com.easy.query.core.expression.parser.core.EntitySQLTableOwner;
import com.easy.query.core.expression.parser.core.available.TableAvailable;
import com.easy.query.core.expression.parser.core.base.WhereAggregatePredicate;
/**
* @author xuejiaming
* @FileName: AggregatePredicate.java
* @Description: 文件说明
* @Date: 2023/2/18 22:17
*/
public interface SQLWhereAggregatePredicate extends EntitySQLTableOwner, LambdaSQLFuncAvailable, SQLLambdaNative> {
WhereAggregatePredicate getWhereAggregatePredicate();
default TableAvailable getTable() {
return getWhereAggregatePredicate().getTable();
}
default QueryRuntimeContext getRuntimeContext() {
return getWhereAggregatePredicate().getRuntimeContext();
}
default SQLWhereAggregatePredicate avg(Property column, AggregatePredicateCompare compare, TProperty val) {
return avg(true, column, compare, val);
}
default SQLWhereAggregatePredicate avg(boolean condition, Property column, AggregatePredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().avg(condition, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default SQLWhereAggregatePredicate avgDistinct(Property column, AggregatePredicateCompare compare, TProperty val) {
return avgDistinct(true, column, compare, val);
}
default SQLWhereAggregatePredicate avgDistinct(boolean condition, Property column, AggregatePredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().avgDistinct(condition, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default SQLWhereAggregatePredicate min(Property column, AggregatePredicateCompare compare, TProperty val) {
return min(true, column, compare, val);
}
default SQLWhereAggregatePredicate min(boolean condition, Property column, AggregatePredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().min(condition, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default SQLWhereAggregatePredicate max(Property column, AggregatePredicateCompare compare, TProperty val) {
return max(true, column, compare, val);
}
default SQLWhereAggregatePredicate max(boolean condition, Property column, AggregatePredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().max(condition, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default SQLWhereAggregatePredicate sum(Property column, AggregatePredicateCompare compare, TProperty val) {
return sum(true, column, compare, val);
}
default SQLWhereAggregatePredicate sum(boolean condition, Property column, AggregatePredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().sum(condition, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default SQLWhereAggregatePredicate sumDistinct(Property column, AggregatePredicateCompare compare, TProperty val) {
return sumDistinct(true, column, compare, val);
}
default SQLWhereAggregatePredicate sumDistinct(boolean condition, Property column, AggregatePredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().sumDistinct(condition, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default SQLWhereAggregatePredicate countDistinct(Property column, AggregatePredicateCompare compare, TProperty val) {
return countDistinct(true, column, compare, val);
}
default SQLWhereAggregatePredicate countDistinct(boolean condition, Property column, AggregatePredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().countDistinct(condition, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default > SQLWhereAggregatePredicate count(Property column, AggregatePredicateCompare compare, TProperty val) {
return count(true, column, compare, val);
}
default > SQLWhereAggregatePredicate count(boolean condition, Property column, SQLPredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().count(condition, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default SQLWhereAggregatePredicate func(boolean condition, ColumnFunction columnFunction, Property column, SQLPredicateCompare compare, TProperty val) {
getWhereAggregatePredicate().func(condition, columnFunction, EasyLambdaUtil.getPropertyName(column), compare, val);
return this;
}
default SQLWhereAggregatePredicate then(SQLWhereAggregatePredicate sub) {
getWhereAggregatePredicate().then(sub.getWhereAggregatePredicate());
return sub;
}
default SQLWhereAggregatePredicate and() {
return and(true);
}
default SQLWhereAggregatePredicate and(boolean condition) {
getWhereAggregatePredicate().and(condition);
return this;
}
default SQLWhereAggregatePredicate and(SQLExpression1> sqlAggregatePredicateSQLExpression) {
return and(true, sqlAggregatePredicateSQLExpression);
}
SQLWhereAggregatePredicate and(boolean condition, SQLExpression1> sqlAggregatePredicateSQLExpression);
default SQLWhereAggregatePredicate or() {
return or(true);
}
default SQLWhereAggregatePredicate or(boolean condition) {
getWhereAggregatePredicate().or(condition);
return this;
}
default SQLWhereAggregatePredicate or(SQLExpression1> sqlAggregatePredicateSQLExpression) {
return or(true, sqlAggregatePredicateSQLExpression);
}
SQLWhereAggregatePredicate or(boolean condition, SQLExpression1> sqlAggregatePredicateSQLExpression);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy