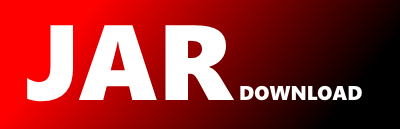
com.ecfeed.core.model.ChoiceCondition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.model;
import java.util.ArrayList;
import java.util.List;
import com.ecfeed.core.utils.EMathRelation;
import com.ecfeed.core.utils.EvaluationResult;
import com.ecfeed.core.utils.ExceptionHelper;
import com.ecfeed.core.utils.JavaTypeHelper;
import com.ecfeed.core.utils.MessageStack;
import com.ecfeed.core.utils.ObjectHelper;
import com.ecfeed.core.utils.RangeHelper;
import com.ecfeed.core.utils.RelationMatcher;
public class ChoiceCondition implements IStatementCondition {
private ChoiceNode fRightChoice;
private RelationStatement fParentRelationStatement;
public ChoiceCondition(ChoiceNode rightChoice, RelationStatement parentRelationStatement) {
fRightChoice = rightChoice;
fParentRelationStatement = parentRelationStatement;
}
@Override
public EvaluationResult evaluate(List choices) {
ChoiceNode choice =
StatementConditionHelper.getChoiceForMethodParameter(
choices, fParentRelationStatement.getLeftParameter());
if (choice == null) {
return EvaluationResult.INSUFFICIENT_DATA;
}
return evaluateChoice(choice);
}
@Override
public boolean adapt(List values) {
return false;
}
@Override
public ChoiceCondition getCopy() {
return new ChoiceCondition(fRightChoice.makeClone(), fParentRelationStatement);
}
@Override
public boolean updateReferences(MethodNode methodNode) {
String parameterName = fParentRelationStatement.getLeftParameter().getFullName();
MethodParameterNode methodParameterNode = methodNode.getMethodParameter(parameterName);
String choiceName = fRightChoice.getQualifiedName();
ChoiceNode choiceNode = methodParameterNode.getChoice(choiceName);
if (choiceNode == null) {
return false;
}
fRightChoice = choiceNode;
return true;
}
@Override
public Object getCondition(){
return fRightChoice;
}
@Override
public boolean compare(IStatementCondition condition) {
if (condition instanceof ChoiceCondition == false) {
return false;
}
ChoiceCondition compared = (ChoiceCondition)condition;
return (fRightChoice.isMatch((ChoiceNode)compared.getCondition()));
}
@Override
public Object accept(IStatementVisitor visitor) throws Exception {
return visitor.visit(this);
}
@Override
public String toString() {
return StatementConditionHelper.createChoiceDescription(fRightChoice.getQualifiedName());
}
@Override
public boolean mentions(MethodParameterNode methodParameterNode) {
return false;
}
@Override
public List getListOfChoices() {
List choices = new ArrayList();
choices.add(fRightChoice);
return choices;
}
public ChoiceNode getRightChoice() {
return fRightChoice;
}
private EvaluationResult evaluateChoice(ChoiceNode actualLeftChoice) {
String substituteType = getSubstituteType(actualLeftChoice);
boolean isRandomizedChoice =
StatementConditionHelper.getChoiceRandomized(
actualLeftChoice,
fParentRelationStatement.getLeftParameter());
if(isRandomizedChoice) {
return evaluateForRandomizedChoice(
actualLeftChoice.getValueString(),
substituteType);
}
return evaluateForConstantChoice(actualLeftChoice, substituteType);
}
private String getSubstituteType(ChoiceNode leftChoice) {
String typeName = leftChoice.getParameter().getType();
return JavaTypeHelper.getSubstituteType(typeName);
}
private EvaluationResult evaluateForConstantChoice(
ChoiceNode actualLeftChoice,
String substituteType) {
EMathRelation relation = fParentRelationStatement.getRelation();
if (relation == EMathRelation.EQUAL || relation == EMathRelation.NOT_EQUAL) {
return evaluateEqualityIncludingParents(relation, actualLeftChoice);
}
String actualLeftValue = JavaTypeHelper.convertValueString(actualLeftChoice.getValueString(), substituteType);
String rightValue = JavaTypeHelper.convertValueString(fRightChoice.getValueString(), substituteType);
if (RelationMatcher.isMatchQuiet(relation, substituteType, actualLeftValue, rightValue)) {
return EvaluationResult.TRUE;
}
return EvaluationResult.FALSE;
}
private EvaluationResult evaluateForRandomizedChoice(
String leftChoiceStr,
String substituteType) {
EMathRelation relation = fParentRelationStatement.getRelation();
String fRightValue = fRightChoice.getValueString();
if (JavaTypeHelper.isStringTypeName(substituteType)) {
return EvaluationResult.TRUE;
}
boolean result =
RangeHelper.isRightRangeInLeftRange(
leftChoiceStr, fRightValue, relation, substituteType);
return EvaluationResult.convertFromBoolean(result);
}
private EvaluationResult evaluateEqualityIncludingParents(EMathRelation relation, ChoiceNode choice) {
boolean isMatch = false;
if (choice == null || fRightChoice == null) {
isMatch = ObjectHelper.isEqualWhenOneOrTwoNulls(choice, fRightChoice);
} else {
isMatch = choice.isMatchIncludingParents(fRightChoice);
}
switch (relation) {
case EQUAL:
return EvaluationResult.convertFromBoolean(isMatch);
case NOT_EQUAL:
return EvaluationResult.convertFromBoolean(!isMatch);
default:
ExceptionHelper.reportRuntimeException("Invalid relation.");
return EvaluationResult.FALSE;
}
}
@Override
public boolean isAmbiguous(List> testDomain, MessageStack messageStack) {
String substituteType = ConditionHelper.getSubstituteType(fParentRelationStatement);
int leftParameterIndex = fParentRelationStatement.getLeftParameter().getMyIndex();
List choicesForParameter = testDomain.get(leftParameterIndex);
EMathRelation relation = fParentRelationStatement.getRelation();
for (ChoiceNode leftChoiceNode : choicesForParameter) {
if (isChoiceAmbiguous(leftChoiceNode, relation, substituteType, messageStack)) {
return true;
}
}
return false;
}
private boolean isChoiceAmbiguous(
ChoiceNode leftChoiceNode,
EMathRelation relation,
String substituteType,
MessageStack messageStack) {
if (!leftChoiceNode.isRandomizedValue()) {
return false;
}
if (ConditionHelper.isRandomizedChoiceAmbiguous(
leftChoiceNode, fRightChoice.getValueString(),
fParentRelationStatement, relation, substituteType)) {
ConditionHelper.addValuesMessageToStack(
leftChoiceNode.toString(), relation, fRightChoice.toString(), messageStack);
return true;
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy