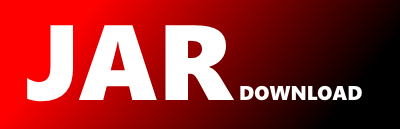
com.ecfeed.core.model.ConstraintNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.model;
import java.util.List;
import java.util.Set;
import com.ecfeed.core.utils.EvaluationResult;
import com.ecfeed.core.utils.JavaTypeHelper;
public class ConstraintNode extends AbstractNode{
private Constraint fConstraint;
@Override
public int getMyIndex() {
if (getMethod() == null) {
return -1;
}
return getMethod().getConstraintNodes().indexOf(this);
}
@Override
public String toString() {
return getFullName() + ": " + getConstraint().toString();
}
@Override
public void setFullName(String name) {
super.setFullName(name);
fConstraint.setName(name);
}
@Override
public ConstraintNode makeClone() {
ConstraintNode copy = new ConstraintNode(getFullName(), getModelChangeRegistrator(), fConstraint.getCopy() );
copy.setProperties(getProperties());
return copy;
}
public ConstraintNode(String name, IModelChangeRegistrator modelChangeRegistrator, Constraint constraint) {
super(name, modelChangeRegistrator);
fConstraint = constraint;
}
public Constraint getConstraint() {
return fConstraint;
}
public List getListOfChoices() {
return fConstraint.getListOfChoices();
}
public MethodNode getMethod() {
AbstractNode parent = getParent();
if (parent == null) {
return null;
}
if (parent instanceof MethodNode) {
return (MethodNode)parent;
}
return null;
}
public void setMethod(MethodNode method) {
setParent(method);
registerChange();
}
public EvaluationResult evaluate(List values) {
if (fConstraint != null) {
return fConstraint.evaluate(values);
}
return EvaluationResult.FALSE;
}
public boolean mentions(ChoiceNode choice) {
if (fConstraint.mentions(choice)) {
return true;
}
return false;
}
public boolean mentions(MethodParameterNode parameter) {
return fConstraint.mentions(parameter);
}
public boolean mentions(AbstractParameterNode parameter) {
if (parameter instanceof MethodParameterNode) {
MethodParameterNode param = (MethodParameterNode)parameter;
return fConstraint.mentions(param);
} else if (parameter instanceof GlobalParameterNode) {
GlobalParameterNode global = (GlobalParameterNode)parameter;
for (MethodParameterNode methodParam: global.getLinkers()) {
return fConstraint.mentions(methodParam);
}
}
return false;
}
public boolean mentions(MethodParameterNode parameter, String label) {
return fConstraint.mentions(parameter, label);
}
public boolean updateReferences(MethodNode method) {
if (fConstraint.updateRefrences(method)) {
setParent(method);
registerChange();
return true;
}
return false;
}
public ConstraintNode getCopy(MethodNode method) {
ConstraintNode copy = makeClone();
if (copy.updateReferences(method))
return copy;
else
return null;
}
@Override
public boolean isMatch(AbstractNode node) {
if (node instanceof ConstraintNode == false) {
return false;
}
ConstraintNode compared = (ConstraintNode)node;
if (getConstraint().getPremise().compare(compared.getConstraint().getPremise()) == false) {
return false;
}
if (getConstraint().getConsequence().compare(compared.getConstraint().getConsequence()) == false) {
return false;
}
return super.isMatch(node);
}
@Override
public Object accept(IModelVisitor visitor) throws Exception {
return visitor.visit(this);
}
public boolean isConsistent() {
if (!areParametersConsistent()) {
return false;
}
if (!areChoicesConsistent()) {
return false;
}
if (!constraintsConsistent()) {
return false;
}
return true;
}
private boolean areParametersConsistent() {
final Set referencedParameters = getConstraint().getReferencedParameters();
final List methodParameters = getMethod().getParameters();
for (AbstractParameterNode referencedParameter : referencedParameters) {
if (!isParameterConsistent(referencedParameter, methodParameters)) {
return false;
}
}
return true;
}
private boolean isParameterConsistent(
AbstractParameterNode argParameter,
List methodParameters) {
for (AbstractParameterNode param : methodParameters) {
MethodParameterNode methodParam = (MethodParameterNode) param;
if (methodParam.isLinked() && methodParam.getLink().equals(argParameter)) {
return true;
}
}
if (!methodParameters.contains(argParameter)) {
return false;
}
return true;
}
private boolean areChoicesConsistent() {
Set referencedChoices = getConstraint().getReferencedChoices();
for (ChoiceNode choiceNode : referencedChoices) {
if (!isChoiceConsistent(choiceNode)) {
return false;
}
}
return true;
}
private boolean isChoiceConsistent(ChoiceNode choiceNode) {
if (choiceNode.getQualifiedName() == null) {
return false;
}
if (!isOkForExpectedParameter(choiceNode)) {
return false;
}
AbstractParameterNode parameter = choiceNode.getParameter();
List parameterMethods = parameter.getMethods();
if (parameterMethods == null) {
return false;
}
MethodNode methodNode = getMethod();
if (parameterMethods.contains(methodNode) == false) {
return false;
}
return true;
}
private static boolean isOkForExpectedParameter(ChoiceNode choiceNode) {
AbstractParameterNode parameter = choiceNode.getParameter();
if (parameter == null && !isMethodParameterNodeExpected(parameter)) {
return false;
}
return true;
}
private static boolean isMethodParameterNodeExpected(AbstractParameterNode parameter) {
if (!(parameter instanceof MethodParameterNode)) {
return false;
}
if (((MethodParameterNode)parameter).isExpected()) {
return true;
}
return false;
}
private boolean constraintsConsistent() {
for (MethodParameterNode parameter : getMethod().getMethodParameters()) {
if (!isConsistentForParameter(parameter)) {
return false;
}
}
return true;
}
private boolean isConsistentForParameter(MethodParameterNode parameter) {
String typeName = parameter.getType();
if (isForbiddenTypeForOrderRelations(typeName)) {
if (fConstraint.mentionsParameterAndOrderRelation(parameter)) {
return false;
}
}
if (!checkLabels(parameter)) {
return false;
}
return true;
}
private boolean isForbiddenTypeForOrderRelations(String typeName) {
if (JavaTypeHelper.isUserType(typeName)) {
return true;
}
if (JavaTypeHelper.isBooleanTypeName(typeName)) {
return true;
}
return false;
}
private boolean checkLabels(MethodParameterNode parameter) {
for (String label : getConstraint().getReferencedLabels(parameter)) {
if (!parameter.getLeafLabels().contains(label)) {
return false;
}
}
return true;
}
@Override
public int getMaxIndex() {
if (getMethod() != null) {
return getMethod().getConstraintNodes().size();
}
return -1;
}
boolean mentionsParameter(MethodParameterNode methodParameter) {
return fConstraint.mentionsParameter(methodParameter);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy