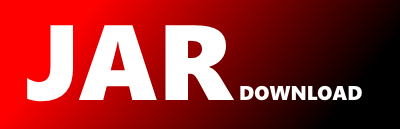
com.ebay.jetstream.util.FifoPriorityQueue Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright © 2012-2015 eBay Software Foundation
* This program is dual licensed under the MIT and Apache 2.0 licenses.
* Please see LICENSE for more information.
*******************************************************************************/
package com.ebay.jetstream.util;
import java.util.ArrayList;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.atomic.AtomicBoolean;
import com.ebay.jetstream.xmlser.XSerializable;
// import java.util.Vector;
/**
* An implementation of a queue of request messages. If a QueueMonitor is attached to this queue, it can monitor
* crossing of the high and low water mark thresholds and notify the queue monitor when thresholds are crossed
*
* *
*
* @author [email protected]
* @version 1.0
*/
/*
package com.ebay.jetstream.util;
import java.util.ArrayList; // import java.util.Vector;
import java.util.concurrent.ConcurrentLinkedQueue;
import java.util.concurrent.atomic.AtomicBoolean;
import com.ebay.jetstream.xmlser.XSerializable;
/**
* An implementation of a queue of request messages. If a QueueMonitor is attached to this queue, it can monitor
* crossing of the high and low water mark thresholds and notify the queue monitor when thresholds are crossed
*
* *
*
* @author [email protected]
* @version 1.0
*/
@edu.umd.cs.findbugs.annotations.SuppressWarnings(value="IS2_INCONSISTENT_SYNC")
public class FifoPriorityQueue implements XSerializable {
public static class FifoPriorityQueueException extends Exception {
/**
*
*/
private static final long serialVersionUID = 1L;
public final static int DATA_OUT_OF_RANGE_ERROR = 1;
public final static int HIGHWATERMARK_LESS_THAN_LOWWATERMARK = 2;
private int m_error = 0;
private String m_message;
/**
* @param error
* @param message
*/
public FifoPriorityQueueException(int error, String message) {
m_error = error;
m_message = message;
}
/**
* @return the error
*/
public int getError() {
return m_error;
}
/**
* @param error
* the error to set
*/
public void setError(int error) {
m_error = error;
}
/**
* @return the message
*/
public String getMessage() {
return m_message;
}
/**
* @param message
* the message to set
*/
public void setMessage(String message) {
m_message = message;
}
public boolean isDataOutOfRange() {
return (m_error == DATA_OUT_OF_RANGE_ERROR);
}
public boolean isHighWaterMarkLessThanLowWaterMark() {
return (m_error == HIGHWATERMARK_LESS_THAN_LOWWATERMARK);
}
}
public final static int HIGH_PRIORITY = 0;
public final static int LOW_PRIORITY = 1;
private float m_highWaterMark = (float) 0.85; // percentage of queue size
private float m_lowWaterMark = (float) 0.25; // percentage of queue size
private QueueMonitor m_queueMonitor = null;
private ArrayList m_pausedList; // each entry corresponds to a priority queue
private volatile boolean m_waitersWaiting = false;
/**
* An array of Vectors, each for a message priority The default constructor assumes only one entry.
*/
private ArrayList> m_requestList;
/**
* Number of priority types.
*/
private int m_numPriority;
/**
* Maximum allowable size of the queues. A value of -1 indicates no limit.
*/
private volatile int m_maxSize = -1;
/**
* Total number of messages comming in to this queue
*/
private long m_msgIn = 0;
/**
* Total number of messages going out of this queue
*/
private long m_msgOut = 0;
/**
* Default constructor. Uses only 1 priority type.
*/
public FifoPriorityQueue() {
m_requestList = new ArrayList>(1);
m_requestList.add(0, new ConcurrentLinkedQueue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy