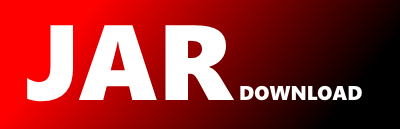
com.ebay.jetstream.event.processor.hdfs.util.JsonUtil Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright © 2012-2015 eBay Software Foundation
* This program is dual licensed under the MIT and Apache 2.0 licenses.
* Please see LICENSE for more information.
*******************************************************************************/
package com.ebay.jetstream.event.processor.hdfs.util;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Map;
import org.codehaus.jackson.map.JsonMappingException;
import org.codehaus.jackson.map.ObjectMapper;
import org.codehaus.jackson.type.TypeReference;
/**
* @author weifang
*
*/
public class JsonUtil {
public static Map jsonStringToMap(String data) {
try {
if (data == null)
return null;
ObjectMapper jsonMapper = new ObjectMapper();
TypeReference
© 2015 - 2025 Weber Informatics LLC | Privacy Policy