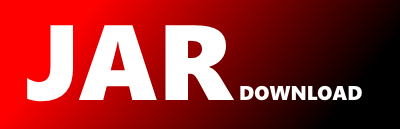
com.ecfeed.core.model.ChoicesParentNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.model;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
public abstract class ChoicesParentNode extends AbstractNode{
protected List fChoices;
public ChoicesParentNode(String name, IModelChangeRegistrator modelChangeRegistrator) {
super(name, modelChangeRegistrator);
fChoices = new ArrayList();
}
public abstract Object accept(IChoicesParentVisitor visitor) throws Exception;
@Override
public List extends AbstractNode> getChildren() {
return fChoices;
}
@Override
public boolean isMatch(AbstractNode node) {
if (node instanceof ChoicesParentNode == false) {
return false;
}
ChoicesParentNode comparedChoiceParent = (ChoicesParentNode)node;
if (getChoices().size() != comparedChoiceParent.getChoices().size()){
return false;
}
for (int i = 0; i < getChoices().size(); i++) {
if (getChoices().get(i).isMatch(comparedChoiceParent.getChoices().get(i)) == false) {
return false;
}
}
return super.isMatch(node);
}
public abstract AbstractParameterNode getParameter();
public void addChoice(ChoiceNode choice) {
addChoice(choice, fChoices.size());
}
public void addChoice(ChoiceNode choice, int index) {
fChoices.add(index, choice);
choice.setParent(this);
registerChange();
}
public void addChoices(List choices) {
for (ChoiceNode p : choices) {
addChoice(p);
}
}
public List getChoices() {
return fChoices;
}
public int getChoiceCount() {
return getChoices().size();
}
public List getChoicesWithCopies() {
return fChoices;
}
public ChoiceNode getChoice(String qualifiedName) {
return (ChoiceNode)getChild(qualifiedName);
}
public boolean choiceExistsAsDirectChild(String choiceNameToFind) {
for (ChoiceNode choiceNode : fChoices) {
if (choiceNode.getFullName().equals(choiceNameToFind)) {
return true;
}
}
return false;
}
public List getLeafChoices() {
return getLeafChoices(getChoices());
}
public List getLeafChoicesWithCopies() {
return getLeafChoices(getChoicesWithCopies());
}
public Set getAllChoices() {
return getAllChoices(getChoices());
}
public Set getChoiceNames() {
return getChoiceNames(getChoices());
}
public Set getAllChoiceNames() {
return getChoiceNames(getAllChoices());
}
public Set getLeafChoiceNames(){
return getChoiceNames(getLeafChoices());
}
public Set getLabeledChoices(String label) {
return getLabeledChoices(label, getChoices());
}
public Set getLeafLabels() {
Set result = new LinkedHashSet();
for (ChoiceNode p : getLeafChoices()) {
result.addAll(p.getAllLabels());
}
return result;
}
public Set getLeafChoiceValues() {
Set result = new LinkedHashSet();
for(ChoiceNode p : getLeafChoices()){
result.add(p.getValueString());
}
return result;
}
public boolean removeChoice(ChoiceNode choice) {
if (fChoices.contains(choice) && fChoices.remove(choice)) {
choice.setParent(null);
registerChange();
return true;
}
return false;
}
public void replaceChoices(List newChoices) {
fChoices.clear();
fChoices.addAll(newChoices);
for (ChoiceNode p : newChoices) {
p.setParent(this);
}
registerChange();
}
protected List getLeafChoices(Collection choices) {
List result = new ArrayList();
for (ChoiceNode p : choices) {
if (p.isAbstract() == false) {
result.add(p);
}
result.addAll(p.getLeafChoices());
}
return result;
}
protected Set getAllChoices(Collection choices) {
Set result = new LinkedHashSet();
for (ChoiceNode p : choices) {
result.add(p);
result.addAll(p.getAllChoices());
}
return result;
}
protected Set getChoiceNames(Collection choices) {
Set result = new LinkedHashSet();
for (ChoiceNode p : choices) {
result.add(p.getQualifiedName());
}
return result;
}
protected Set getLabeledChoices(String label, List choices) {
Set result = new LinkedHashSet();
for(ChoiceNode p : choices) {
if(p.getLabels().contains(label)){
result.add(p);
}
result.addAll(p.getLabeledChoices(label));
}
return result;
}
public static String generateNewChoiceName(ChoicesParentNode fChoicesParentNode, String startChoiceName) {
for (int i = 1; ; i++) {
String newParameterName = startChoiceName + String.valueOf(i);
if (!fChoicesParentNode.choiceExistsAsDirectChild(newParameterName)) {
return newParameterName;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy