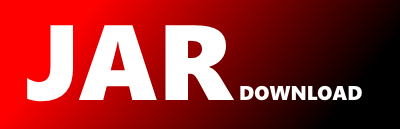
com.ecfeed.core.model.IsNodeIncludedInGenerationPredicate Maven / Gradle / Ivy
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.model;
import java.util.ArrayList;
import java.util.List;
public class IsNodeIncludedInGenerationPredicate {
private final MethodNode fGeneratorMethodNode;
private final List> fAllowedChoiceInput;
private final List> fAllowedConstraints;
public IsNodeIncludedInGenerationPredicate(
MethodNode generatorMethodNode,
List> allowedChoiceInput,
List> allowedConstraints) {
fGeneratorMethodNode = generatorMethodNode;
if (allowedChoiceInput == null) {
fAllowedChoiceInput = new ArrayList>();
} else {
fAllowedChoiceInput = allowedChoiceInput;
}
if (allowedConstraints != null) {
fAllowedChoiceInput.add(getListOfChoices(allowedConstraints));
}
if (allowedConstraints == null) {
fAllowedConstraints = new ArrayList>();
} else {
fAllowedConstraints = allowedConstraints;
}
}
private static List getListOfChoices(List> allowedConstraints) {
List listOfChoices = new ArrayList();
for (IConstraint iconstraint : allowedConstraints) {
Constraint constraint = (Constraint)iconstraint;
List referencedConstraints = constraint.getListOfChoices();
listOfChoices.addAll(referencedConstraints);
}
return listOfChoices;
}
public boolean test(AbstractNode abstractNode) {
if (abstractNode instanceof RootNode) {
return true;
}
if (abstractNode instanceof ClassNode) {
return shouldSerializeClassNode(abstractNode, fGeneratorMethodNode);
}
if (abstractNode instanceof MethodNode) {
return shouldSerializeMethodNode(abstractNode, fGeneratorMethodNode);
}
if (abstractNode instanceof MethodParameterNode) {
return shouldSerializeMethodParameterNode(abstractNode, fGeneratorMethodNode);
}
if (abstractNode instanceof GlobalParameterNode) {
return shouldSerializeGlobalParameterNode(abstractNode, fAllowedChoiceInput);
}
if (abstractNode instanceof ChoiceNode) {
return shouldSerializeChoiceNode(abstractNode, fAllowedChoiceInput, fAllowedConstraints);
}
if (abstractNode instanceof ConstraintNode) {
return shouldSerializeConstraintNode(abstractNode, fAllowedConstraints);
}
return false;
}
private static boolean shouldSerializeClassNode(AbstractNode abstractNode, MethodNode generatorMethodNode) {
ClassNode classNode = (ClassNode)abstractNode;
ClassNode parentOfMethod = (ClassNode)generatorMethodNode.getParent();
if (classNode.equals(parentOfMethod)) {
return true;
}
return false;
}
private static boolean shouldSerializeMethodNode(AbstractNode abstractNode, MethodNode generatorMethodNode) {
MethodNode methodNode = (MethodNode)abstractNode;
if (methodNode.equals(generatorMethodNode)) {
return true;
}
return false;
}
private static boolean shouldSerializeMethodParameterNode(
AbstractNode abstractNode, MethodNode generatorMethodNode) {
if (abstractNode.getParent().equals(generatorMethodNode)) {
return true;
}
return false;
}
private static boolean shouldSerializeGlobalParameterNode(
AbstractNode abstractNode,
List> allowedChoiceInput) {
return isAncestorOfAllowedChoices(abstractNode, allowedChoiceInput);
}
private static boolean shouldSerializeChoiceNode(
AbstractNode abstractNode,
List> allowedChoiceInput,
List> allowedConstraints) {
ChoiceNode choiceNode = (ChoiceNode)abstractNode;
if (isChoiceAllowed(choiceNode, allowedChoiceInput)) {
return true;
}
return isAncestorOfAllowedChoices(abstractNode, allowedChoiceInput);
}
private static boolean shouldSerializeConstraintNode(
AbstractNode abstractNode,
List> allowedConstraints) {
ConstraintNode constraintNode = (ConstraintNode)abstractNode;
Constraint constraintFromNode = constraintNode.getConstraint();
for (IConstraint constraint : allowedConstraints) {
if (constraint.equals(constraintFromNode)) {
return true;
}
}
return false;
}
private static boolean isChoiceAllowed(ChoiceNode testedChoiceNode, List> allowedChoiceInput) {
for (List choiceList : allowedChoiceInput) {
for (ChoiceNode allowedChoiceNode : choiceList) {
if (allowedChoiceNode.equals(testedChoiceNode)) {
return true;
}
}
}
return false;
}
private static boolean isAncestorOfAllowedChoices(
AbstractNode abstractNode,
List> allowedChoiceInput) {
for (List choiceList : allowedChoiceInput) {
for (ChoiceNode choiceNode : choiceList) {
if (choiceNode.isMyAncestor(abstractNode)) {
return true;
}
}
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy