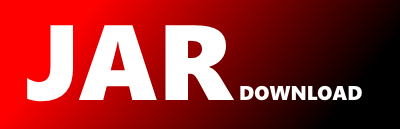
com.ecfeed.core.benchmark.BenchmarkResults Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
package com.ecfeed.core.benchmark;
public class BenchmarkResults {
private long fNumberOfGeneratedTests;
private long fProcessingTime;
private long fUsedMemory;
private String fNameAlgorithm;
private String fNameBenchmark;
private int fUsedMemoryIterations;
private Runtime fRuntime;
public BenchmarkResults() {
// This part does not have to work. We could try though.
// VM arguments -verbose:gc
System.gc();
System.runFinalization();
fNameAlgorithm = "";
fNameBenchmark = "";
fRuntime = Runtime.getRuntime();
}
BenchmarkResults setNameAlgorithm(String nameAlgorithm) {
fNameAlgorithm = nameAlgorithm;
return this;
}
BenchmarkResults setNameBenchmark(String nameBenchmark) {
fNameBenchmark = nameBenchmark;
return this;
}
BenchmarkResults updateNumberOfGeneratedTests() {
fNumberOfGeneratedTests++;
return this;
}
BenchmarkResults updateUsedMemory() {
fUsedMemory += (fRuntime.totalMemory() - fRuntime.freeMemory());
fUsedMemoryIterations++;
return this;
}
BenchmarkResults processingTimeStart() {
fProcessingTime = System.currentTimeMillis();
return this;
}
BenchmarkResults processingTimeEnd() {
fProcessingTime = System.currentTimeMillis() - fProcessingTime;
return this;
}
public long getNumberOfGeneratedTests() {
return fNumberOfGeneratedTests;
}
public long getProcessingTime() {
return fProcessingTime;
}
public long getUsedMemory() {
return fUsedMemory > 0 ? (fUsedMemory / fUsedMemoryIterations) / 1024 / 1024 : 0;
}
public String getNameAlgorithm() {
return fNameAlgorithm;
}
public String getNameBenchmark() {
return fNameBenchmark;
}
@Override
public String toString() {
return fNameAlgorithm
+ " | Case: " + fNumberOfGeneratedTests
+ " , Time: " + fProcessingTime
+ " , Memory: " + getUsedMemory() + " MB"
+ " | " + fNameBenchmark;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy