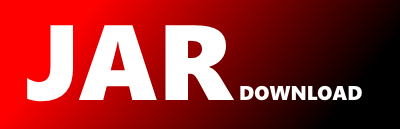
com.ecfeed.core.model.ChoiceNodeHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.model;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ChoiceNodeHelper {
public static ChoiceNode createSubstitutePath(ChoiceNode choice, MethodParameterNode parameter) {
List copies = createListOfCopies(choice);
setParentsOfChoices(copies, parameter);
return copies.get(0);
}
private static List createListOfCopies(ChoiceNode choice) {
ChoiceNode orgChoice = choice;
List copies = new ArrayList();
for(;;) {
ChoiceNode copy =
new ChoiceNode(
orgChoice.getFullName(), orgChoice.getModelChangeRegistrator(), orgChoice.getValueString());
copies.add(copy);
AbstractNode orgParent = orgChoice.getParent();
if (!(orgParent instanceof ChoiceNode)) {
break;
}
orgChoice = (ChoiceNode)orgParent;
}
return copies;
}
private static void setParentsOfChoices(List copies, MethodParameterNode parameter) {
int copiesSize = copies.size();
int lastIndex = copiesSize - 1;
for (int index = 0; index < lastIndex; index++) {
ChoiceNode current = copies.get(index);
ChoiceNode next = copies.get(index + 1);
current.setParent(next);
}
ChoiceNode last = copies.get(copiesSize - 1);
last.setParent(parameter);
}
public static Map> convertToParamAndChoiceNames(MethodNode methodNode, List> algorithmInput) {
Map> paramAndChoiceNames = new HashMap>();
int parametersCount = methodNode.getParametersCount();
for (int parameterIndex = 0; parameterIndex < parametersCount; parameterIndex++) {
String parameterName = methodNode.getParameter(parameterIndex).getFullName();
List choicesForParameter = algorithmInput.get(parameterIndex);
paramAndChoiceNames.put(parameterName, getChoiceNames(choicesForParameter));
}
return paramAndChoiceNames;
}
public static List getChoiceNames(List choiceNodes) {
List choiceNames = new ArrayList<>();
for (ChoiceNode choiceNode : choiceNodes) {
String choiceName = choiceNode.getQualifiedName();
choiceNames.add(choiceName);
}
return choiceNames;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy