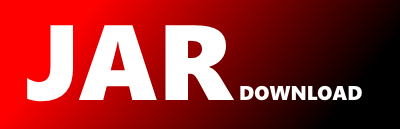
com.ecfeed.core.model.Constraint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.model;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import com.ecfeed.core.utils.EvaluationResult;
import com.ecfeed.core.utils.MessageStack;
public class Constraint implements IConstraint {
private String fName;
private final IModelChangeRegistrator fModelChangeRegistrator;
private AbstractStatement fPremise;
private AbstractStatement fConsequence;
public Constraint(String name,
IModelChangeRegistrator modelChangeRegistrator,
AbstractStatement premise,
AbstractStatement consequence) {
if (name == null) {
fName = "constraint";
}
fName = name;
fModelChangeRegistrator = modelChangeRegistrator;
fPremise = premise;
fConsequence = consequence;
}
public String getName() {
return fName;
}
public void setName(String name) {
fName = name;
}
public boolean isAmbiguous(
List> testDomain,
MessageStack outWhyAmbiguous) {
if (isAmbiguousForPremiseOrConsequence(testDomain, outWhyAmbiguous)) {
ConditionHelper.addConstraintNameToMesageStack(getName(), outWhyAmbiguous);
return true;
}
return false;
}
private boolean isAmbiguousForPremiseOrConsequence(
List> testDomain, MessageStack outWhyAmbiguous) {
if (fPremise.isAmbiguous(testDomain, outWhyAmbiguous)) {
return true;
}
if (fConsequence.isAmbiguous(testDomain, outWhyAmbiguous)) {
return true;
}
return false;
}
@Override
public EvaluationResult evaluate(List values) {
if (fPremise == null) {
return EvaluationResult.TRUE;
}
EvaluationResult premiseEvaluationResult = fPremise.evaluate(values);
if (premiseEvaluationResult == EvaluationResult.FALSE) {
return EvaluationResult.TRUE;
}
if (premiseEvaluationResult == EvaluationResult.INSUFFICIENT_DATA) {
return EvaluationResult.INSUFFICIENT_DATA;
}
if (fConsequence == null) {
return EvaluationResult.FALSE;
}
EvaluationResult consequenceEvaluationResult = fConsequence.evaluate(values);
if (consequenceEvaluationResult == EvaluationResult.TRUE) {
return EvaluationResult.TRUE;
}
if (consequenceEvaluationResult == EvaluationResult.INSUFFICIENT_DATA) {
return EvaluationResult.INSUFFICIENT_DATA;
}
return EvaluationResult.FALSE;
}
@Override
public boolean adapt(List values) {
if (fPremise == null) {
return true;
}
if (fPremise.evaluate(values) == EvaluationResult.TRUE) {
return fConsequence.adapt(values);
}
return true;
}
@Override
public String toString() {
String premiseString = (fPremise != null) ? fPremise.toString() : "EMPTY";
String consequenceString = (fConsequence != null) ? fConsequence.toString() : "EMPTY";
return premiseString + " \u21d2 " + consequenceString;
}
@Override
public boolean mentions(int dimension) {
if (fPremise.mentions(dimension)) {
return true;
}
if (fConsequence.mentions(dimension)) {
return true;
}
return false;
}
public AbstractStatement getPremise() {
return fPremise;
}
public AbstractStatement getConsequence() {
return fConsequence;
}
public void setPremise(AbstractStatement statement) {
fPremise = statement;
if (fModelChangeRegistrator != null) {
fModelChangeRegistrator.registerChange();
}
}
public void setConsequence(AbstractStatement consequence) {
fConsequence = consequence;
if (fModelChangeRegistrator != null) {
fModelChangeRegistrator.registerChange();
}
}
public boolean mentions(MethodParameterNode parameter) {
return fPremise.mentions(parameter) || fConsequence.mentions(parameter);
}
public boolean mentions(MethodParameterNode parameter, String label) {
return fPremise.mentions(parameter, label) || fConsequence.mentions(parameter, label);
}
public boolean mentions(ChoiceNode choice) {
return fPremise.mentions(choice) || fConsequence.mentions(choice);
}
public List getListOfChoices() { // TODO - remove ? exists: getReferencedChoices
List result = new ArrayList();
result.addAll(fPremise.getListOfChoices());
result.addAll(fConsequence.getListOfChoices());
return result;
}
public boolean mentionsParameterAndOrderRelation(MethodParameterNode parameter) {
if (fPremise.mentionsParameterAndOrderRelation(parameter)) {
return true;
}
if (fConsequence.mentionsParameterAndOrderRelation(parameter)) {
return true;
}
return false;
}
public Constraint getCopy(){
AbstractStatement premise = fPremise.getCopy();
AbstractStatement consequence = fConsequence.getCopy();
return new Constraint(new String(fName), fModelChangeRegistrator, premise, consequence);
}
public boolean updateRefrences(MethodNode method) {
if (fPremise.updateReferences(method) && fConsequence.updateReferences(method)) {
return true;
}
return false;
}
@SuppressWarnings("unchecked")
public Set getReferencedChoices() {
try {
Set referenced = (Set)fPremise.accept(new ReferencedChoicesProvider());
referenced.addAll((Set)fConsequence.accept(new ReferencedChoicesProvider()));
return referenced;
} catch(Exception e) {
return new HashSet();
}
}
@SuppressWarnings("unchecked")
public Set getReferencedParameters() {
try{
Set referenced =
(Set)fPremise.accept(new ReferencedParametersProvider());
referenced.addAll(
(Set)fConsequence.accept(new ReferencedParametersProvider()));
return referenced;
} catch(Exception e) {
return new HashSet();
}
}
@SuppressWarnings("unchecked")
public Set getReferencedLabels(MethodParameterNode parameter) {
try {
Set referenced = (Set)fPremise.accept(new ReferencedLabelsProvider(parameter));
referenced.addAll((Set)fConsequence.accept(new ReferencedLabelsProvider(parameter)));
return referenced;
} catch(Exception e) {
return new HashSet();
}
}
boolean mentionsParameter(MethodParameterNode methodParameter) {
if (fPremise.mentions(methodParameter)) {
return true;
}
if (fConsequence.mentions(methodParameter)) {
return true;
}
return false;
}
private class ReferencedChoicesProvider implements IStatementVisitor {
@Override
public Object visit(StaticStatement statement) throws Exception {
return new HashSet();
}
@SuppressWarnings("unchecked")
@Override
public Object visit(StatementArray statement) throws Exception {
Set set = new HashSet();
for (AbstractStatement s : statement.getStatements()) {
set.addAll((Set)s.accept(this));
}
return set;
}
@Override
public Object visit(ExpectedValueStatement statement) throws Exception {
Set result = new HashSet<>();
if (statement.isParameterPrimitive()) {
result.add(statement.getCondition());
}
return result;
}
@Override
public Object visit(RelationStatement statement) throws Exception {
return statement.getCondition().accept(this);
}
@Override
public Object visit(LabelCondition condition) throws Exception {
return new HashSet();
}
@Override
public Object visit(ChoiceCondition condition) throws Exception {
Set set = new HashSet();
set.add(condition.getRightChoice());
return set;
}
@Override
public Object visit(ParameterCondition condition) throws Exception {
return new HashSet();
}
@Override
public Object visit(ValueCondition condition) throws Exception {
return new HashSet();
}
}
private class ReferencedParametersProvider implements IStatementVisitor {
@Override
public Object visit(StaticStatement statement) throws Exception {
return new HashSet();
}
@SuppressWarnings("unchecked")
@Override
public Object visit(StatementArray statement) throws Exception {
Set set = new HashSet();
for (AbstractStatement s : statement.getStatements()) {
set.addAll((Set)s.accept(this));
}
return set;
}
@Override
public Object visit(ExpectedValueStatement statement) throws Exception {
Set set = new HashSet();
set.add(statement.getParameter());
return set;
}
@Override
public Object visit(RelationStatement statement) throws Exception {
return statement.getCondition().accept(this);
}
@Override
public Object visit(LabelCondition condition) throws Exception {
return new HashSet();
}
@Override
public Object visit(ChoiceCondition condition) throws Exception {
Set set = new HashSet();
AbstractParameterNode parameter = condition.getRightChoice().getParameter();
if (parameter != null) {
set.add(parameter);
}
return set;
}
@Override
public Object visit(ParameterCondition condition) throws Exception {
Set set = new HashSet();
set.add(condition.getRightParameterNode());
return set;
}
@Override
public Object visit(ValueCondition condition) throws Exception {
return new HashSet();
}
}
private class ReferencedLabelsProvider implements IStatementVisitor {
private MethodParameterNode fParameter;
private Set EMPTY_SET = new HashSet();
public ReferencedLabelsProvider(MethodParameterNode parameter) {
fParameter = parameter;
}
@Override
public Object visit(StaticStatement statement) throws Exception {
return EMPTY_SET;
}
@SuppressWarnings("unchecked")
@Override
public Object visit(StatementArray statement) throws Exception {
Set set = new HashSet();
for (AbstractStatement s : statement.getStatements()) {
set.addAll((Set)s.accept(this));
}
return set;
}
@Override
public Object visit(ExpectedValueStatement statement) throws Exception {
return EMPTY_SET;
}
@Override
public Object visit(RelationStatement statement) throws Exception {
if (fParameter == statement.getLeftParameter()) {
return statement.getCondition().accept(this);
}
return EMPTY_SET;
}
@Override
public Object visit(LabelCondition condition) throws Exception {
Set result = new HashSet();
result.add(condition.getRightLabel());
return result;
}
@Override
public Object visit(ChoiceCondition condition) throws Exception {
return EMPTY_SET;
}
@Override
public Object visit(ParameterCondition condition) throws Exception {
return EMPTY_SET;
}
@Override
public Object visit(ValueCondition condition) throws Exception {
return EMPTY_SET;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy