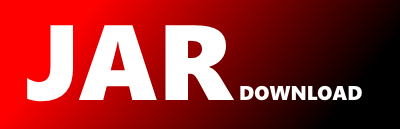
com.easy.query.api4j.sql.SQLWherePredicate Maven / Gradle / Ivy
package com.easy.query.api4j.sql;
import com.easy.query.api4j.sql.core.SQLLambdaNative;
import com.easy.query.api4j.sql.core.available.LambdaSQLFuncAvailable;
import com.easy.query.api4j.sql.core.filter.SQLAssertPredicate;
import com.easy.query.api4j.sql.core.filter.SQLFuncColumnPredicate;
import com.easy.query.api4j.sql.core.filter.SQLFuncValuePredicate;
import com.easy.query.api4j.sql.core.filter.SQLLikePredicate;
import com.easy.query.api4j.sql.core.filter.SQLRangePredicate;
import com.easy.query.api4j.sql.core.filter.SQLSelfPredicate;
import com.easy.query.api4j.sql.core.filter.SQLSubQueryPredicate;
import com.easy.query.api4j.sql.core.filter.SQLValuePredicate;
import com.easy.query.api4j.sql.core.filter.SQLValuesPredicate;
import com.easy.query.api4j.sql.impl.SQLWherePredicateImpl;
import com.easy.query.api4j.util.EasyLambdaUtil;
import com.easy.query.core.context.QueryRuntimeContext;
import com.easy.query.core.enums.SQLPredicateCompare;
import com.easy.query.core.expression.func.ColumnPropertyFunction;
import com.easy.query.core.expression.lambda.Property;
import com.easy.query.core.expression.lambda.SQLActionExpression;
import com.easy.query.core.expression.lambda.SQLExpression1;
import com.easy.query.core.expression.lambda.SQLExpression2;
import com.easy.query.core.expression.parser.core.EntitySQLTableOwner;
import com.easy.query.core.expression.parser.core.available.TableAvailable;
import com.easy.query.core.expression.parser.core.base.WherePredicate;
/**
* @author xuejiaming
* @FileName: WherePredicate.java
* @Description: 文件说明
* @Date: 2023/2/5 09:09
*/
public interface SQLWherePredicate extends EntitySQLTableOwner, LambdaSQLFuncAvailable, SQLLambdaNative>
, SQLLikePredicate>
, SQLRangePredicate>
, SQLSelfPredicate>
, SQLFuncColumnPredicate>
, SQLFuncValuePredicate>
, SQLValuePredicate>
, SQLValuesPredicate>
, SQLSubQueryPredicate>
, SQLAssertPredicate> {
WherePredicate getWherePredicate();
default TableAvailable getTable() {
return getWherePredicate().getTable();
}
default QueryRuntimeContext getRuntimeContext() {
return getWherePredicate().getRuntimeContext();
}
default SQLWherePredicate columnFunc(ColumnPropertyFunction columnPropertyFunction, SQLPredicateCompare sqlPredicateCompare, TProperty val) {
return columnFunc(true, columnPropertyFunction, sqlPredicateCompare, val);
}
default SQLWherePredicate columnFunc(boolean condition, ColumnPropertyFunction columnPropertyFunction, SQLPredicateCompare sqlPredicateCompare, TProperty val) {
getWherePredicate().columnFunc(condition, columnPropertyFunction, sqlPredicateCompare, val);
return this;
}
default SQLWherePredicate then(SQLWherePredicate sub) {
getWherePredicate().then(sub.getWherePredicate());
return sub;
}
default SQLWherePredicate and() {
return and(true);
}
default SQLWherePredicate and(boolean condition) {
getWherePredicate().and(condition);
return this;
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param sqlWherePredicateSQLExpression
* @return
*/
@Deprecated
default SQLWherePredicate and(SQLExpression1> sqlWherePredicateSQLExpression) {
return and(true, sqlWherePredicateSQLExpression);
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param condition
* @param sqlWherePredicateSQLExpression
* @return
*/
@Deprecated
SQLWherePredicate and(boolean condition, SQLExpression1> sqlWherePredicateSQLExpression);
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param sqlWherePredicateSQLExpression
* @return
*/
default SQLWherePredicate and(SQLActionExpression sqlWherePredicateSQLExpression) {
return and(true, sqlWherePredicateSQLExpression);
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param condition
* @param sqlWherePredicateSQLExpression
* @return
*/
default SQLWherePredicate and(boolean condition, SQLActionExpression sqlWherePredicateSQLExpression){
if(condition){
getWherePredicate().and(sqlWherePredicateSQLExpression);
}
return this;
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param t2SQLWherePredicate
* @param sqlWherePredicateSQLExpression
* @return
* @param
*/
@Deprecated
default SQLWherePredicate and(SQLWherePredicate t2SQLWherePredicate, SQLExpression2, SQLWherePredicate> sqlWherePredicateSQLExpression) {
return and(true, t2SQLWherePredicate, sqlWherePredicateSQLExpression);
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param condition
* @param t2SQLWherePredicate
* @param sqlWherePredicateSQLExpression
* @return
* @param
*/
@Deprecated
SQLWherePredicate and(boolean condition, SQLWherePredicate t2SQLWherePredicate, SQLExpression2, SQLWherePredicate> sqlWherePredicateSQLExpression);
default SQLWherePredicate or() {
return or(true);
}
default SQLWherePredicate or(boolean condition) {
getWherePredicate().or(condition);
return this;
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param sqlWherePredicateSQLExpression
* @return
*/
@Deprecated
default SQLWherePredicate or(SQLExpression1> sqlWherePredicateSQLExpression) {
return or(true, sqlWherePredicateSQLExpression);
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param condition
* @param sqlWherePredicateSQLExpression
* @return
*/
@Deprecated
SQLWherePredicate or(boolean condition, SQLExpression1> sqlWherePredicateSQLExpression);
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param sqlWherePredicateSQLExpression
* @return
*/
default SQLWherePredicate or(SQLActionExpression sqlWherePredicateSQLExpression) {
return or(true, sqlWherePredicateSQLExpression);
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param condition
* @param sqlWherePredicateSQLExpression
* @return
*/
default SQLWherePredicate or(boolean condition, SQLActionExpression sqlWherePredicateSQLExpression){
if(condition){
getWherePredicate().or(sqlWherePredicateSQLExpression);
}
return this;
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param t2SQLWherePredicate
* @param sqlWherePredicateSQLExpression
* @return
* @param
*/
@Deprecated
default SQLWherePredicate or(SQLWherePredicate t2SQLWherePredicate, SQLExpression2, SQLWherePredicate> sqlWherePredicateSQLExpression) {
return or(true, t2SQLWherePredicate, sqlWherePredicateSQLExpression);
}
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param condition
* @param t2SQLWherePredicate
* @param sqlWherePredicateSQLExpression
* @return
* @param
*/
@Deprecated
SQLWherePredicate or(boolean condition, SQLWherePredicate t2SQLWherePredicate, SQLExpression2, SQLWherePredicate> sqlWherePredicateSQLExpression);
/**
* 采用无参数and or处理括号和多表问题
* (t.`stars` = ? OR t1.`order` = ?) AND t1.`id` = ? AND (t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
*
* {@code
* .where((t1, t2) -> {
* t1.and(()->{
* t1.eq(Topic::getStars, 1).or();
* t2.eq(BlogEntity::getOrder, "1");
* }); //(t.`stars` = ? OR t1.`order` = ?)
* t2.eq(BlogEntity::getId,1);//t1.`id` = ?
* t2.and(()->{
* t1.eq(Topic::getStars, 1).or(()->{ //使用or表示or内部是括号括号和外面是or链接
* t1.eq(Topic::getCreateTime,LocalDateTime.now())
* .or();
* t2.like(BlogEntity::getContent,"111");
* });
* });//(t.`stars` = ? OR (t.`create_time` = ? OR t1.`content` LIKE ?))
* })}
*
* @param wherePredicate
* @return
* @param
*/
@Deprecated
default SQLWherePredicate withOther(SQLWherePredicate wherePredicate){
WherePredicate with = getWherePredicate().withOther(wherePredicate.getWherePredicate());
return new SQLWherePredicateImpl<>(with);
}
@Override
default SQLWherePredicate isBank(boolean condition, Property column) {
getWherePredicate().isBank(condition, EasyLambdaUtil.getPropertyName(column));
return this;
}
@Override
default SQLWherePredicate isNotBank(boolean condition, Property column) {
getWherePredicate().isNotBank(condition, EasyLambdaUtil.getPropertyName(column));
return this;
}
@Override
default SQLWherePredicate isEmpty(boolean condition, Property column) {
getWherePredicate().isEmpty(condition, EasyLambdaUtil.getPropertyName(column));
return this;
}
@Override
default SQLWherePredicate isNotEmpty(boolean condition, Property column) {
getWherePredicate().isNotEmpty(condition, EasyLambdaUtil.getPropertyName(column));
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy