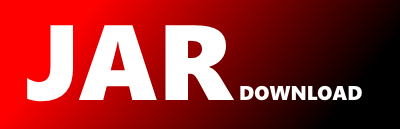
com.ecfeed.core.model.MethodNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ecfeed.junit Show documentation
Show all versions of ecfeed.junit Show documentation
An open library used to connect to the ecFeed service. It can be also used as a standalone testing tool. It is integrated with Junit5 and generates a stream of test cases using a selected algorithm (e.g. Cartesian, N-Wise). There are no limitations associated with the off-line version but the user cannot access the on-line computation servers and the model database.
The newest version!
/*******************************************************************************
*
* Copyright (c) 2016 ecFeed AS.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
*******************************************************************************/
package com.ecfeed.core.model;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
public class MethodNode extends ParametersParentNode {
private List fTestCases;
private List fConstraints;
public MethodNode(String name, IModelChangeRegistrator modelChangeRegistrator){
super(name, modelChangeRegistrator);
fTestCases = new ArrayList();
fConstraints = new ArrayList();
setDefaultPropertyValues();
}
private void setDefaultPropertyValues() {
setPropertyDefaultValue(NodePropertyDefs.PropertyId.PROPERTY_METHOD_RUNNER);
setPropertyDefaultValue(NodePropertyDefs.PropertyId.PROPERTY_MAP_BROWSER_TO_PARAM);
setPropertyDefaultValue(NodePropertyDefs.PropertyId.PROPERTY_WEB_BROWSER);
setPropertyDefaultValue(NodePropertyDefs.PropertyId.PROPERTY_MAP_START_URL_TO_PARAM);
registerChange();
}
public static interface ConstraintsItr {
}
private static class ConstraintsItrImpl implements ConstraintsItr {
Iterator fIterator;
ConstraintsItrImpl(Iterator iterator) {
fIterator = iterator;
}
}
public ConstraintsItr getIterator() {
return new ConstraintsItrImpl(fConstraints.iterator());
}
public boolean hasNextConstraint(ConstraintsItr contIterator) {
return ((ConstraintsItrImpl)contIterator).fIterator.hasNext();
}
public ConstraintNode getNextConstraint(ConstraintsItr contIterator) {
return ((ConstraintsItrImpl)contIterator).fIterator.next();
}
public void removeConstraint(ConstraintsItr contIterator) {
((ConstraintsItrImpl)contIterator).fIterator.remove();
registerChange();
}
@Override
public String toString(){
String result = new String(getFullName()) + "(";
List types = getParameterTypes();
List names = getParametersNames();
for(int i = 0; i < types.size(); i++){
if(getMethodParameters().get(i).isExpected()){
result += "[e]";
}
result += types.get(i);
result += " ";
result += names.get(i);
if(i < types.size() - 1) result += ", ";
}
result += ")";
return result;
}
public String getShortSignature() {
List types = getParameterTypes();
List names = getParametersNames();
String result = new String(getFullName()) + "(";
for (int i = 0; i < types.size(); i++) {
result += types.get(i);
result += " ";
result += names.get(i);
if (i < types.size() - 1) {
result += ", ";
}
}
result += ")";
return result;
}
public String getLongSignature() {
return getParent().getFullName() + "." + getShortSignature();
}
@Override
public List extends AbstractNode> getChildren(){
List children = new ArrayList(super.getChildren());
children.addAll(fConstraints);
children.addAll(fTestCases);
return children;
}
@Override
public boolean hasChildren(){
return(getParameters().size() != 0 || fConstraints.size() != 0 || fTestCases.size() != 0);
}
@Override
public MethodNode makeClone(){
MethodNode copy = new MethodNode(getFullName(), getModelChangeRegistrator());
copy.setProperties(getProperties());
for(MethodParameterNode parameter : getMethodParameters()){
copy.addParameter(parameter.makeClone());
}
for(TestCaseNode testcase : fTestCases){
TestCaseNode tcase = testcase.getCopy(copy);
if(tcase != null)
copy.addTestCase(tcase);
}
for(ConstraintNode constraint : fConstraints){
constraint = constraint.getCopy(copy);
if(constraint != null)
copy.addConstraint(constraint);
}
copy.setParent(getParent());
return copy;
}
public int getMyMethodIndex() {
if (getParent() == null) {
return -1;
}
int index = -1;
for (AbstractNode abstractNode : getParent().getChildren()) {
if (abstractNode instanceof MethodNode) {
index++;
}
if (abstractNode.equals(this)) {
return index;
}
}
return -1;
}
public MethodNode getSibling(List argTypes){
ClassNode parent = getClassNode();
if(parent == null) return null;
MethodNode sibling = parent.getMethod(getFullName(), argTypes);
if(sibling == null || sibling == this){
return null;
}
return sibling;
}
public boolean checkDuplicate(int index, String newType){
List argTypes = getParameterTypes();
argTypes.set(index, newType);
return getSibling(argTypes) != null;
}
public void addConstraint(ConstraintNode constraint) {
addConstraint(constraint, fConstraints.size());
}
public void addConstraint(ConstraintNode constraint, int index) {
constraint.setParent(this);
fConstraints.add(index, constraint);
registerChange();
}
public void addTestCase(TestCaseNode testCase){
addTestCase(testCase, fTestCases.size());
}
public void addTestCase(TestCaseNode testCase, int index){
fTestCases.add(index, testCase);
testCase.setParent(this);
registerChange();
}
public ClassNode getClassNode() {
return (ClassNode)getParent();
}
public boolean getRunOnAndroid() {
ClassNode classNode = getClassNode();
return classNode.getRunOnAndroid();
}
public MethodParameterNode getMethodParameter(String name){
return (MethodParameterNode)getParameter(name);
}
public ArrayList getParametersNames(boolean expected) {
ArrayList names = new ArrayList();
for(MethodParameterNode parameter : getMethodParameters()){
if(parameter.isExpected() == expected){
names.add(parameter.getFullName());
}
}
return names;
}
public MethodParameterNode getMethodParameter(int index) {
return (MethodParameterNode)(getParameters().get(index));
}
public int getMethodParameterCount()
{
return getParameters().size();
}
public List getConstraintNodes(){
return fConstraints;
}
public List> getAllConstraints(){
List> constraints = new ArrayList>();
for(ConstraintNode node : fConstraints){
constraints.add(node.getConstraint());
}
return constraints;
}
public List> getConstraints(String name) {
List> constraints = new ArrayList>();
for(ConstraintNode node : fConstraints){
if(node.getFullName().equals(name)){
constraints.add(node.getConstraint());
}
}
return constraints;
}
public Set getConstraintsNames() {
Set names = new HashSet();
for(ConstraintNode constraint : fConstraints){
names.add(constraint.getFullName());
}
return names;
}
public List> getTestDomain() {
List> testDomain = new ArrayList<>();
int parameterCount = getParametersCount();
for (int parameterIndex = 0; parameterIndex < parameterCount; parameterIndex++) {
testDomain.add(getTestDomainProcessAbstractParameterNode(parameterIndex));
}
return testDomain;
}
private List getTestDomainProcessAbstractParameterNode(int parameterIndex) {
AbstractParameterNode abstractParameterNode = getParameter(parameterIndex);
if (abstractParameterNode instanceof MethodParameterNode) {
return getTestDomainProcessMethodParameterNode(abstractParameterNode);
} else {
return abstractParameterNode.getLeafChoicesWithCopies();
}
}
private List getTestDomainProcessMethodParameterNode(AbstractParameterNode abstractParameterNode) {
MethodParameterNode methodParameterNode = (MethodParameterNode) abstractParameterNode;
List choicesForParameter;
if (methodParameterNode.isExpected()) {
ChoiceNode choiceNode = getTestDomainCreateExpectedChoiceNode(methodParameterNode);
choicesForParameter = new ArrayList<>();
choicesForParameter.add(choiceNode);
} else {
choicesForParameter = abstractParameterNode.getLeafChoicesWithCopies();
}
return choicesForParameter;
}
private ChoiceNode getTestDomainCreateExpectedChoiceNode(MethodParameterNode methodParameterNode) {
String defaultValue = methodParameterNode.getDefaultValue();
ChoiceNode choiceNode = new ChoiceNode("@expected", methodParameterNode.getModelChangeRegistrator(), defaultValue);
choiceNode.setParent(methodParameterNode);
return choiceNode;
}
public List getTestCases(){
return fTestCases;
}
public boolean hasParameters() {
if (getParameters().isEmpty()) {
return false;
}
return true;
}
public boolean hasTestCases() {
if (fTestCases.isEmpty()) {
return false;
}
return true;
}
public Collection getTestCases(String testSuite) {
ArrayList testCases = new ArrayList();
for(TestCaseNode testCase : getTestCases()){
if(testSuite.equals(testCase.getFullName())){
testCases.add(testCase);
}
}
return testCases;
}
public Set getTestCaseNames() {
Set names = new HashSet();
for(TestCaseNode testCase : getTestCases()){
names.add(testCase.getFullName());
}
return names;
}
public boolean removeTestCase(TestCaseNode testCase){
testCase.setParent(null);
boolean result = fTestCases.remove(testCase);
registerChange();
return result;
}
public void removeTestCases(){
fTestCases.clear();
registerChange();
}
public boolean removeConstraint(ConstraintNode constraint) {
constraint.setParent(null);
boolean result = fConstraints.remove(constraint);
registerChange();
return result;
}
public void removeTestSuite(String suiteName) {
Iterator iterator = getTestCases().iterator();
while(iterator.hasNext()){
TestCaseNode testCase = iterator.next();
if(testCase.getFullName().equals(suiteName)){
iterator.remove();
}
}
registerChange();
}
public boolean isChoiceMentioned(ChoiceNode choice){
for(ConstraintNode constraint : fConstraints){
if(constraint.mentions(choice)){
return true;
}
}
for(TestCaseNode testCase: fTestCases){
if(testCase.mentions(choice)){
return true;
}
}
return false;
}
public Set getMentioningConstraints(Collection parameters) {
Set result = new HashSet();
for(MethodParameterNode parameter : parameters){
result.addAll(getMentioningConstraints(parameter));
}
return result;
}
public Set getMentioningConstraints(MethodParameterNode parameter) {
Set result = new HashSet();
for(ConstraintNode constraint : fConstraints){
if(constraint.mentions(parameter)){
result.add(constraint);
}
}
return result;
}
public Set getMentioningConstraints(MethodParameterNode parameter, String label) {
Set result = new HashSet();
for(ConstraintNode constraint : fConstraints){
if(constraint.mentions(parameter, label)){
result.add(constraint);
}
}
return result;
}
public Set getMentioningConstraints(ChoiceNode choice){
Set result = new HashSet();
for(ConstraintNode constraint : fConstraints){
if(constraint.mentions(choice)){
result.add(constraint);
}
}
return result;
}
public List getMentioningTestCases(ChoiceNode choice){
List result = new ArrayList();
for(TestCaseNode testCase : fTestCases){
if(testCase.getTestData().contains(choice)){
result.add(testCase);
}
}
return result;
}
public boolean isParameterMentioned(MethodParameterNode parameter){
for(ConstraintNode constraint : fConstraints){
if(constraint.mentions(parameter)){
return true;
}
}
if(fTestCases.isEmpty()){
return false;
}
return true;
}
public void removeAllTestCases() {
fTestCases.clear();
registerChange();
}
public void replaceTestCases(List testCases){
fTestCases.clear();
fTestCases.addAll(testCases);
registerChange();
}
public void replaceConstraints(List constraints){
fConstraints.clear();
fConstraints.addAll(constraints);
registerChange();
}
public void removeAllConstraints() {
fConstraints.clear();
registerChange();
}
@Override
public int getMaxChildIndex(AbstractNode potentialChild) {
if (potentialChild instanceof AbstractParameterNode) {
return getParameters().size();
}
if (potentialChild instanceof ConstraintNode) {
return getConstraintNodes().size();
}
if (potentialChild instanceof TestCaseNode) {
return getTestCases().size();
}
return super.getMaxChildIndex(potentialChild);
}
@Override
public Object accept(IModelVisitor visitor) throws Exception{
return visitor.visit(this);
}
@Override
public boolean isMatch(AbstractNode node){
if(node instanceof MethodNode == false){
return false;
}
MethodNode comparedMethod = (MethodNode)node;
int testCasesCount = getTestCases().size();
int constraintsCount = getConstraintNodes().size();
if(testCasesCount != comparedMethod.getTestCases().size() ||
constraintsCount != comparedMethod.getConstraintNodes().size()){
return false;
}
for(int i = 0; i < testCasesCount; i++){
if(getTestCases().get(i).isMatch(comparedMethod.getTestCases().get(i)) == false){
return false;
}
}
for(int i = 0; i < constraintsCount; i++){
if(getConstraintNodes().get(i).isMatch(comparedMethod.getConstraintNodes().get(i)) == false){
return false;
}
}
return super.isMatch(node);
}
@Override
public List getMethods(AbstractParameterNode parameter) {
return Arrays.asList(new MethodNode[]{this});
}
public List getLinkers(GlobalParameterNode globalParameter){
List result = new ArrayList();
for(MethodParameterNode localParameter : getMethodParameters()){
if(localParameter.isLinked() && localParameter.getLink() == globalParameter){
result.add(localParameter);
}
}
return result;
}
public final List getMethodParameters() {
List result = new ArrayList<>();
for(AbstractParameterNode parameter : getParameters()){
result.add((MethodParameterNode)parameter);
}
return result;
}
public List getMethodParameters(boolean expected) {
List result = new ArrayList<>();
for(MethodParameterNode parameter : getMethodParameters()){
if(parameter.isExpected()){
result.add(parameter);
}
}
return result;
}
public MethodParameterNode getMethodParameter(ChoiceNode choice){
AbstractParameterNode parameter = choice.getParameter();
for(MethodParameterNode methodParameter : getMethodParameters()){
if(methodParameter == parameter || methodParameter.getLink() == parameter){
return methodParameter;
}
}
return null;
}
public List getAvailableGlobalParameters() {
if(getClassNode() != null){
return getClassNode().getAvailableGlobalParameters();
}
return new ArrayList<>();
}
public void removeConstraintsWithParameter(MethodParameterNode methodParameter) {
ArrayList constraintsToDelete = new ArrayList();
for(ConstraintNode constraint : fConstraints){
if (constraint.mentionsParameter(methodParameter)) {
constraintsToDelete.add(constraint);
}
}
for (ConstraintNode constraint : constraintsToDelete) {
fConstraints.remove(constraint);
}
registerChange();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy