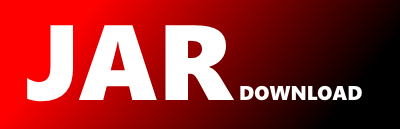
com.easyinnova.implementation_checker.model.TiffValidationObject Maven / Gradle / Ivy
Show all versions of tiffimplementationchecker Show documentation
/**
* TiffValidationObject.java
This program is free software: you can redistribute it
* and/or modify it under the terms of the GNU General Public License as published by the Free
* Software Foundation, either version 3 of the License, or (at your option) any later version; or,
* at your choice, under the terms of the Mozilla Public License, v. 2.0. SPDX GPL-3.0+ or MPL-2.0+.
*
This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR
* PURPOSE. See the GNU General Public License and the Mozilla Public License for more details.
* You should have received a copy of the GNU General Public License and the Mozilla Public
* License along with this program. If not, see http://www.gnu.org/licenses/
* and at http://mozilla.org/MPL/2.0 .
NB: for the
* © statement, include Easy Innova SL or other company/Person contributing the code.
©
* 2015 Easy Innova, SL
*
* @author Víctor Muñoz Sola
* @version 1.0
* @since 23/7/2015
*/
package com.easyinnova.implementation_checker.model;
import java.io.File;
import java.io.StringWriter;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlRootElement;
/**
* Created by easy on 08/03/2016.
*/
@XmlRootElement
public class TiffValidationObject extends TiffNode implements TiffNodeInterface {
TiffHeader header;
TiffIfds lifds;
long size;
String byteOrder;
int numberImages;
String iccProfileClass = "";
HashMap> hashObjects = null;
public void setSize(long size) {
this.size = size;
}
public long getSize() {
return size;
}
@XmlAttribute
public void setIccProfileClass(String iccProfileClass) {
this.iccProfileClass = iccProfileClass;
}
public String getIccProfileClass() {
return iccProfileClass;
}
@XmlAttribute
public void setByteOrder(String byteOrder) {
this.byteOrder = byteOrder;
}
public String getByteOrder() {
return byteOrder;
}
@XmlAttribute
public void setNumberImages(int numberImages) {
this.numberImages = numberImages;
}
public int getNumberImages() {
return numberImages;
}
public void setHeader(TiffHeader header) {
this.header = header;
}
public TiffHeader getHeader() {
return header;
}
public void setIfds(TiffIfds ifds) {
this.lifds = ifds;
}
public TiffIfds getIfds() {
return lifds;
}
public List getChildren(boolean subchilds) {
List childs = new ArrayList();
childs.add(header);
if (subchilds) childs.addAll(header.getChildren(subchilds));
childs.add(lifds);
if (subchilds) childs.addAll(lifds.getChildren(subchilds));
childs.add(new TiffSingleNode("size", size + ""));
// for policy checker
childs.add(new TiffSingleNode("byteOrder", byteOrder));
childs.add(new TiffSingleNode("numberImages", numberImages + ""));
childs.add(new TiffSingleNode("iccProfileClass", iccProfileClass + ""));
return childs;
}
public List getObjectsFromContext(String context, boolean subchilds) {
String key = context;
if (subchilds) key += "1"; else key += "0";
if (hashObjects != null && hashObjects.containsKey(key)) return hashObjects.get(key);
List objects = new ArrayList<>();
objects.add(this);
objects.addAll(getChildren(subchilds));
List objectsFromContext = new ArrayList();
for (TiffNode node : objects) {
if (node == null) {
// Why?
} else {
if (node.contextMatch(context)) {
objectsFromContext.add(node);
}
}
}
if (hashObjects == null) hashObjects = new HashMap<>();
hashObjects.put(key, objectsFromContext);
return objectsFromContext;
}
public String getContext() {
return "tiffValidationObject";
}
public void writeXml(String filename) {
try {
File file = new File(filename);
JAXBContext jaxbContext = JAXBContext.newInstance(TiffValidationObject.class);
Marshaller jaxbMarshaller = jaxbContext.createMarshaller();
// output pretty printed
jaxbMarshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
jaxbMarshaller.marshal(this, file);
} catch (JAXBException e) {
e.printStackTrace();
}
}
public String getXml() {
try {
JAXBContext jaxbContext = JAXBContext.newInstance(TiffValidationObject.class);
Marshaller jaxbMarshaller = jaxbContext.createMarshaller();
// output pretty printed
jaxbMarshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
StringWriter sw = new StringWriter();
jaxbMarshaller.marshal(this, sw);
return sw.toString();
} catch (JAXBException e) {
e.printStackTrace();
}
return "";
}
}